From the data sheet, we can know that the CD pin is actually the I/O that controls the switching of commands and data. In the program, we use RS instead of
The following figure shows the 8080 parallel port connection diagram
The following figure shows the pinout of N76E003 (because I don’t have the money to make a board, I used thermal transfer to make and test it, so the GPIO allocation is unreasonable)
D0-D7 are assigned values using the following function. The specific operation is to right shift once each time and perform & operation with 1 at the lowest bit.
void SET_DATA(uchar val)
{
P04=val&1;
P03=(val>>1)&1;
P01=(val>>2)&1;
P00=(val>>3)&1;
P10=(val>>4)&1;
P11=(val>>5)&1;
P12=(val>>6)&1;
P13=(val>>7)&1;
}
Secondly, all GPIO are set to strong push-pull mode
P00_PushPull_Mode;
P01_PushPull_Mode;
P02_PushPull_Mode;
P03_PushPull_Mode;
P04_PushPull_Mode;
P05_PushPull_Mode;
P06_PushPull_Mode;
P07_PushPull_Mode;
P10_PushPull_Mode;
P11_PushPull_Mode;
P12_PushPull_Mode;
P13_PushPull_Mode;
P14_PushPull_Mode;
P15_PushPull_Mode;
P16_PushPull_Mode;
P17_PushPull_Mode;
P30_PushPull_Mode;
The following figure shows the UC1705 chip initialization process
Here we take soft reset as an example, the command is 0XE2
void LCD_Write_Byte(uchar Cmd,uchar Dat)//Operate according to the data sheet
{
LCD_CS=0; //Chip select
LCD_RS=Cmd; //Choose whether to send command or data
LCD_RD=0;
LCD_WR=0;
SET_DATA(Dat);
LCD_RD=1;
Timer0_Delay1ms(2);
LCD_CS=1;
LCD_RD=0;
}
LCD_Write_Byte(0,0xE2); //Send soft reset command
1
The rest of the commands are not described here. The following is the complete code
#include "N76E003.h"
#include "Common.h"
#include "Delay.h"
#include "SFR_Macro.h"
#include "Function_define.h"
#define uint unsigned int
#define uchar unsigned char
sbit LCD_CS=P0^5; //Chip select port
sbit LCD_RST=P0^6; // reset
sbit LCD_RS=P0^7; //Data/command switch C/D
sbit LCD_WR=P3^0; //write data
sbit LCD_RD=P1^7; //read data
const uchar Number8X16[]={
/*-- Text: 0 --*/
/*-- Songti 12; The corresponding dot matrix under this font is: width x height = 8x16 --*/
0x00,0xE0,0x10,0x08,0x08,0x10,0xE0,0x00,0x00,0x0F,0x10,0x20,0x20,0x10,0x0F,0x00,
/*-- Text: 1 --*/
/*-- Songti 12; The corresponding dot matrix under this font is: width x height = 8x16 --*/
0x00,0x10,0x10,0xF8,0x00,0x00,0x00,0x00,0x00,0x20,0x20,0x3F,0x20,0x20,0x00,0x00,
/*-- Text: 2 --*/
/*-- Songti 12; The corresponding dot matrix under this font is: width x height = 8x16 --*/
0x00,0x70,0x08,0x08,0x08,0x88,0x70,0x00,0x00,0x30,0x28,0x24,0x22,0x21,0x30,0x00,
/*-- Text: 3 --*/
/*-- Songti 12; The corresponding dot matrix under this font is: width x height = 8x16 --*/
0x00,0x30,0x08,0x88,0x88,0x48,0x30,0x00,0x00,0x18,0x20,0x20,0x20,0x11,0x0E,0x00,
/*-- Characters: 4 --*/
/*-- Songti 12; The corresponding dot matrix under this font is: width x height = 8x16 --*/
0x00,0x00,0xC0,0x20,0x10,0xF8,0x00,0x00,0x00,0x07,0x04,0x24,0x24,0x3F,0x24,0x00,
/*-- Characters: 5 --*/
/*-- Songti 12; The corresponding dot matrix under this font is: width x height = 8x16 --*/
0x00,0xF8,0x08,0x88,0x88,0x08,0x08,0x00,0x00,0x19,0x21,0x20,0x20,0x11,0x0E,0x00,
/*-- Characters: 6 --*/
/*-- Songti 12; The corresponding dot matrix under this font is: width x height = 8x16 --*/
0x00,0xE0,0x10,0x88,0x88,0x18,0x00,0x00,0x00,0x0F,0x11,0x20,0x20,0x11,0x0E,0x00,
/*-- Text: 7 --*/
/*-- Songti 12; The corresponding dot matrix under this font is: width x height = 8x16 --*/
0x00,0x38,0x08,0x08,0xC8,0x38,0x08,0x00,0x00,0x00,0x00,0x3F,0x00,0x00,0x00,0x00,
/*-- Characters: 8 --*/
/*-- Songti 12; The corresponding dot matrix under this font is: width x height = 8x16 --*/
0x00,0x70,0x88,0x08,0x08,0x88,0x70,0x00,0x00,0x1C,0x22,0x21,0x21,0x22,0x1C,0x00,
