Well, I have to admit that I am very used to using Freescale's XS128 microcontroller. But when I first started using atmega8, what I disliked the most was that atmega8 could not operate the IO pins, and had to use some cumbersome bit operations. I don't want to do that. I want to operate like Freescale...
then......
Put the following header file I wrote into include/avr in the winavr directory, and include this header file at the end of the io.h header file.
Hehe, everything has become so intimate and familiar. . . . .
/***************************************************************
* Library Description: ATMEGE8
* Version: v1.0
* Edited by: Pang Hui, Wuhu Lianda Freescale Studio
* Modified on: August 4, 2011
*
* Description: None
*
* new version update:
*
************************************************************
*Note: None
***************************************************************/
#ifndef _BIT_
#define _BIT_
//Define an 8-byte bit segment. Bit 0 to 7 are the names of each bit segment. 1 represents one bit. PBIT is the name of the entire bit segment.
typedef struct
{
unsigned bit0 : 1 ;
unsigned bit1 : 1 ;
unsigned bit2 : 1 ;
unsigned bit3 : 1 ;
unsigned bit4 : 1 ;
unsigned bit5 : 1 ;
unsigned bit6 : 1 ;
unsigned bit7 : 1 ;
}PBIT;
//Force conversion
#define PORTABIT (*(volatile PBIT *)0x3B)
#define DDRABIT (*(volatile PBIT *)0x3A)
#define PINABIT (*(volatile PBIT *)0x39)
#define PORTBBIT (*(volatile PBIT *)0x38)
#define DDRBBIT (*(volatile PBIT *)0x37)
#define PINBBIT (*(volatile PBIT *)0x36)
#define PORTCBIT (*(volatile PBIT *)0x35)
#define DDRCBIT (*(volatile PBIT *)0x34)
#define PINCBIT (*(volatile PBIT *)0x33)
#define PORTDBIT (*(volatile PBIT *)0x32)
#define DDRDBIT (*(volatile PBIT *)0x31)
#define PINDBIT (*(volatile PBIT *)0x30)
//Continue packaging
#define PORTA_PA0 PORTABIT.bit0
#define PORTA_PA1 PORTABIT.bit1
#define PORTA_PA2 PORTABIT.bit2
#define PORTA_PA3 PORTABIT.bit3
#define PORTA_PA4 PORTABIT.bit4
#define PORTA_PA5 PORTABIT.bit5
#define PORTA_PA6 PORTABIT.bit6
#define PORTA_PA7 PORTABIT.bit7
#define PORTB_PB0 PORTBBIT.bit0
#define PORTB_PB1 PORTBBIT.bit1
#define PORTB_PB2 PORTBBIT.bit2
#define PORTB_PB3 PORTBBIT.bit3
#define PORTB_PB4 PORTBBIT.bit4
#define PORTB_PB5 PORTBBIT.bit5
#define PORTB_PB6 PORTBBIT.bit6
#define PORTB_PB7 PORTBBIT.bit7
#define PORTC_PC0 PORTCBIT.bit0
#define PORTC_PC1 PORTCBIT.bit1
#define PORTC_PC2 PORTCBIT.bit2
#define PORTC_PC3 PORTCBIT.bit3
#define PORTC_PC4 PORTCBIT.bit4
#define PORTC_PC5 PORTCBIT.bit5
#define PORTC_PC6 PORTCBIT.bit6
#define PORTC_PC7 PORTCBIT.bit7
#define PORTD_PD0 PORTDBIT.bit0
#define PORTD_PD1 PORTDBIT.bit1
#define PORTD_PD2 PORTDBIT.bit2
#define PORTD_PD3 PORTDBIT.bit3
#define PORTD_PD4 PORTDBIT.bit4
#define PORTD_PD5 PORTDBIT.bit5
#define PORTD_PD6 PORTDBIT.bit6
#define PORTD_PD7 PORTDBIT.bit7
//**********************
#define DDRA_DDRA0 DDRABIT.bit0
#define DDRA_DDRA1 DDRABIT.bit1
#define DDRA_DDRA2 DDRABIT.bit2
#define DDRA_DDRA3 DDRABIT.bit3
#define DDRA_DDRA4 DDRABIT.bit4
#define DDRA_DDRA5 DDRABIT.bit5
#define DDRA_DDRA6 DDRABIT.bit6
#define DDRA_DDRA7 DDRABIT.bit7
#define DDRB_DDRB0 DDRBBIT.bit0
#define DDRB_DDRB1 DDRBBIT.bit1
#define DDRB_DDRB2 DDRBBIT.bit2
#define DDRB_DDRB3 DDRBBIT.bit3
#define DDRB_DDRB4 DDRBBIT.bit4
#define DDRB_DDRB5 DDRBBIT.bit5
#define DDRB_DDRB6 DDRBBIT.bit6
#define DDRB_DDRB7 DDRBBIT.bit7
