#include #include "./delay/delay.h" #define LCD_WRITE_DATA 1 #define LCD_WRITE_COM 0 #define LCDPORT P0 #define SUCC 0 #define ERR 1 sbit RS = P2^4; sbit RW = P2^5; sbit E = P2^6; sbit SCL = P1^0; sbit SDA = P1^1; bit ack = 0; void iic_start() { SDA = 1; SCL = 1; delay_us(1); SDA = 0; delay_us(1); SCL = 0; } void iic_stop() { SDA = 0; SCL = 1; delay_us(1); SDA = 1; delay_us(1); SCL = 0; } bit iic_send_byte(unsigned char byte) { unsigned char i; SDA = 1; for(i = 0;i < 8;i++) { SDA = byte & 0x80; SCL = 1; delay_us(1); SCL = 0; byte <<= 1; } SCL = 1; SDA = 1; delay_us(1); if(0 == SDA) { ack = 1; } else { ack = 0; } SCL = 0; return ack; } void iic_noack() { SDA = 1; SCL = 1; delay_us(1); SCL = 0; } void iic_ack() { SDA = 0; SCL = 1; delay_us(1); SCL = 0; } unsigned char iic_rcv_byte() { unsigned char i; unsigned char temp = 0; unsigned char a; SDA = 1; for(i = 0;i < 8;i++) { SCL = 0; delay_us(1); SCL = 1; if(SDA == 1) { a = 0x01; } else { a = 0x0; } temp |= (a<<(7-i)); delay_us(1); } SCL = 0; return temp; } unsigned char AT24C02_send_str(unsigned char devaddr,unsigned char romaddr,unsigned char *s,unsigned char num) { unsigned char i; iic_start(); iic_send_byte(devaddr); if(0 == ack) return ERR; iic_send_byte(romaddr); if(0 == ack) return ERR; for(i = 0;i < num;i++) { iic_send_byte(*s); if(0 == ack) return ERR; s++; } iic_stop(); return SUCC; } unsigned char AT24C02_rcv_byte(unsigned char devaddr,unsigned char romaddr,unsigned char *s,unsigned char num) { unsigned char i; iic_start(); iic_send_byte(devaddr); if(0 == ack) return ERR; iic_send_byte(romaddr); if(0 == ack) return ERR; iic_start(); iic_send_byte(devaddr + 1); for(i = 0;i < num - 1;i++) { *s = iic_rcv_byte(); iic_ack(); s++; } *s = iic_rcv_byte(); iic_noack(); iic_stop(); return SUCC; } void lcd1602_write_data(unsigned char byte,unsigned char flag) { if(flag) { RS = 1; } else { RS = 0; } RW = 0; E = 1; LCDPORT = byte; delay_us(5); E = 0; } void lcd_init() { delay_ms(15); lcd1602_write_data(0x38,LCD_WRITE_COM); delay_ms(5); lcd1602_write_data(0x38,LCD_WRITE_COM); delay_ms(5); lcd1602_write_data(0x38,LCD_WRITE_COM); delay_ms(5); lcd1602_write_data(0x38,LCD_WRITE_COM); delay_ms(5); lcd1602_write_data(0x08,LCD_WRITE_COM); delay_ms(5); lcd1602_write_data(0x01,LCD_WRITE_COM); delay_ms(5); lcd1602_write_data(0x06,LCD_WRITE_COM); delay_ms(5); lcd1602_write_data(0x0c,LCD_WRITE_COM); delay_ms(5); } void lcd_write_str(unsigned char x,unsigned char y,unsigned char *p) { if((x > 15) || (y > 1)) { return; } if(0 == y) { lcd1602_write_data(0x80+x,LCD_WRITE_COM); } else { lcd1602_write_data(0x80+0x40+x,LCD_WRITE_COM); } while(*p != '') { lcd1602_write_data(*p,LCD_WRITE_DATA); p++; } } unsigned char AD_read() { unsigned char temp; iic_start(); iic_send_byte(0x90); if(0 == ack) return ERR; iic_send_byte(0x40); iic_start(); iic_send_byte(0x90+1); if(0 == ack) return ERR; } temp = iic_rcv_byte(); iic_noack(); iic_stop(); return temp; void lcd_dis_char(unsigned char x, unsigned char y, unsigned char byte) { if((x > 15) || (y > 1)) { return ; } if(0 == y) { lcd1602_write_data(0x80 + x,LCD_WRITE_COM); } else { lcd1602_write_data(0x80 + 0x40 + x,LCD_WRITE_COM); } lcd1602_write_data(byte,LCD_WRITE_DATA); } unsigned char DA_write(unsigned char num) { iic_start(); iic_send_byte(0x90); if(0 == ack) return ERR; iic_send_byte(0x40); if(0 == ack) return ERR; iic_send_byte(num); if(0 == ack) return ERR; iic_stop(); return SUCC; } void main() { unsigned char num; unsigned char test; unsigned char v; lcd_init(); while(1) { test = AD_read(); v = test*100 / 51; lcd_dis_char(0,0,(test/100)+0x30); lcd_dis_char(1,0,(test%100/10)+0x30); lcd_dis_char(2,0,(test%10)+0x30); lcd_dis_char(0,1,(v/100)+0x30); lcd_dis_char(1,1,'.'); lcd_dis_char(2,1,(v%100)/10+0x30); lcd_dis_char(3,1,(v%10)+0x30); DA_write(num); num++; delay_ms(2); } }
Previous article:High impedance state and I/O port working state
Next article:80C51 serial port structure and principle (2-program)
Recommended ReadingLatest update time:2024-11-17 05:00
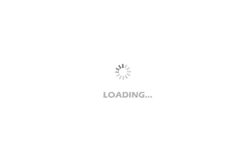
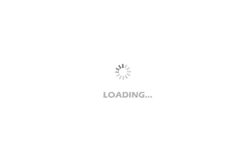
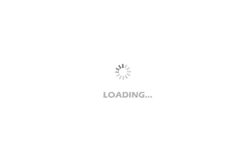
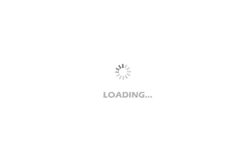
- Popular Resources
- Popular amplifiers
-
MATLAB and FPGA implementation of wireless communication
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Operational Amplifier Practical Reference Handbook (Edited by Liu Changsheng, Zhao Mingying, Liu Xu, etc.)
-
A Complete Illustrated Guide to Operational Amplifier Applications (Written by Wang Zhenhong)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- Rambus Launches Industry's First HBM 4 Controller IP: What Are the Technical Details Behind It?
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- msp430G2231 ADC10 configuration
- [Domestic RISC-V Linux Board Fang·Starlight VisionFive Trial Report] Tornado Data Writing and Reading
- stm32 register boundary address problem
- 【Gravity:AS7341 Review】+First Look at AS7341
- With 6 development boards per person and over 10,000 yuan in prize money, ON Semiconductor and Avnet's IoT Innovation Design Competition is waiting for you to join!
- DM6437 peripheral detailed tutorial
- 【EasyARM-RT1052 Review】 + cJSON transplantation and use
- Construction of the Crazy Shell AI open source drone development environment
- Causes and solutions for poor wetting in PCBA processing
- T6963C LCD often flickers