.long 0x00000700 ; BANKCON4
.long 0x00000700 ; BANKCON5
.long 0x00018005 ; BANKCON6
.long 0x00018005 ; BANKCON7
.long 0x008C07A3 ; REFRESH
.long 0x000000B1 ; BANKSIZE
.long 0x00000030 ; MRSRB6
.long 0x00000030 ; MRSRB7
Next, start writing the makefile. Before writing the makefile, you first need to understand the compilation process of gcc:
The program compilation process can be divided into four steps:
Preprocessing: mainly.c and.h files generate a file (processing a header file, macro definition, precompilation instructions, etc.)
Compilation: Generate .s files from the previous step (including syntax analysis, optimization, etc.)
Assembly: .s file generates .o file (translates assembly language code into target machine instructions)
Linking: .o and .a are connected into an executable file, the default is a.out (connecting related target files to each other)
The following code is used to illustrate each process:
/*hello.c*/
#include int main () { printf("hellon") return 0; } First, preprocess: gcc –E hello.c The -E parameter tells gcc to do only preprocessing without compiling, assembling, or linking. When executed, a lot of information will be printed in the terminal. This is the file generated after preprocessing. Then execute gcc –S hello.c The -S parameter tells gcc to only compile without assembling or linking. After executing this command, you will get a .s assembly file with the following content: .file "hello.c" .section .rodata .LC0: .string "hello" .text .globl main .type main, @function main: .LFB0: .cfi_startproc pushl %ebp .cfi_def_cfa_offset 8 .cfi_offset 5, -8 movl %esp, %ebp .cfi_def_cfa_register5 andl $-16, %esp subl $16, %esp movl $.LC0, (%esp) call puts movl $0, %eax leave .cfi_restore 5 .cfi_def_cfa 4, 4 ret .cfi_endproc .LFE0: .size main, .-main .ident "GCC: (Ubuntu 4.8.2-19ubuntu1)4.8.2" .section .note.GNU-stack,"",@progbits Then run: gcc -c hello.c Get hello.o, the content of hello.o is machine code and cannot be viewed in plain text Finally, run the link. The link requires the ld command, which is used directly here: gcc hello.c All steps can be completed. This is the default generation of .out files. Next some makefiles Makefile: For execution in SRAM, it can be: CFLAGS := -Wall -Wstrict-prototypes -O2 -nostdlib led.bin :init.S led.c arm-linux-gcc $(CFLAGS) -c -o init.oinit.S arm-linux-gcc $(CFLAGS) -c -o led.o led.c arm-linux-ld -Ttext 0 init.o led.o -o led_elf arm-linux-objcopy -O binary -S led_elf led.bin #arm-linux-objdump -D -m arm led_elf > led.dis clean: rm -rf *.o *elf *bin *.dis For CFAGS, that is, the gcc option is explained as follows: -Wall generates all warning messages. -Wstrict-prototypes Warn if a function definition or declaration does not specify argument types. There are five -O settings: -O0, -O1, -O2, -O3, and -Os O0: This level (the letter "O" followed by a zero) turns off all optimization options and is the default level when no -O level is set in CFLAGS or CXXFLAGS. This will not optimize the code, which is usually not what we want. -O1: This is the most basic optimization level. The compiler will try to generate faster and smaller code without spending too much compilation time. These optimizations are very basic, but generally these tasks will definitely be completed successfully. -O2: An improvement over -O1. This is the recommended optimization level unless you have special requirements. -O2 enables more flags than -O1. With -O2, the compiler will try to improve code performance without increasing the size and compilation time. -O3: This is the highest and most dangerous optimization level. Using this option will increase the time it takes to compile code, and should not be enabled globally on a system using gcc 4.x. The behavior of gcc has changed significantly since 3.x. In 3.x, -O3 generated code that was only slightly faster than -O2, and it may not be faster in gcc 4.x. Compiling all packages with -O3 will produce larger, more memory-intensive binaries, and greatly increase the chance of compilation failures or unpredictable program behavior (including errors). Doing so will not be worth the effort, and too much is as bad as too little. Using -O3 with gcc 4.x is not recommended. -Os: This level is used to optimize for code size. It enables the code generation options of -O2 that do not increase disk space usage. This is useful on machines with extremely limited disk space or small CPU caches. However, it can cause some problems, so most ebuilds in the software tree filter out this level of optimization. Using -Os is not recommended. (All parameters can be viewed in man gcc) The next two lines are compiled into .o files respectively For this sentence: arm-linux-ld -Ttext 0 init.o led.o -o led_elf, the function of -Ttext is to specify the starting address of the program segment. Here, only the running address of the code segment is specified, and the following data segment will not be stored in the back. That is, the program jumps to 0 (the starting address of sran) to run. Another point is to link init.0 and led.o to generate an executable file of ELF. ELF (excutable and linking format) is a binary file in an executable and linkable format. It can be used to represent relocatablefile, executablefile or sharedobject file, all three of which are target files (objectfile). The so-called "executable" means that it can be loaded into the memory for direct operation by the CPU; "linkable" means that multiple ELF format target files can be linked together to form an executable file. arm-linux-objcopy is used to copy the contents of an object file to another file. This option can convert the format. Here, the elf format file is converted to a .bin binary file. For arm-linux-objcopy -Obinary -S led_elf led.bin -O is followed by the output query type, and -S means not to copy the relocation information and symbol information from the input file to the target file. As for the led.c file, the program for turning on the light by pressing the button above is still used. When everything is ready, you can compile and then download the final generated led.bin/ file to the development board. If successful, the LED will light up when a button is pressed and will go out when the button is released.
Previous article:6. 2440 bare metal development LCD operation
Next article:3. Setting the clock and timer of the bare metal system of s3c2440
Recommended ReadingLatest update time:2024-11-16 13:31
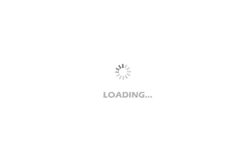
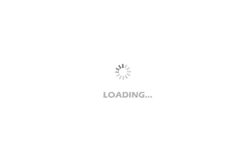
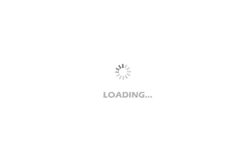
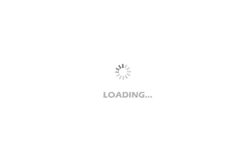
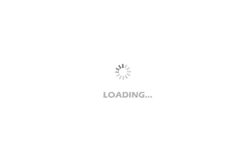
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- A comprehensive look at the entire family of MSP430 microcontrollers
- CircuitPython upgraded to 5.2.0
- TI shared power bank design experience sharing, if you like it, please read it
- Installation and sound source localization algorithm of a diamond microphone array
- How to start the design of adjustable voltage regulated power supply application
- Has anyone used the AT32F403 microcontroller? Is there any example program or something?
- A detail of MSP430 interrupt
- The upper limit for each mobile phone is US$2.5. How much 5G patent fees can Huawei collect?
- Schematic diagram of one-input and eight-output terminal block
- IEEE Top Programming Language Rankings 2021