#define DDRC_DDRC0 DDRCBIT.bit0
#define DDRC_DDRC1 DDRCBIT.bit1
#define DDRC_DDRC2 DDRCBIT.bit2
#define DDRC_DDRC3 DDRCBIT.bit3
#define DDRC_DDRC4 DDRCBIT.bit4
#define DDRC_DDRC5 DDRCBIT.bit5
#define DDRC_DDRC6 DDRCBIT.bit6
#define DDRC_DDRC7 DDRCBIT.bit7
#define DDRD_DDRD0 DDRDBIT.bit0
#define DDRD_DDRD1 DDRDBIT.bit1
#define DDRD_DDRD2 DDRDBIT.bit2
#define DDRD_DDRD3 DDRDBIT.bit3
#define DDRD_DDRD4 DDRDBIT.bit4
#define DDRD_DDRD5 DDRDBIT.bit5
#define DDRD_DDRD6 DDRDBIT.bit6
#define DDRD_DDRD7 DDRDBIT.bit7
//********************
#define PINA_PA0 PINABIT.bit0
#define PINA_PA1 PINABIT.bit1
#define PINA_PA2 PINABIT.bit2
#define PINA_PA3 PINABIT.bit3
#define PINA_PA4 PINABIT.bit4
#define PINA_PA5 PINABIT.bit5
#define PINA_PA6 PINABIT.bit6
#define PINA_PA7 PINABIT.bit7
#define PINB_PB0 PINBBIT.bit0
#define PINB_PB1 PINBBIT.bit1
#define PINB_PB2 PINBBIT.bit2
#define PINB_PB3 PINBBIT.bit3
#define PINB_PB4 PINBBIT.bit4
#define PINB_PB5 PINBBIT.bit5
#define PINB_PB6 PINBBIT.bit6
#define PINB_PB7 PINBBIT.bit7
#define PINC_PC0 PINCBIT.bit0
#define PINC_PC1 PINCBIT.bit1
#define PINC_PC2 PINCBIT.bit2
#define PINC_PC3 PINCBIT.bit3
#define PINC_PC4 PINCBIT.bit4
#define PINC_PC5 PINCBIT.bit5
#define PINC_PC6 PINCBIT.bit6
#define PINC_PC7 PINCBIT.bit7
#define PIND_PD0 PINDBIT.bit0
#define PIND_PD1 PINDBIT.bit1
#define PIND_PD2 PINDBIT.bit2
#define PIND_PD3 PINDBIT.bit3
#define PIND_PD4 PINDBIT.bit4
#define PIND_PD5 PINDBIT.bit5
#define PIND_PD6 PINDBIT.bit6
#define PIND_PD7 PINDBIT.bit7
#endif
Previous article:Use of atmega8 Flash
Next article:atmega8 example: T1 timer CTC mode square wave output
Recommended ReadingLatest update time:2024-11-15 10:51
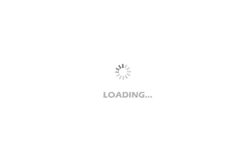
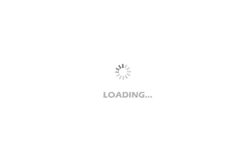
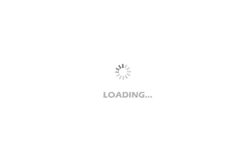
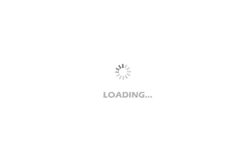
- Popular Resources
- Popular amplifiers
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- ASML predicts that its revenue in 2030 will exceed 457 billion yuan! Gross profit margin 56-60%
- Detailed explanation of intelligent car body perception system
- How to solve the problem that the servo drive is not enabled
- Why does the servo drive not power on?
- What point should I connect to when the servo is turned on?
- How to turn on the internal enable of Panasonic servo drive?
- What is the rigidity setting of Panasonic servo drive?
- How to change the inertia ratio of Panasonic servo drive
- What is the inertia ratio of the servo motor?
- Is it better for the motor to have a large or small moment of inertia?
- Design and implementation of asynchronous FIFO based on Verilog HDL.pdf
- The common-mode gain, differential-mode gain, and power supply rejection ratio of the op amp are shown in the figure.
- 01. Environment construction and driving 0.96OLED
- 【RT-Thread software package application work】Small alarm clock
- Qorvo 100 MHz Envelope Tracking Solution for 5G
- 【Beetle ESP32-C3】3. Adjust LED brightness with knob (potentiometer) (Arduino)
- China's first batch of autonomous driving unmanned commercial licenses issued! Do you dare to sit in an unmanned car? ?
- GDB debugging and DSP
- The IO pin of MSP430 is set as input, but it receives the output signal of the Hall sensor, and the chip-side signal does not switch.
- [Serial] [Starlight Lightning STM32F407 Development Board] Chapter 10 Serial Communication Experiment