Here, timer 0 is required to provide 5ms precise timing, so each timing cycle requires (5*10^-3)/(1*10^-6)=5000 times of adding 1 count, so the initial value of timer 0 is set to 65536 - 5000 = 60536 = EA84H.
Here we first implement two countdown timers working simultaneously, and the next step is to consider how to implement two countdown timers working asynchronously.
#include <STC89C5xRC.H>
void delay() //Use timer 0 to achieve 5ms precise timing
{
TMOD = 0x01;
TH0 = 0xEA;
TL0 = 0x84; //65536 - 5000 = 60036
TF0 = 0; //Set the overflow flag to 0
TR0 = 1; //Start timer 0
while(TF0 == 0);
TR0 = 0; // Pause timer 0
}
void disp_digit(int d, int r)
{
unsigned char code DIG_CODE[10] = {0x3f, 0x06, 0x5b, 0x4f, 0x66, 0x6d, 0x7d, 0x07, 0x7f, 0x6f};
//First countdown
//Display the single digit
P2 = 0; // P2 = 0 -> (P24, P23, P22) = (0, 0, 0) -> the first number from the right lights up
P0 = DIG_CODE[d % 10];
delay(); //5ms precise timing
//Display the tens digit
P2 = 1 << 2; //P2 = 0000 0100 -> (P24, P23, P22) = (0, 0, 1) -> the second digit from the right lights up
P0 = DIG_CODE[d / 10];
delay(); //5ms precise timing
//Second countdown
//Display the single digit
P2 = 6 << 2; //P2 = 0001 1000 -> (P24, P23, P22) = (1, 1, 0) -> The seventh number from the right lights up
P0 = DIG_CODE[r % 10];
delay(); //5ms precise timing
//Display the tens digit
P2 = 7 << 2; //P2 = 0001 1100 -> (P24, P23, P22) = (1, 1, 1) -> The eighth number from the right lights up
P0 = DIG_CODE[r / 10];
delay(); //5ms precise timing
}
int main()
{
int i;
int sec1, sec2;
while(1)
{
sec1 = 15;
sec2 = 15;
while(sec1 >= 0)
{
for(i = 0; i < 50; i++)
{
disp_digit(sec1, sec2); // takes about 20ms
}//20ms*50=1000ms=1s
sec1 --;
sec2 --;
}
}
return 0;
}
Previous article:MCU Learning Journey (IV) Buzzer Singing
Next article:8051 MCU (STC89C52) timer achieves 10ms accurate timing
Recommended ReadingLatest update time:2024-11-15 16:18
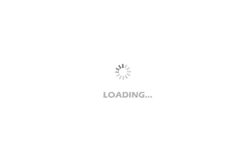
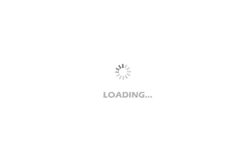
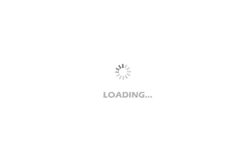
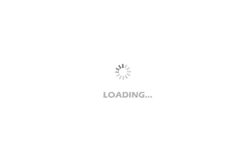
- Popular Resources
- Popular amplifiers
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
Principles and Applications of Single Chip Microcomputers 3rd Edition (Zhang Yigang)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
-
STC32G Series MCU Technical Reference Manual
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Huawei's Strategic Department Director Gai Gang: The cumulative installed base of open source Euler operating system exceeds 10 million sets
- Download from the Internet--ARM Getting Started Notes
- Learn ARM development(22)
- Learn ARM development(21)
- Learn ARM development(20)
- Learn ARM development(19)
- Learn ARM development(14)
- Learn ARM development(15)
- Analysis of the application of several common contact parts in high-voltage connectors of new energy vehicles
- Wiring harness durability test and contact voltage drop test method
- Detailed explanation of ZigBee networking principle
- [McQueen Trial] + My first program "The King asked me to patrol the mountains"
- Power supply project
- This flyback switching power supply based on TinySwitch-III
- RF wireless transmission distance
- TMS320C6678 Evaluation Module
- Data collection and display of dsp
- Power Supply Problem Summary
- The one with the highest score wins: ST Sensors Conquer the World: Driver Transplantation Competition + Bone Vibration Sensor Evaluation
- EEWORLD University Hall----TI's new generation C2000? microcontroller: full support for servo and motor drive applications