/*-- Characters: 9 --*/
/*-- Songti 12; The corresponding dot matrix under this font is: width x height = 8x16 --*/
0x00,0xE0,0x10,0x08,0x08,0x10,0xE0,0x00,0x00,0x00,0x31,0x22,0x22,0x11,0x0F,0x00,
};
void SET_DATA(uchar val)
{
P04=val&1;
P03=(val>>1)&1;
P01=(val>>2)&1;
P00=(val>>3)&1;
P10=(val>>4)&1;
P11=(val>>5)&1;
P12=(val>>6)&1;
P13=(val>>7)&1;
}
/******************************************************************************************
**Function name: void LCD_Write_Byte(u8 Cmd,u8 Dat)
**Function: Write command to LCD12864
**Description: None
**Parameter: Cmd =0 Command Cmd =1 Data
**************************************************************************************/
void LCD_Write_Byte(uchar Cmd,uchar Dat) //LCD write function
{
LCD_CS=0;
LCD_RS=Cmd;
LCD_RD=0;
LCD_WR=0;
SET_DATA(Dat);
LCD_RD=1;
Timer0_Delay1ms(2);
LCD_CS=1;
LCD_RD=0;
}
///**********************************************************************************
//**Function name: void LCD_Write_Byte(u8 Cmd,u8 Dat)
//**Function: Write command to LCD12864
//**Description: None
//**Parameter: Cmd =0 Command Cmd=1 Data
//**************************************************************************************/
void LCD_Write_Byte80(uchar Cmd,uchar Dat) //LCD write function
{
// u16 para;
LCD_CS=0;
LCD_RS=Cmd;
LCD_WR=0;
SET_DATA(Dat);
Timer0_Delay1ms(2);
LCD_RD=1;
Timer0_Delay1ms(2);
LCD_CS=1;
}
///**********************************************************************************
//**Function name: void LCD_Reset()
//**Function: Reset LCD12864
//**Description: None
//**************************************************************************************/
void LCD_Reset() //LCD hardware reset
{
LCD_RST=0;
Timer0_Delay1ms(50);
LCD_RST=0;
Timer0_Delay1ms(50);
LCD_RST=1;
Timer0_Delay1ms(50);
}
void LCD_Coor(uchar x, uchar y) //LCD coordinate control
{
LCD_Write_Byte(0,0xb0+y); //Set page address
LCD_Write_Byte(0,(x>>4)+0x10); //Set the high 4 bits of the column address
LCD_Write_Byte(0,x&0x0f); //Set the lower 4 bits of the column address
}
void LCD_Clr() //Clear screen function
{
uchar i,j;
for(i=0;i<9;i++)
{
LCD_Coor(0,i);
for(j=0;j<132;j++)
{
LCD_Write_Byte(1,0x00);
}
}
}
void LCD_UC1705_Init()
{
LCD_Reset();
Timer0_Delay1ms(20);
LCD_Write_Byte(0,0xE2); Software reset
Timer0_Delay1ms(20);
//--The 8th command in the table, 0xA0 segment (left and right) direction selects the normal direction (0xA1 is the reverse direction)--//
LCD_Write_Byte(0,0xA0); //ADC select segment direction
Timer0_Delay1ms(20);
//--The 15th command in the table, 0xC8 is the reverse direction for normal (up and down) direction selection, 0xC0 is the normal direction--//
LCD_Write_Byte(0,0xC8); //Common direction
Timer0_Delay1ms(20);
//--The 9th command in the table, 0xA6 sets the font to black and the background to white---//
//--0xA7 sets the font to white and the background to black---//
LCD_Write_Byte(0,0xA2); // //reverse display
Timer0_Delay1ms(20);
//--Table No. 10 command, 0xA4 pixels are displayed normally, 0xA5 pixels are fully open--//
LCD_Write_Byte(0,0xA4); // //normal display
Timer0_Delay1ms(20);
//--The 11th command in the table, 0xA3 bias is 1/7, 0xA2 bias is 1/9--//
LCD_Write_Byte(0,0xA2); //bias set 1/9
Timer0_Delay1ms(20);
//--The 19th command in the table, this is a double-byte command, 0xF800 selects the boost to 4X;--//
//--0xF801, select boost to 5X, the effect is almost the same--//
LCD_Write_Byte(0,0xF8); //Boost ratio set
Timer0_Delay1ms(20);
LCD_Write_Byte(0,0x01); //x4
Timer0_Delay1ms(20);
//--The 18th command in the table, this is a double-byte command, the high byte is 0X81, the low byte can be--//
//--Select from 0x00 to 0x3F. Used to set the background light contrast. ---/
