This program displays the data read from the color recognition module on LCD1602, and generates corresponding PWM waves to operate the three-color lights to display different colors, so as to achieve color replication and display. The wiring methods of each module are introduced in detail in the source code. At the same time, related project files and materials can be downloaded at the bottom of the article.
Regarding the problem of garbled characters when pasting and copying: If garbled characters appear in the comments when the program is copied to the Keil compiler, you can first create a .c file, that is, do not edit it in Keil, then open the .c file with Notepad, copy the source code into it, and then add the file in Keil.
/******************************************************************************************
Based on TCS3200/230 color reproduction display
* Purpose: Used for color copy display, the TCS3200 module converts the acquired data into the corresponding PWM wave to display it on the three-color light and also displays it on the LCD1602
*Wiring method
P0 is LCD1602 data port
P2.5 connects to LCD1602 RS
P2.6 connects to LCD1602 RW
P2.7 connects to LCD1602 EN
*Three-color light connection method
P1.5 to the red terminal
P1.6 connects to the green terminal
P1.7 connects to the blue terminal
*TCS3200 connection method
Module S2-----MCU P1.1
Module S3-----MCU P1.0
Module OUT----MCU P3.5 (Counter 1 input)
Module VCC----MCU VCC
Module GND----MCU GND
******************************************************************************************/
#include #include #include #include #define uchar unsigned char #define uint unsigned int #define DataPort P0 //LCD1602 data port sbit LCM_RS=P2^5; //LCD1602 control port sbit LCM_RW=P2^6; //LCD1602 control port sbit LCM_EN=P2^7; //LCD1602 control port sbit Red=P1^5; sbit Green=P1^6; sbit Blue=P1^7; /**Pin definition**/ sbit s2=P1^1; //TCS3200 S2 sbit s3=P1^0; //TCS3200 S3 //TCS3200 S0 module internal default pull-up //TCS3200 S1 module internal default pull-up //TCS3200 OE module internal grounding sbit test_pin=P1^2; //Use an oscilloscope to observe this pin to know the timer interrupt frequency //Definition of variables and constants uchar ge,shi,bai ; uchar rp=3,gp=3,bp=6; //Define the scale factor, which can be modified in specific environment uchar count; //Color flag (0: red 1: green 2: blue) uint RC=0,GC=0,BC=0; // Display array uchar disp_R[3]; //red uchar disp_G[3]; //green uchar disp_B[3]; //blue //********Define function********************************* void delay(unsigned int k); void InitLcd(); void WriteDataLCM(uchar dataW); void WriteCommandLCM(uchar CMD,uchar Attribc); void DisplayOneChar(uchar X,uchar Y,uchar DData); //************LCD1602 initialization************************ void InitLcd() { WriteCommandLCM(0x38,1); WriteCommandLCM(0x08,1); WriteCommandLCM(0x01,1); WriteCommandLCM(0x06,1); WriteCommandLCM(0x0c,1); } //**********Detect busy signal**************************** void WaitForEnable(void) { DataPort=0xff; LCM_RS=0;LCM_RW=1;_nop_(); LCM_EN=1;_nop_();_nop_(); while(DataPort&0x80); LCM_EN=0; } //**********Write command to LCD*********************** void WriteCommandLCM(uchar CMD,uchar Attribc) { if(Attribc)WaitForEnable(); LCM_RS=0;LCM_RW=0;_nop_(); DataPort=CMD;_nop_(); LCM_EN=1;_nop_();_nop_();LCM_EN=0; } //**********Write data to LCD************************ void WriteDataLCM(uchar dataW) { WaitForEnable(); LCM_RS=1;LCM_RW=0;_nop_(); DataPort=dataW;_nop_(); LCM_EN=1;_nop_();_nop_();LCM_EN=0; } //************Write a character data to the specified target************ void DisplayOneChar(uchar X,uchar Y,uchar DData) { Y&=1; X&=15; if(Y)X|=0x40; X|=0x80; WriteCommandLCM(X,0); WriteDataLCM(DData); } //**********Delay function*************** void delay(unsigned int k) { unsigned int i,j; for(i=0;i for(j=0;j<121;j++) {;} } } /*********************************************** * Function name: t0_init() * Function: Timer 0 initialization * Input parameters: None * Export parameters: None /************************************************/ void t0_init() { TMOD=0x51; //T1 counts T0 timing working mode 1 TH1=0x00; //Counting initial value TL1=0x00; TH0=0xE0; TL0=0x00; //11.0592M crystal oscillator 10ms EA=1; //Enable interrupt ET0=1; TR0=1; //Start TR1=1; } //************************************************ // Convert the value into ASCII code of tens, hundreds, and thousands //************************************************ void conversion(uint temp_data) { bai=temp_data/100+0x30; temp_data=temp_data%100; //Remainder operation shi=temp_data/10+0x30; ge=temp_data%10+0x30; // remainder operation } /*********************************************** * Function name: main() /************************************************/ void