The development of ARM11 is very different from the STM32 development that we learned before. For STM32, the code is burned into the STM32 chip FLASH, and then the code is executed from the FLASH. Moreover, the program execution does not require external RAM, because a certain amount of RAM is integrated inside the chip.
ARM11 is different. It does not have FLASH and RAM inside, so it requires external flash devices and RAM devices, and then ARM11 operates these devices through the storage controller.
The difference can be seen intuitively from the picture. For S3C6410, because there is no internal memory, the code needs to be burned into an external FLASH device. There can be many types of FLASH devices here, but they must be flash supported by the chip. This is explained in the chip data sheet. Program execution requires memory, so an external memory device is also required to execute the program. There can also be many types of memory devices here, but it is also necessary to check the chip data sheet to see which memory device is supported.
The two development methods are also different. When developing STM32 programs, we basically use the Keil graphical interface. Compiling and linking are all handled by the compiler. We don't need to write makefiles, link scripts, or startup codes, because ST has done all of these for us. And the programs we write are basically written in C language.
But ARM11 development is different. It needs to be developed in Linux and a cross-compilation tool chain needs to be installed. At this time, there is no graphical development interface. You need to write your own makefile and link script. More importantly, you need to write your own bootloader, which is the startup code.
The instructions of the two developments are also different. ARM11 uses ARM instructions, which are 32-bit instructions, and are all 4-byte aligned. However, STM32 uses THUMB2 instructions, which include THUMB's 16-bit instructions, so the instructions are not strictly 4-byte aligned. But when writing STM32 programs, they are all written in C language, so I don't care much about these.
But developing in ARM11 is different, because when developing bootloader code, it needs to be written in assembly code, so you need to be familiar with ARM11 assembly instructions. You need to be able to write makefiles and link scripts.
Of course, there are many other differences between STM32 and ARM11, which I will not introduce here, and will explain in detail when I get to the specific places.
Let's first understand the startup process of ARM11.
This is a screenshot from the ARM boot manual. The BL in the picture refers to the bootloader.
1. After power-on, the program first executes the BL0 program, which is the program that the chip manufacturer has fixed in the chip. In this program, there will be some initialization things, the most important of which is the initialization of the memory controller, so that the chip can access the external FLASH boot device.
2. After BL0 is initialized, it will copy the first 8K data of the external boot storage device to the stepping stone according to the boot pin selection (because ARM11 supports multiple storage media boot), and then jump to the stepping stone for execution. At this time, the BL1 program we wrote is executed.
3. In the BL1 program, we will set some registers of the chip and initialize some external peripherals, such as nandflash, dram, serial port, etc. And configure the C language environment, because the subsequent programs may be written in C language. Then copy the remaining programs over 8K in the external storage boot device to DRAM, and then jump to DRAM to execute the program.
Therefore, we first need to set the BL1 program. In this program, we need to set some registers of the chip, initialize the external peripherals, and then copy the code in FLASH to DRAM, set the C language environment, that is, configure the heap, stack, and clear the BSS segment.
Let's look at the STM32 startup process. After power-on, the chip will collect the values of the boot0 and boot1 pins, and then decide whether to start from FLASH or RAM. After selecting, the startup code program will be executed, the stack will be defined (the stack size and address will be set), and then the interrupt vector table will be set, and then the clock and NVIC will be initialized. Then _main will be called. Note that _main here is not the main function we wrote, but the system's own function. This function is to initialize the resources required by C, that is, to set the stack, map the variables in the code to the memory, and then jump to the main function we wrote to start executing the program we wrote.
It can be seen that STM32 is relatively simple, because ST has already done all the work for STM32, that is, the startup assembly code added before writing the program, so what we care about is the design of the main function we wrote. But in ARM11, in addition to caring about our main function design, we also need to care about the bootloader design.
Without further ado, let’s move on to ARM11.
First of all, you must install the compilation environment. First, you need to install a virtual machine, and then install the cross tool chain on the virtual machine. That is, the arm-linux-compilation tool. There are many tutorials on the Internet, and you can achieve it by referring to the tutorials.
Then comes the writing of the program, that is, the writing of the bootloader. Here, the bootloader program is saved in the start.S file. In addition, the program is finally placed in nandflash, and the program is started from nandflash. I named this bootloader qboot.
Then write the makefile.
Codeall: start.o arm-linux-ld -Tqboot.lds -o qboot.elf $^ arm-linux-objcopy -O binary qboot.elf qboot.bin arm-linux-objdump -D -S qboot.elf > dump %.o : %.S arm-linux-gcc -g -c $^ %.o : %.c arm-linux-gcc -g -c $^ clean: rm *.o *.elf dump
In fact, it is also very simple, just convert the start.S assembly code we wrote into a bin file. Because the bin file can be burned into nandflah and executed by the chip.
Then write the link script
+ View Code
ENTRY(_start) @ The program entry is _start
SECTIONS{
. = 0x50008000; @ The starting address of the link is 0x50008000
. = ALIGN(4); @ Address 4-byte alignment
.text : @ code snippet
{
start.o(.text) @ start code segment is placed first
*(.text) @ * represents all the remaining codes
}
. = ALIGN(4); @ Code segment address, 4-byte alignment
.data : @ data section
{
*(.data) @ all data segments
}
. = ALIGN(4); @ bss segment address, 4-byte alignment
bss_start = .; @ indicates the starting address of the bss_start segment, which will be used later
.bss :
{
*(.bss) @ all bss segments
}
bss_end = .; @ indicates the end address of the bss_start segment, which will be used later
}
The link script is used to determine the address of program instructions later. In the link script, the link address of the code segment, data segment, and bss segment must be set. The starting address of the link here is 0x50008000, which is within the address range of the memory, which is reflected in the data manual.
From the figure, we can see that the address range of the first dram starts from 0x50000000 and ends at 0x5FFFFFFF. So here we set the link address to this range, because the program will be copied to the memory and executed in the memory later.
With these basic things, you can start your programming journey.
Previous article:ARM high-resolution piezoelectric ceramic drive power supply design
Next article:Arm system architecture knowledge summary
Recommended ReadingLatest update time:2024-11-16 11:50
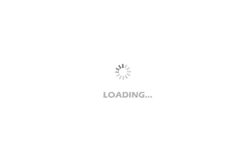
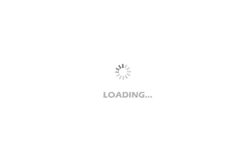
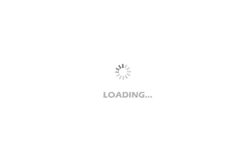
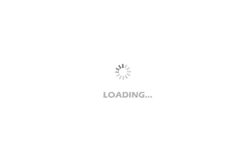
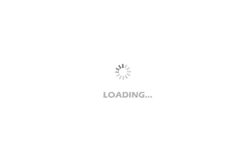
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- MCU Basics: Detailed explanation of button single click, double click, and long press
- 【McQueen Trial】Main functions corresponding to driver pins
- ti dsp (tms320VC5502) + isp1581 usb2.0 high speed data acquisition solution
- [Problem Feedback] Anlu TD4.6.6-64bit pin constraint input PIN number bank does not automatically update the problem
- Tesla's electric motor
- Design of prison security system by integrating wireless sensors with control network
- Fast charging knowledge sharing: PD protocol fast charging
- Solution of power monitoring system of Zhongjun Yunjingtai in Shangqiu, Henan
- [Voice Recognition Positioning] Material Unboxing-ESP32-S2-KALUGA-1
- EEWORLD University ---- The latest version of RTOS training - 15 days to get started with RT-Thread kernel