1. ECT module introduction
The enhanced capture timer module (ECT) of the XEP100 microcontroller is a timer module developed by adding some functions on the basis of the standard timer module (TIM). ECT is particularly suitable for the application of automobile ABS, ESP and other systems. The ECT module includes a 16-bit programmable counter. ECT has multiple functions, the most important of which are: input capture (IC), output comparison (OC), pulse accumulation (PAI) and modulus down counting (MDC). This article mainly studies the most commonly used input capture and output comparison functions. The figure below is the functional block diagram of the ECT module.
The ECT module has 8 input capture and output compare channels. When a channel is set to input, it has input capture function. The input capture function can measure some characteristics of the input pulse signal. It can measure the period, duty cycle and frequency of the pulse.
The input capture channel consists of 4 buffered channels IC0~IC3 and 4 unbuffered channels IC4~IC7. The functions of these two parts are to capture the moment when external events occur, but the buffered channel has a holding register TCnH in addition to the IC/OC register TCn. In addition, a delay counter is set at the entrance to improve the anti-interference ability. The unbuffered channel has no holding register and no delay counter.
The following figure is a block diagram of the input capture function. When the ECT module is enabled, a clock will keep counting. This clock is called TCNT, which is the "16-bit master clock" in the figure. TCNT starts counting from $0000 and increases by 1 for each ECT clock cycle. After adding to $FFFF, TCNT is reset to $0000. The pulse signal is input from the microcontroller pin, and the signal is input to the ECT module after passing through the edge detector and delay counter. When a valid edge (rising edge or falling edge) is detected, the ECT module captures the value of TCNT into the input capture register TCn, and the value of TCNT captured by the previous pulse edge will be saved in TCnH. The programmer can calculate the time interval between the two edges, that is, the pulse period, by reading the values captured by two adjacent edges and calculating the difference between the two values.
When the channel is set to output, the channel has an output comparison function. The output comparison function is generally used for precise timing and can also be used to generate PWM signals. When used, first write a value to the output comparison register TCn. When the value of TCNT is equal to the value of TCn, an output comparison event can be generated, and the flag bit CnF is set to 1. If the interrupt function is enabled, an interrupt can be triggered. The corresponding program can be executed in the interrupt program.
2. ECT output comparison test
The resources in this article include the test code for the output comparison and input capture functions of the ECT module. In this experiment, we will study the output comparison function of ECT. The following code is the initialization of the ECT module.
void initialize_ect(void){
ECT_TSCR1_TFFCA = 1; // Timer flag clear quickly
ECT_TSCR1_TEN = 1; // Timer enable bit. 1 = Allow the timer to work normally; 0 = Disable the main timer (including the counter)
ECT_TIOS = 0xff; //Specify all channels as output comparison mode
ECT_TCTL1 = 0x00; // The last four channels are set as timers disconnected from the output pins
ECT_TCTL2 = 0x00; // The first four channels are set as timers disconnected from the output pins
ECT_DLYCT = 0x00; // Delay control function disabled
ECT_ICOVW = 0x00; // The corresponding register is allowed to be overwritten; NOVWx = 1, the corresponding register is not allowed to be overwritten
ECT_ICSYS = 0x00; // Disable IC and PAC holding registers
ECT_TIE = 0x00; // Disable all channel timing interrupts
ECT_TSCR2 = 0x07; // Pre-scaling coefficient pr2-pr0: 111,, clock cycle is 4us,
ECT_TFLG1 = 0xff; // Clear each IC/OC interrupt flag
ECT_TFLG2 = 0xff; // Clear the free timer interrupt flag
}
Detailed comments have been added to the code. You can analyze the code based on the comments and chip manual. The main function of this function is to enable the ECT module, set all channels to output comparison function, and disconnect all channels from the IO pins. Set the period of the ECT counter TCNT to 4us. Disable all channel interrupts.
The main function of the program is shown below.
void main(void) {
DisableInterrupts;
INIT_PLL();
INIT_LED();
initialize_ect();
EnableInterrupts;
for(;;)
{
ECT_TFLG1_C0F = 1; // Clear the flag
ECT_TC0 = ECT_TCNT + 31250; //Set the output comparison time to 0.125s
while(ECT_TFLG1_C0F == 0); //Wait until an output comparison event occurs
ECT_TFLG1_C0F = 1; // Clear the flag
ECT_TC0 = ECT_TCNT + 31250; //Set the output comparison time to 0.125s
while(ECT_TFLG1_C0F == 0); //Wait until an output comparison event occurs
ECT_TFLG1_C0F = 1; // Clear the flag
ECT_TC0 = ECT_TCNT + 31250; //Set the output comparison time to 0.125s
while(ECT_TFLG1_C0F == 0); //Wait until an output comparison event occurs
ECT_TFLG1_C0F = 1; // Clear the flag
ECT_TC0 = ECT_TCNT + 31250; //Set the output comparison time to 0.125s
while(ECT_TFLG1_C0F == 0); //Wait until an output comparison event occurs
LEDCPU = ~LEDCPU; //Reverse light status
}
}
In the main loop of the main function, ECT_TC0 = ECT_TCNT + 31250; is used for timing. Since the period of TCNT is 4us, the timing time is 4us*31250=0.125s. When the time is reached, the flag ECT_TFLG1_C0F is set to 1, and then the timing is restarted until the end of 4 cycles, that is, 0.5s is timed, and the LED's on and off state is changed. The final effect is equivalent to the LED flashing at a frequency of 1Hz. Download the program to the microcontroller and run it, and you can see the corresponding phenomenon.
