Part 1 Stack Initialization
1. Full/Empty Stack
1.1 Full stack: When the stack pointer SP always points to the last data pushed into the stack (used by ARM)
1.2 Empty stack: When the stack pointer SP always points to the next empty location where data will be placed
2. Stack Up/Down
According to the moving direction of the SP pointer when pushing into the stack: if it is from low to high, it is stacking up, and from high to low, it is stacking down (used by ARM)
3. Stack Frame
Definition: The part of the stack used by a function is called a stack frame
Two boundaries of the stack frame: fp(r11) and sp(r13)
3. Stack Function
3.1 Saving local variables
3.2 Passing parameters: When the number of parameters to be passed is greater than 4, use the stack to pass them, otherwise, use general registers to pass them
3.3 Passing Register Values
Part 2 BSS segment 1 initialization
Part 3 Jump from assembly to C
Jump mode: absolute jump
Part 4 C and assembly mixed programming
1. Where to use assembly: 1. Where efficiency is high; 2. Where direct hardware operation is required (such as coprocessor operation)
2. Calling C in assembly: directly assign the entry pointer of C to PC
(C code is in a separate C file)
3. Calling assembly from C: Declare the label as .global in the assembly file, and then call it directly as a function in C.
(Assembly code is in a separate file)
IV. Embedded Assembly in C
4.1 Format
__asm__(
Assembly statement part
: Output section
: Input part
: Destruction description section
);
//Example 1
void write_p15_c1(unsigned long value)
{
__asm__(
“mcr p15, 0, %0, c1, c0, 0n” @%0 is a placeholder parameter
:
:"r" (value) @r indicates a general register
);
}
//Example 2
usigned long read_p15_c1 (void)
{
usigned long value
__asm__(
"mrc p15, 0, %0, c1, c0, 0n" The value read from @c1 is sent to Value
:"=r" (value) @'=' indicates a write-only operand, used for output
:
:”memory”
);
return value;
}
4.2 Optimization
In programming, volatile is used to tell the compiler not to optimize the following part of the code (especially when operating on hardware)
Previous article:TQ2440 development board learning record (2) --- Setting up the stack and calling C functions
Next article:The role of the stack in the program (ARM structure)
Recommended ReadingLatest update time:2024-11-16 15:56
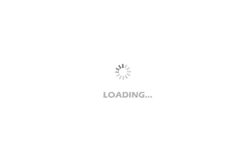
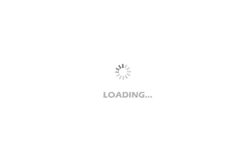
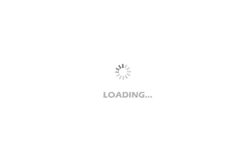
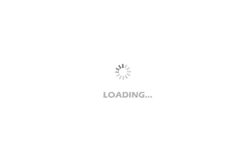
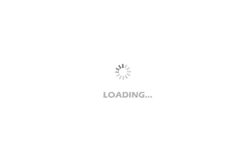
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Current Sensing Using Nanopower Op Amps
- Smart Micro MM32SPIN25PF is compatible with MM32F031CBT6
- TMS320F28335 GPIO pin general input/output port
- This is probably the best circuit design guide for analog engineers (including a compilation of articles on op amps, etc.)
- Principles and methods for selecting voltage zener diodes
- 2018 TI Cup Undergraduate Electronic Design Competition
- A new switching power supply circuit based on Schmitt trigger
- Looking for a round display similar to a smart watch
- EEWORLD University - Hejian Software Classroom - What kind of EDA tools are needed for advanced packaging?
- A small question about switching power supply