Editor: LCD backlight control is actually an I/O port driver, which is exactly the same as LED control, or even simpler, because it usually controls several LEDs at the same time. This is a standard entry-level driver. I won't say much about this, just follow the manual. The I/O port here is GPG4.
1 LCD backlight control principle
In the mini2440/micro2440 development board, the LCD backlight is controlled by the LCD_PWR pin of the CPU. As can be seen from the schematic diagram, it corresponds to GPG4. When the LCD_PWR output is a high level "1", the backlight will be turned on; when the output is a low level "0", the backlight will be turned off (Note: here it only turns the backlight on and off, and does not adjust the backlight brightness).
2 Adding backlight driver to the kernel
Now, we need to add a simple backlight driver so that the backlight can be easily turned on and off by software. Our goal is to turn off the backlight by sending an even number such as "0" to the backlight device in the command terminal, and turn on the backlight by sending an odd number such as "1". This makes it much more convenient to use without the need for a dedicated application to control it, as described in the user manual (2.5.10 Controlling LCD backlight):
Tip: LCD backlight device file: /dev/backlight
Enter in the command line: echo 0 > /dev/backlight to turn off the LCD backlight.
Enter in the command line: echo 1 > /dev/backlight to turn on the LCD backlight.
To achieve this, we add a mini2440_backlight.c file in the linux-2.6.32.2/drivers/video directory
with the following content:
;The following header files may not be required for every one of them, but the redundant ones will not affect the content of the driver
#include
#include
#include
linux/slab.h>
#include
#include
#include
linux/delay.h>
#include <
linux/clk.h>
#include
linux/gpio.h
> #include <
asm/io.h>
#include
#include
#include
#include
#include
#include
#undef DEBUG
//#define DEBUG
#ifdef DEBUG
#define DPRINTK(x...) {printk(__FUNCTION__"(%d): ",__LINE__);printk(##x);}
#else
#define DPRINTK(x...) (void)(0)
#endif
;Define the name of the backlight driver as backligh, which will appear in /dev/backlight
#define DEVICE_NAME "backlight"
;Define the backlight variable bl_state to record the on/off state of the backlight
static unsigned int bl_state;
;Set the function of the backlight switch, mainly to flip the backlight variable bl_state
static inline void set_bl(int state)
{
bl_state = !!state; //Flip the bl_state variable
s3c2410_gpio_setpin(S3C2410_GPG(4), bl_state); //Write the result to the register GPG4 used by the backlight
}
;Get the backlight state
static inline unsigned int get_bl(void)
{
return bl_state;
}
;Read parameters from the application and pass them to the kernel
static ssize_t dev_write(struct file *file, const char *buffer, size_t count, loff_t * ppos)
{
unsigned char ch;
int ret;
if (count == 0) {
return count;
}
;Use copy_from_user function to read parameters from the user layer/application layer
ret = copy_from_user(&ch, buffer, sizeof ch) ? -EFAULT : 0;
if (ret) {
return ret;
}
ch &= 0x01; //Judge whether it is an odd or even number
set_bl(ch); //Set backlight status
return count;
}
;Pass kernel parameters to the read function of user layer/application layer
static ssize_t dev_read(struct file *filp, char *buffer, size_t count, loff_t *ppos)
{
int ret;
unsigned char str[] = {'0', '1' };
if (count == 0) {
return 0;
}
;Use copy_to_user function to pass kernel parameters to user layer/application layer
ret = copy_to_user(buffer, str + get_bl(), sizeof(unsigned char) ) ? -EFAULT : 0;
if (ret) {
return ret;
}
return sizeof(unsigned char);
}
;Device operation set
static struct file_operations dev_fops = {
owner: THIS_MODULE,