LCD_Write_Byte(0,0x81); //V0 a set
Timer0_Delay1ms(20);
LCD_Write_Byte(0,0x23); //
Timer0_Delay1ms(20);
//--The 17th command in the table, select to adjust the resistivity--//
LCD_Write_Byte(0,0x25); //Ra/Rb set
Timer0_Delay1ms(20);
LCD_Write_Byte(0,0x2F); //--The 16th command in the table, power settings. --//
Timer0_Delay1ms(20);
LCD_Write_Byte(0,0x40); //Starting line starts from the first line
Timer0_Delay1ms(20);
// LCD_Write_Byte(0,0xB0);
Timer0_Delay1ms(20);
// LCD_Write_Byte(0,0x10);
Timer0_Delay1ms(20);
// LCD_Write_Byte(0,0x00);
Timer0_Delay1ms(20);
LCD_Write_Byte(0,0xAF); //Display on
Timer0_Delay1ms(20);
LCD_Clr();
}
///********************************************************************************/
Function Name: Disp_Dat(uchar Row,uchar Col,uchar Number,uchar fs)
Function: Output the displayed data to the specified position on the screen
Input parameter: low row address
Input parameter: Col column address
Input parameter: Number Display data
Input parameter: fs display mode (0, reverse display, otherwise normal display)
Return value: None
///********************************************************************************/
void Disp_Dat(uchar Row,uchar Col,uchar Number,uchar fs)
{
uchar L_H,L_L; //column
uchar Page; //page
//Calculate the page address
Page=0xb0+Row;
L_H=0x10+(Col>>4);
L_L=(Col&0x0f);
LCD_Write_Byte(0,Page);
LCD_Write_Byte(0,0x1f&L_H); //Column address, write high and low bytes twice, starting from column 0
LCD_Write_Byte(0,L_L);
if(fs==0)
{
LCD_Write_Byte(1,~Number);
}
else
{
LCD_Write_Byte(1,Number);
}
}
///********************************************************************************/
Function Name: Disp_Nub8X16(uchar Row,uchar Col,uchar Number,uchar fs)
Function: Output the 25x48 dot matrix value to the specified position on the screen for display
Input parameter: low row address
Input parameter: Col column address
Input parameter: Number Display value
Input parameter: fs display mode (0, reverse display, otherwise normal display)
Return value: None
///********************************************************************************/
void Disp_Nub8X16(uchar Row,uchar Col,uchar Number,uchar fs)
{
uchar i,j;
uchar Temp;
const uchar *STR_p;
STR_p=&Number8X16[Number*16];
for(i=0;i<2;i++)
{
for(j=Col;j
//Temp=pgm_read_byte(STR_p);//
Temp=*STR_p;
Disp_Dat(Row,j,Temp,fs);
STR_p++;
}
Row++;
}
}
void main(void)
{
uint x=0;
P00_Quasi_Mode;
Previous article:PID Control of Motor Speed Based on Single Chip Microcomputer
Next article:N76E003 download pins Dat, Clk, Rst for normal use
Recommended ReadingLatest update time:2024-11-16 13:03
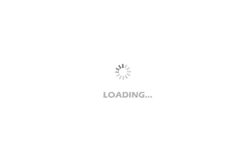
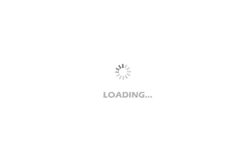
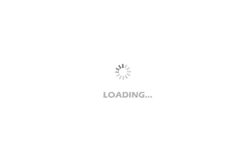
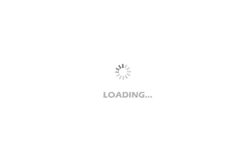
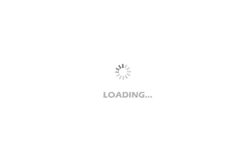
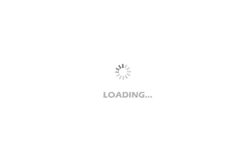
- Popular Resources
- Popular amplifiers
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
Modern Product Design Guide
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Where do you usually find pdf materials on IC?
- Here comes the useful information! Future layout and solutions of the Internet of Things
- [Mil MYS-8MMX] Mil MYS-8MMQ6-8E2D-180-C unpacking report 1——intuitive experience
- Design and application of dielectric filters
- Embedded media processor that handles tasks of both MCU and DSP
- [Modelsim FAQ] ModelSim has no timing simulation option
- msp430 MCU ADC12 sampling voltage to 1602 display program
- MOS tube working status problem after turning on
- Please help analyze this bistable circuit
- Is there a schematic library and package library for basic components candance?