introduction
With the popularity of personal digital assistants (PDAs) and thin-capacity computers, touch screens are becoming more and more common in our lives as a medium for terminal and user interaction. Touch screens are divided into resistive, capacitive, surface acoustic wave and infrared scanning types, and the most commonly used is the 4-wire resistive touch screen.
This paper is based on the touch screen interface of Samsung's ARM9 core chip S3C2410, and forms the hardware foundation by connecting an external 4-wire resistive touch screen. On this basis, a touch screen surface drawing board program is developed.
1 Touch screen principle
The circuit principle of S3C2410 connected to a 4-wire resistive touch screen is shown in Figure 1.
The entire touch screen consists of a module-wise resistance ratio and a longitudinal resistance line. The four control signals nYPON, YMON, nXPON, and XMON control the on and off of four MOS tubes (S1, S2, S3, and S4). S3C2410 has eight analog input channels. Among them, channel 7 is used as the X coordinate input of the touch screen interface (AIN[7] in Figure 1), and channel 5 is used as the Y coordinate input of the touch screen interface (AIN[5] in Figure 1). The circuit is shown in Figure 2.
Before connecting to the S3C2410 touch screen interface, they all pass through a RC low-pass filter to remove the coordinate signal noise. The filtering here is very important. If the interference in the signal transmitted to the S3C2410 analog input interface is too large, it will be detrimental to the subsequent software processing. During the sampling process, the software only needs to set the special register, and the S3C2410 touch screen controller will automatically control the touch screen interface to open or close each MOS tube, and complete the X coordinate point acquisition and Y coordinate point acquisition in sequence.
2 S3C2410 touch screen controller
The S3C2410 touch screen controller has two processing modes:
①X/Y position conversion mode. The touch screen controller includes two control stages, the X coordinate conversion stage and the Y coordinate conversion stage.
②X/Y position automatic conversion mode. The touch screen controller will automatically convert the X and Y coordinates.
This article uses the X/Y position automatic conversion mode.
3 S3C2410 touch screen programming
Since the selection and optimization of parameters in the touch screen program requires multiple experiments, and adding operating system test parameters, each compilation and download takes too much time, which is not easy to conduct experiments, so we directly write bare metal touch screen programs. Samsung has opened the S3C2410 test program 2410test (which can be downloaded from the Samsung website), and provides a program example ts_auto.c for the automatic conversion mode of the touch screen interface. Based on this example, this article writes a touch screen drawing board program - drawing the traces of the touch pen on the display screen.
In view of the noise generated when sampling coordinate points, this paper adopts a noise filtering algorithm and writes a corresponding noise filtering program to filter out interfering sampling points. The processing flow of the entire touch screen drawing board program is shown in Figure 3.
3.1 Program Initialization
Initialize the touch screen controller to the automatic conversion mode. The value of the register ADCDLY needs to be selected according to the specific test. You can run the program provided in this article to see the effect of drawing lines to select specific parameters. The touch screen interrupt handler Adc_or_TsAuto determines whether the touch screen is pressed. If the touch screen is pressed, assign the global variable Flag_Touch to Touch_Down, otherwise assign it to Touch_Up.
Initialize the pulse width modulation timer (PWM TIMER), select timer 4 as the clock, define 10ms interrupt once, and provide the touch screen sampling time base, that is, 10ms touch screen sampling once. In the counter interrupt handler Timer4Intr, if Flag_Touch is assigned to Touch_Down, the global variable gTouchStartSample is set to control the touch screen sampling.
Then clear the touch screen interrupt and timer interrupt mask bits, accept the interrupt response, and the timer starts timing.
3.2 Touch screen sampling procedure
If gTouchStartSample is TRUE, the touch screen interface starts sampling the analog values of coordinates X and Y. The appropriate number of acquisitions is selected based on the experiment. This article uses 9 acquisitions, which are recorded in the ptx[TouchSample] and pty[TouchSample] arrays respectively, where TouchSample is the number of acquisitions.
In order to reduce the amount of calculation, ptx[] and pty[] are divided into three groups and the average values are stored in px[3] and py[3]. Here we take the processing of X coordinate as an example:
px[0]=(ptx[0]+ptx[1]+ptx[2])/3;
px[1]=(ptx[3]+ptx[4]+ptx[5])/3;
px[2]=(ptx[6]+ptx[7]+ptx[8])/3;
Calculate the difference between the above three sets of data:
dlXDiff0=px[0]-px[1];
dlXDiff1=px[1]-px[2];
dlXDiff2=px[2]-px[0];
Then take the absolute value of the above difference, and the result is referred to as the absolute difference:
dlXDiff0=dlXDiff0>0?dlXDiff0:-dlXDiff0;
dlXDiff1=dlXDiff1>0?dlXDiff1:-dlXDiff1;
dlXDiff2=dlXDiff2>0?dlXDiff2:-dlXDiff2;
Determine whether the color pair differences calculated above all exceed the difference threshold. If the three absolute differences all exceed the threshold, the sampling point is determined to be a wild point, the sampling point is discarded, and the program returns to wait for the next sampling. The difference threshold needs to be obtained based on experimental tests, and this article takes the value of 2.
