2. Flowing light design and buzzer sound
1. Simple delay program:
Use the while() loop to achieve a delay effect
#include void main() { sbit D1=p1^0; //declare a variable, set p0 equal to D1 int=a; while(1) //This is an infinite loop { a=500; // indicates time delay D1=0; // indicates low level, i.e. the light is off while (a--); // means a is decremented to 0 and the following program is executed a=500; D1=1; while(a--); } } 2. Subroutine call: Design a delay function first, then call it directly! #include #define uint unsigned char //This is a macro definition. In the future, you can directly input uahr using char without declaring it again! #defne uint unsigned int sbit D1=P1^0; void delay(); //The main function calls the sub-function, declare the function first, a function without parameters! void main() { D1=0; delay(); D1=1; delay(); } void delay()//This is a delay function to control x, y can control the delay time! No parameters! { uint x,y; for(x=100;x>0;x--) for(y=200;y>0;y--) } Sub-function with parameters: more convenient to adjust. #include #define uint unsigned char //This is a macro definition. In the future, you can directly input uahr using char without declaring it again! #defne uint unsigned int sbit D1=P1^0; void delay(uint z);//The main function calls the sub-function, declare the function first, the function with parameters! void main() { D1=0; delay(600); D1=1; delay(600); } void delay(uint z) //This is a delay function to control x, y can control the delay time! With parameters! { uint x,y; for(x=100;x>0;x--) for(y=z;y>0;y--) } 3. Flowing water lamp design A running light has 8 pieces, corresponding to 8 bits, and the binary representation is: 1111 1110 ~1111 1101~1111 1011~~~ This forms a running light (0 is bright) At this time, you need to call the left shift function: to implement the above pipeline #include #include "instrins.h//Left shift function header file #define uchar unsigned char //This is a macro definition. In the future, you can directly input uchahr using char without declaring it again! #defne uint unsigned int uchar=temp; //define an eight-bit variable void delay(uint z);//The main function calls the sub-function, declare the function first, the function with parameters! void main() { temp=0xfe; //0xfe means: 1111 1110 defines P1 port P1=temp; while(1) { temp=_crol_(temp,1);//Call left shift function delay(1000); P1=temp; } } void delay(uint z) //This is a delay function to control x, y can control the delay time! With parameters! { uint x,y; for(x=z;x>0;x--) for(y=z;y>0;y--) } 4. Buzzer design PNP transistor: When b is low level, the ec circuit forms a voltage drop, and the buzzer sounds. When b is high level, there is no voltage drop and the buzzer does not sound. (b is controlled by the microcontroller) Method: Just control the buzzer io port directly! #include #include "instrins.h//Left shift function header file #define uchar unsigned char //This is a macro definition. In the future, you can directly input uchahr using char without declaring it again! #defne uint unsigned int uchar=temp; //define an eight-bit variable sbit feng = P2^3; //The third bit of P2 port is the buzzer port void main() { temp=0xfe; //0xfe means: 1111 1110 defines P1 port P1=temp; feng=0;//At this time, the buzzer keeps ringing and the running light starts moving! while(1) { temp=_crol_(temp,1);//Call left shift function delay(60); P1=temp; } } void delay(uint z) //This is a delay function to control x, y can control the delay time! With parameters! { uint x,y; for(x=100;x>0;x--) for(y=z;y>0;y--) } 加油!!!你一定行!
Previous article:51 MCU interrupt mainly about timing -- timing -- calculating the initial value of the timer -- introduction
Next article:51 MCU Learning——8.3--Serial Communication
Recommended ReadingLatest update time:2024-11-15 16:03
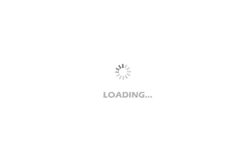
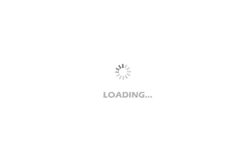
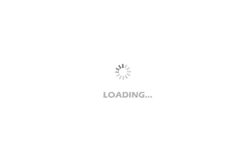
- Popular Resources
- Popular amplifiers
-
Siemens PLC Programming Technology and Application Cases (Edited by Liu Zhenquan, Wang Hanzhi, Yang Kun, etc.)
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
Implementing a Deep Learning Framework with Python (Zhang Juefei, Chen Zhen)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Huawei's Strategic Department Director Gai Gang: The cumulative installed base of open source Euler operating system exceeds 10 million sets
- Download from the Internet--ARM Getting Started Notes
- Learn ARM development(22)
- Learn ARM development(21)
- Learn ARM development(20)
- Learn ARM development(19)
- Learn ARM development(14)
- Learn ARM development(15)
- Analysis of the application of several common contact parts in high-voltage connectors of new energy vehicles
- Wiring harness durability test and contact voltage drop test method
- Technical Title
- CS83702 single lithium battery 3.7V power supply built-in boost 18W mono class D amplifier IC
- 【Renovation of old things】 Graffiti desk lamp——lugl4313820
- Share the silkscreen search method of TI device models
- Some thoughts on the development of embedded technology
- Uncover the secrets of Shenzhou XII’s journey home!
- Maximize power density with buck-boost charging and USB Type-C PD technology
- MYZR-IMX6-CB336 core board
- [Telink's new generation low power consumption, high performance, multi-protocol wireless kit B91 review] Unboxing + lighting
- Seek guidance from the experts and find routines.