I recently encountered some problems in debugging on the STM8S microcontroller, so I shared them with you!
Question: When debugging STM8S with the IAR compiler, the program was paused and found that it could not run normally and was stuck in the serial port receive interrupt function.
Analysis: It is suspected that the serial port receiving interrupt function did not clear the flag bit, but the function does have a corresponding clear interrupt statement, which is as follows:
uint8_t tmp;
UART3_ClearFlag(UART1_FLAG_RXNE);
tmp = UART3_ReceiveData8();
Enter the UART3_ClearFlag(UART1_FLAG_RXNE) function to view the code:
void UART3_ClearFlag(UART3_Flag_TypeDef UART3_FLAG)
{
/* Check the parameters */
assert_param(IS_UART3_CLEAR_FLAG_OK(UART3_FLAG));
/*Clear the Receive Register Not Empty flag */
if (UART3_FLAG == UART3_FLAG_RXNE)
{
UART3->SR = (uint8_t)~(UART3_SR_RXNE);
}
/*Clear the LIN Break Detection flag */
else if (UART3_FLAG == UART3_FLAG_LBDF)
{
UART3->CR4 &= (uint8_t)(~UART3_CR4_LBDF);
}
/*Clear the LIN Header Detection Flag */
else if (UART3_FLAG == UART3_FLAG_LHDF)
{
UART3->CR6 &= (uint8_t)(~UART3_CR6_LHDF);
}
/*Clear the LIN Synch Field flag */
else
{
UART3->CR6 &= (uint8_t)(~UART3_CR6_LSF);
}
}
It was found that the receive interrupt flag was indeed cleared, so I went to the STM8S reference manual to look at the description of the UART3->SR register and found that in addition to the receive interrupt, the serial port communication also had an overload error interrupt.
The receive interrupt is described as follows:
RXNE: Read data register not empty
This bit is set by hardware when the data in the RDR shift register is transferred to the UART_DR register.
If the RXNEIE bit in the register is 1, an interrupt is generated. A read operation on UART_DR can clear this bit. The RXNE bit can also be
For UART2 and UART3, this bit can also be cleared by writing 0.
0: data not received;
1: Data received and can be read.
The overload error interrupt is described as follows:
RXNE: Read data register not empty
This bit is set by hardware when the data in the RDR shift register is transferred to the UART_DR register.
If the RXNEIE bit in the register is 1, an interrupt is generated. A read operation on UART_DR can clear this bit. The RXNE bit can also be
For UART2 and UART3, this bit can also be cleared by writing 0.
0: data not received;
1: Data received and can be read.
Therefore, it is found that UART3_ClearFlag (UART1_FLAG_RXNE) only clears the receive interrupt, but does not clear the overload error interrupt, because to clear the overload error interrupt, UART_SR must be read first, and then UART_DR.
The statement executed in the UART3_ClearFlag(UART1_FLAG_RXNE) function only writes UART->SR but does not read UART->SR.
UART3->SR = (uint8_t)~(UART3_SR_RXNE);
Solution: Therefore, use UART3->SR &= 0xDF instead of UART3_ClearFlag(UART1_FLAG_RXNE), which can clear both the receive interrupt and the overload error interrupt. In this way, if you pause during debugging, the program will not die in the interrupt.
uint8_t tmp;
UART3->SR &= 0xDF;
tmp = (uint8_t)UART3->DR;
Question: During STM8S debugging, sometimes some statements cannot hit breakpoints.
Analysis: I have read articles about optimization before and felt that it was related to optimization. After trying it, it turned out to be true.
Solution: Because the optimization level in the program configuration is too high, change it to no optimization, and breakpoints can be successfully set during debugging.
Previous article:STM 8 AD conversion problem
Next article:STM8S003F uses I/O port to simulate serial port (I) to send data
Recommended ReadingLatest update time:2024-11-16 07:51
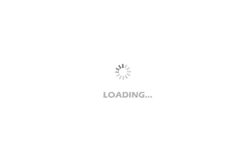
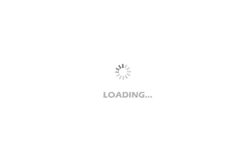
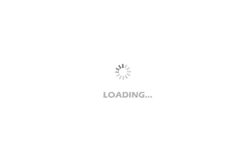
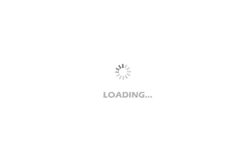
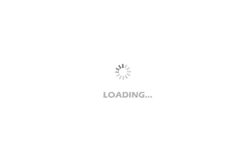
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Recruitment: Head of automotive lighting R&D, structural engineer, optical engineer, electronic engineer, 2 CAE engineers
- UV Sterilization Box TI Solution
- 【TI recommended course】#TI millimeter wave radar technology introduction#
- The new version of the Download Center is officially launched!
- Please tell me the process technology and manufacturing nodes of the current mainstream TOF sensors
- Thanks EE, I am very happy to receive the New Year gift
- [ESP32-Audio-Kit Audio Development Board] - 3: Install "esp-a...
- Understand DNS basics in one article
- Application of Dacai UART screen in environmental testing equipment
- Moto 360 Wireless Charging Base Disassembly