main() { delay(10); InitLcd(); //lcd initialization s2=0; //Initial setting of S2 pin s3=0; //Initial setting of S3 pin t0_init(); //Timer counting initialization? while(1) { uint count = 0; /**********************The dumbest way Display various colors through the main function loop ******************/ while(1) { if(count<=RC) { Red = 0; } else { Red = 1; } if(count<=BC) { Blue = 0; } else { Blue = 1; } if(count<=GC) { Green = 0; } else { Green = 1; } count++; if(count>=256) { count = 0; } } } } /*********************************************** * Function name: c10ms_out() * Function: Timer interrupt 0 service routine Modify the color flag disp_tc (0: red 1: green 2: blue) Set S0 S1 S2 Select filter Count pulses and read color values * Input parameters: None * Export parameters: None /************************************************/ void c10ms_out() interrupt 1 { uint temp; test_pin=!test_pin; //Test the timer interrupt frequency pin, which can be observed with an oscilloscope TR0=0; //Turn off timing TR1=0; //Close the count // count+1 detects green first, then blue, then red, and repeats the detection if(count==0) { count++; s2=1;s3=1; //Select filter as green temp=(8< GC=temp; conversion(temp); disp_R[2]=ge; disp_R[1]=shi; disp_R[0]=bai; } else if(count==1) { count++; s2=1;s3=0; //Select filter as blue temp=(8< BC=temp; conversion(temp); disp_G[2]=ge; disp_G[1]=shi; disp_G[0]=bai; } else if(count==2) { count=0; s2=0;s3=0; //Select filter as red temp=(8< RC=temp; conversion(temp); disp_B[2]=ge; disp_B[1]=shi; disp_B[0]=bai; } DisplayOneChar(0, 0, 'T'); DisplayOneChar(1, 0, 'C'); DisplayOneChar(2, 0, 'S'); DisplayOneChar(3, 0, '2'); DisplayOneChar(4, 0, '3'); DisplayOneChar(5, 0, '0'); DisplayOneChar(10, 0, 'R'); DisplayOneChar(11, 0, '['); DisplayOneChar(12, 0, disp_R[0]); DisplayOneChar(13, 0, disp_R[1]); DisplayOneChar(14, 0, disp_R[2]); DisplayOneChar(15, 0, ']'); DisplayOneChar(0, 1, 'G'); DisplayOneChar(1, 1, '['); DisplayOneChar(2, 1, disp_G[0]); DisplayOneChar(3, 1, disp_G[1]); DisplayOneChar(4, 1, disp_G[2]); DisplayOneChar(5, 1, ']'); DisplayOneChar(10, 1, 'B'); DisplayOneChar(11, 1, '['); DisplayOneChar(12, 1, disp_B[0]); DisplayOneChar(13, 1, disp_B[1]); DisplayOneChar(14, 1, disp_B[2]); DisplayOneChar(15, 1, ']'); //Re-initialize the timer counter TH0=0x01; TL0=0x00; //11.0592M crystal oscillator, 10ms TL1=0x00; //Counter clear TH1=0x00; //Counter clear TR0=1; //Turn on the timer TR1=1; //Open the counter }
Previous article:The smallest fragment of a single chip microcomputer [with pictures] [very detailed]
Next article:51 single chip microcomputer course design: temperature and humidity alarm based on DHT11
Recommended ReadingLatest update time:2024-11-15 15:25
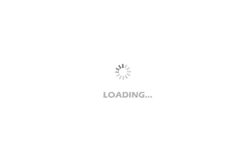
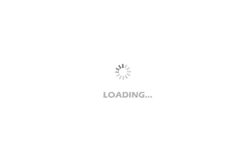
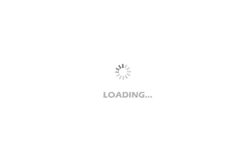
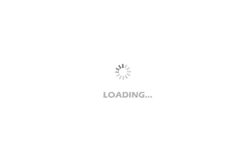
- Popular Resources
- Popular amplifiers
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Fundamentals and Applications of Single Chip Microcomputers (Edited by Zhang Liguang and Chen Zhongxiao)
-
Single chip microcomputer control technology (Li Shuping, Wang Yan, Zhu Yu, Zhang Xiaoyun)
- Learn ARM development(19)
- Learn ARM development(14)
- Learn ARM development(15)
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Learn ARM development(19)
- Learn ARM development(14)
- Learn ARM development(15)
- Analysis of the application of several common contact parts in high-voltage connectors of new energy vehicles
- Wiring harness durability test and contact voltage drop test method
- From probes to power supplies, Tektronix is leading the way in comprehensive innovation in power electronics testing
- From probes to power supplies, Tektronix is leading the way in comprehensive innovation in power electronics testing
- Sn-doped CuO nanostructure-based ethanol gas sensor for real-time drunk driving detection in vehicles
- Design considerations for automotive battery wiring harness
- Do you know all the various motors commonly used in automotive electronics?
- ALLEGRO's latest solution for hybrid and electric vehicles - starter generator
- Analysis of the schematic diagram of infrared diode sensing circuit
- [Raspberry Pi Pico Review] MicroPython Development Environment Setup
- Vivado Getting Started and Improved (High Runner-up)
- MicroPython Hands-on (38) - Controlling the Touch Screen
- Use serial port tools and serial port assistants to monitor the communication process between the mainboard MCU and the Bluetooth module--
- [SAMR21 New Gameplay] 20. Analog Input and Analog Output
- The meaning of some letters in transistor models
- [RVB2601 development board trial experience] GPIO output test
- Experience in debugging Ethernet half-duplex of GD32F450