Find the two sets of data with the smallest absolute difference, average them, and assign them to tmx:
if(dlXDiff0 if(dlXDiff2 tmx=((px[0]+px[2]>>1); } else{ tmx=((px[0]+px[1])>>1); } } else if(dlXDiff2 tmx=((px[0]+px[2])>>1); } else{ tmx=((px[1]+px[2])>>1); } The function Touch_Coordinate Conversion converts the touch screen sampling value into display coordinates. There are different conversion methods according to different hardware. The touch screen sampling coordinates and display coordinates are shown in Figures 4 and 5. Among them, TOUCH_MAX_X and TOUCH_MIN_X are the maximum and minimum values of the touch screen X coordinate sampling value; the same is true for the Y coordinate. You can run the program in this article and use the touch pen to obtain the maximum and minimum sampling values at the four corners of the touch screen. The 320×240 TFT screen is used here, so the TOUCH_X value is 320. The following is the conversion program for the X coordinate: Touch_CoordinateConversio(int*px){ TmpX=(tmx>=TOUCH_MAX_X)?(TOUCH_MAX_X):*px; TmpX-=TOUCH_MIN_X; TmpX=(TmpX)?TmpX:0; *px=(TmpX*TOUCH_X)/(TOUCH_MAX_X-TOUCH_MIN_X); } 3.3 Coordinate filtering procedure The coordinate filtering program Touch_Pen_filtering considers that there are three types of touch screen operations in the human-machine interface: *The position of the stylus on the touch screen remains unchanged; *The stylus slides continuously on the touch screen; *The stylus jumps wildly on the touch screen. Assume that three consecutive sampling times are T1, T2, and T3 (T3> T2> T1), and the sampling interval is 10ms. Since the sampling interval is much smaller than the human reaction time, in the first two operation modes, if the sampling point is valid, the sampling values at T1 and T3 are averaged. The average value compared with the sampling value at T2 is generally not greater than a certain threshold, otherwise the sampling point is judged to be a wild point. For the third mode, the sampling point data will have a large jump. The data during the jump process is unstable. Although the data is recorded, it is judged to be an invalid sampling point. Therefore, a static array x[2] needs to be defined in the program to record the two adjacent sampling data. Only when the current and subsequent data remain stable for a period of time, the sampling point is considered valid. The interval threshold FILTER_LIMIT used in the program needs to be selected through experiments. Only the filtering process of the X coordinate is given here. //*px is the sampling value at time T3, count is a static variable that records the number of consecutive valid sampling points, marking the duration of the current data stability. Once it is found to be greater than //FILTER_LIMIT, the value of count will start counting from 0 again. Int Touch_Pen_filtering(int *px){ BOOL retVal; Static int count=0; count++; //If the number of consecutive valid sampling points is greater than 2, start the filtering algorithm if(count>2){ count=2; //Average the sampling value at time T3 and the sampling value at time T1 TmpX=(x[0]+*px)/2; //Calculate the difference between the average value and the sampling value at time T2 dx=(x[1]>TmpX)?(x[1]-TmpX):(TmpX-x[1]); //If the difference is greater than the threshold, it means that the sampling value of T3 is invalid, and it is judged as a wild point and the return value is FALSE. In order to avoid excessive jumps, it is assumed that the touch pen coordinates have changed, and the sampling value at time T2 is used to replace the current sampling point. At the same time, the data in the static variable x[] remains unchanged, and count starts to record the number of consecutive valid sampling points again. if((dx>FIL TER_LIMIT)){ *px=x[1]; retVal=FLASE; count=0; } //Otherwise, the sampling point is valid and the return value is TRUE. The sampling point of T3 is recorded in x[1], and the sampling point of T2 is moved to x[0] else{ x[0]=x[1]; x[1]=*px; retVal=TRUE; } } else{ //When the number of consecutive valid sampling times is less than 2, the sampling value of T3 is recorded in x[1], and the sampling value of T2 is moved to x[0] without filtering.
Previous article:Implementation of Trusted Computing Based on ARM
Next article:Introduction to ARM processor registers
Recommended ReadingLatest update time:2024-11-16 21:35
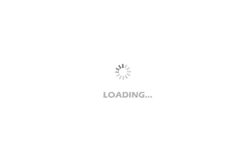
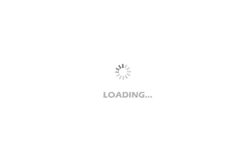
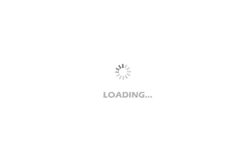
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- RF PA circuit design based on gallium nitride (GaN) devices
- [Project source code] NIOS II custom IP core writing basic framework
- What circuit is generally used to achieve the LED light gradually turning on and off function?
- Sugar Glider Part 7: Software Design of the Exercise Reward System for Sugar Glider Based on RSL10
- The magical problem encountered by KICAD
- X-NUCLEO-IKS01A3 Official Technical Data
- AT32F425-Evaluation Report-Serial Port and Waveform Display Host Computer Debugging-09
- There is a car image simulation project for paid consultation, using the Freescale solution
- Take a look at the USB Host microcontroller in gaming peripherals
- What is the component B93 on the circuit board?