3. ECT input capture test
Input capture is used to measure the characteristics of pulse signals. In order to generate a pulse signal, we use the PWM module of the microcontroller to generate a PWM pulse signal. The code is as follows.
void init_pwm(void)
{
PWMCTL_CON01= 1; //Connect channel 0, 1 to 16-bit PWM
PWMPOL_PPOL1= 1; //The polarity of channel 01 is high level valid
PWMPRCLK = 0x55; //The division factor of A clock and B clock is 32, and the frequency is 1MHz
PWMSCLA = 100; //SA clock frequency is 5KHz
PWMSCLB = 100; //SB clock frequency is 5KHz
PWMCLK = 0x02; //Channel 01 uses SA clock as the clock source
PWMCAE = 0x00; //Pulse mode is left-aligned mode
PWMPER01 = 500; //The period of channel 01 is 10Hz
PWMDTY01 = 250; //The duty cycle of channel 01 is 50%
PWME_PWME1 = 1; // Enable channel 01
}
This code sets the PWM signal to 10Hz and a duty cycle of 50%. For an explanation of this code, please refer to the article "Freescale 16-bit Microcontroller (VIII) - PWM Module Test".
The next step is to set up the ECT module. The code is as follows
void initialize_ect(void){
ECT_TSCR1_TFFCA = 1; // Timer flag clear quickly
ECT_TSCR1_TEN = 1; // Timer enable bit. 1 = Allow the timer to work normally; 0 = Disable the main timer (including the counter)
ECT_TIOS = 0xfe; //Specify all channels as output comparison mode
ECT_TCTL4 = 0x01; // Set channel 0 to capture rising edge mode
ECT_DLYCT = 0x00; // Delay control function disabled
ECT_ICOVW = 0x00; // The corresponding register is allowed to be overwritten; NOVWx = 1, the corresponding register is not allowed to be overwritten
ECT_ICSYS = 0x00; // Disable IC and PAC holding registers
ECT_TIE = 0x01; // Enable channel 0 interrupt
ECT_TSCR2 = 0x07; // Pre-scaling coefficient pr2-pr0: 111,, clock cycle is 4us,
ECT_TFLG1 = 0xff; // Clear each IC/OC interrupt flag
ECT_TFLG2 = 0xff; // Clear the free timer interrupt flag
}
This setting is quite different from the output comparison. Channel 0 is set as input function, and is set to capture rising edge, and the interrupt function of channel 0 is enabled. Other settings are consistent with the output comparison.
In this program, the interrupt method is used to process the input capture time, and the interrupt service function is shown below.
interrupt void capture(void)
{
if(ECT_TFLG1_C0F == 1)
ECT_TFLG1_C0F = 1;
time1 = time2;
time2 = ECT_TC0;
delaytime = time2-time1;
LEDCPU = ~LEDCPU;
}
When the rising edge of the external input pulse is captured, an interrupt is triggered. In the interrupt, the flag is first cleared, and then the count value captured by TC0 is read. Finally, the time interval between adjacent rising edges is calculated and saved in the delaytime variable.
Connect the PWM output pin of the microcontroller to the channel 0 pin of the ECT, and download the program to the microcontroller and run it. The value of delaytime can be seen in the debugging interface, as shown below.
It can be seen that the value of delaytime is 25000, which is the clock number of TCNT. The period of TCNT is 4us, so it can be calculated that the time interval between two rising edges is 4us*25000 = 0.1s, that is, the frequency of the pulse is 10Hz.
Previous article:Freescale 16-bit MCU (10) - PIT module test
Next article:Freescale 16-bit MCU (VIII) - PWM module test
Recommended ReadingLatest update time:2024-11-16 14:36
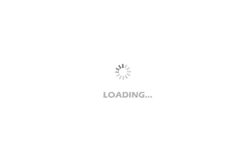
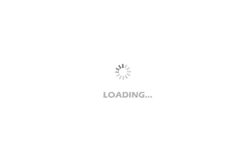
- Popular Resources
- Popular amplifiers
-
Practical Development of Automotive FlexRay Bus System (Written by Wu Baoxin, Guo Yonghong, Cao Yi, Zhao Dongyang, etc.)
-
Automotive Electronics KEA Series Microcontrollers——Based on ARM Cortex-M0+ Core
-
Automotive integrated circuits and their applications
-
ADS2008 RF Circuit Design and Simulation Examples (Edited by Xu Xingfu)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Overview of SAW and BAW Filters
- [Nucleo G071 Review] Unboxing & Introduction & Power-on & Engineering Environment Setup & Lighting & Serial Port
- RAPIO-PCIE high-speed data transmission card and linux source code, vxworks, windows and other drivers
- [RT-Thread reading notes] Part 1 Bare metal and system
- Protel 99 SE and AD have copper holes and copper grooves
- Things you have to know about FPGA
- STM32CubeIDE imports the STM32 project automatically generated by Gizwits Online (based on the MDK environment
- MSP430F5529 key interrupt scanning and pwm program
- Hello, I need help, but it's not urgent. How can I send an email using ESP8266?
- Saiyuan MCU baby bed remote control system