read:dev_read,
write: dev_write,
};
static struct miscdevice misc = {
.minor = MISC_DYNAMIC_MINOR,
.name = DEVICE_NAME,
.fops = &dev_fops,
};
;Device initialization, effective when the kernel starts
static int __init dev_init(void)
{
int ret;
ret = misc_register(&misc);
printk (DEVICE_NAME"tinitializedn");
;Initialize the backlight port GPG4 as output
s3c2410_gpio_cfgpin(S3C2410_GPG(4), S3C2410_GPIO_OUTPUT);
;Turn on the backlight when starting the kernel
set_bl(1);
return ret;
}
static void __exit dev_exit(void)
{
misc_deregister(&misc);
}
module_init(dev_init); //Register backlight driver module
module_exit(dev_exit); //Uninstall backlight driver module
MODULE_LICENSE("GPL");
MODULE_AUTHOR("FriendlyARM Inc.");
MODULE_AUTHOR("FriendlyARM Inc.");
Then add the backlight configuration option to the kernel configuration menu, open linux-2.6.32.2/drivers/video/Kconfig, and add it in the following location:
config FB_S3C2410_DEBUG
bool "S3C2410 lcd debug messages"
depends on FB_S3C2410
help
Turn on debugging messages. Note that you can set/unset at run time
through sysfs
#Add the backlight driver configuration of MINI2440 here
config BACKLIGHT_MINI2440
tristate "Backlight support for mini2440 from FriendlyARM"
depends on MACH_MINI2440 && FB_S3C2410
help
backlight driver for MINI2440 from FriendlyARM
config FB_SM501
tristate "Silicon Motion SM501 framebuffer support"
depends on FB && MFD_SM501
select FB_CFB_FILLRECT
select FB_CFB_COPYAREA
select FB_CFB_IMAGEBLIT
Then open linux-2.6.32.2/drivers/video/Makefile and add the driver target file according to the configuration definition, as shown in the figure:
# the test framebuffer is last
obj-$(CONFIG_FB_VIRTUAL) += vfb.o
#video output switch sysfs driver
obj-$(CONFIG_VIDEO_OUTPUT_CONTROL) += output.o
obj-$(CONFIG_BACKLIGHT_MINI2440) += mini2440_backlight.o
In this way, we have transplanted the mini2440 backlight driver into the kernel. In the kernel source code root directory, execute:
make menuconfig, and select the following submenus in turn:
Device Drivers --->
Graphics support --->
< *> Support for frame buffer devices --->
<*>backlight support for mini2440 from Friendlyarm
Here, press the space bar to select the mini2440 configuration item we just added, then exit and save the kernel configuration menu. Execute in the command line: make zImage
will generate arch/arm/boot/zImage, use supervivi's "k" function to burn it into the development board, and you can see the penguin image as shown in the figure at startup, which means that we have turned on the backlight, but there are still some problems with the LCD driver. In the next section, we will introduce in detail how to port the LCD driver.
The added device file node is shown in the figure:
Previous article:Linux-2.6.32 transplanted on mini2440 development board - RTC transplanted
Next article:Linux-2.6.32 transplanted on mini2440 development board - W35 LCD driver transplanted
Recommended ReadingLatest update time:2024-11-16 10:33
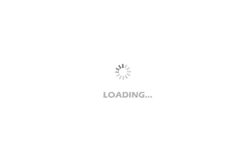
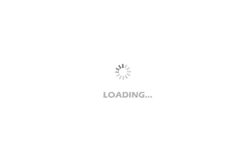
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Work and classes are suspended, but learning continues! Rohm R Classroom is officially launched online
- How to design the switching power supply input filter circuit?
- 【BLE 5.3 wireless MCU CH582】6. PWM breathing light
- In these years in the forum
- Supercapacitor constant power charging and voltage regulation
- Design of low power consumption, wireless type wireless sensor network
- EEWORLD University ---- Big Data Machine Learning (Yuan Chun)
- BLE GATT service characteristic values and types
- Boss, what's your home WIFI password? ——Qorvo~Wi-Fi 6
- [RVB2601 Creative Application Development] 2 Realize long press and short press