The main chip is 89c51, with motor, temperature sensor, infrared pyroelectric, LCD1602 as peripherals. The main function is to automatically adjust the motor speed according to the room temperature, and recognize the human body, open it when someone is on, and turn off the power when no one is on. The indoor temperature and the upper and lower limits can be adjusted by keystrokes and displayed on the 1602.
The compressed package contains the schematic, PCB board, and library. There are also codes and documents.
The schematic and PCB diagram drawn by Altium Designer are as follows:
The microcontroller source program is as follows:
#include #define uchar unsigned char //unsigned character macro definition variable range 0~255 #define uint unsigned int //Unsigned integer macro definition variable range 0~65535 #include sbit dq = P2^4; //18b20 IO port definition sbit BGVCC = P2^7; uint temperature ; // bit flag_200ms; bit flag_lj_en; //key-on enable bit flag_lj_3_en; // Press the button three times in a row to enable the added number. uchar key_time,key_value; //Used as intermediate variable for continuous addition bit key_500ms; sbit hw = P2^5; uchar miao = 30; uchar flag_en; sbit buzz=P1^3; uchar code table_num[]="0123456789abcdefg"; sbit rs=P1^2; //Register selection signal H: data register L: instruction register sbit rw=P1^1; //Register selection signal H: data register L: instruction register sbit e =P1^0; //Chip select signal falling edge trigger sbit pwm = P2^3; uchar f_pwm_l; // uchar menu_1; //Menu design variables uint t_high = 300, t_low = 200; /**************************1ms delay function********************************/ void delay_1ms(uint q) { uint i,j; for(i=0;i } /******************************************************************** * Name: delay_uint() * Function: small delay. * Input: None * Output: None ***************************************************************************/ void delay_uint(uint q) { while(q--); } /******************************************************************** * Name: write_com(uchar com) * Function: 1602 command function * Input: Input command value * Output: None ***************************************************************************/ void write_com(uchar com) { e=0; rs=0; rw=0; P0=com; delay_uint(25); e=1; delay_uint(100); e=0; } /******************************************************************** * Name: write_data(uchar dat) * Function: 1602 write data function * Input: Data to be written to 1602 * Output: None ***************************************************************************/ void write_data(uchar dat) { e=0; rs=1; rw=0; P0=dat; delay_uint(25); e=1; delay_uint(100); e=0; } /******************************************************************** * Name: write_string(uchar hang,uchar add,uchar *p) * Function: Change the value of a certain bit in the LCD. If you want the fifth character in the first line to start displaying "ab cd ef", call this function as follows write_string(1,5,"ab cd ef;") * Input: row, column, need to enter 1602 data * Output: None ***************************************************************************/ void write_string(uchar hang,uchar add,uchar *p) { if(hang==1) write_com(0x80+add); else write_com(0x80+0x40+add); while(1) { if(*p == '') break; write_data(*p); p++; } } /***********************Display specific characters on lcd1602****************************/ void write_zifu(uchar hang,uchar add,uchar date) { if(hang==1) write_com(0x80+add); else write_com(0x80+0x40+add); write_data(date); } /***********************LCD1602 displays two decimal numbers************************/ void write_sfm3_18B20(uchar hang,uchar add,uint date) { if(hang==1) write_com(0x80+add); else write_com(0x80+0x40+add); write_data(0x30+date/100%10); write_data(0x30+date/10%10); write_data('.'); write_data(0x30+date%10); } /***********************lcd1602 initialization settings****************************/ void init_1602() { write_com(0x38); // write_com(0x0c); write_com(0x06); delay_uint(1000); write_string(1,0,"temp: "); write_string(2,0,"H: L: "); write_sfm3_18B20(2,2,t_high); write_sfm3_18B20(2,10,t_low); write_zifu(1,9,0xdf); //display degree } /***************************18b20 initialization function*********************************/ void init_18b20() { bit q; EA=0; dq = 1; //Put the bus high delay_uint(1); //15us dq = 0; // give reset pulse delay_uint(80); //750us dq = 1; //Put the bus high and wait delay_uint(10); //110us q = dq; //Read 18b20 initialization signal delay_uint(20); //200us dq = 1; //Pull the bus high to release the bus EA=1 ; } /*************Write the data in 18b20***************/ void write_18b20(uchar dat) { uchar i; EA=0; for(i=0;i<8;i++) { //Write data starts from low bit dq = 0; // Pull the bus low to start the write time interval dq = dat & 0x01; //Write data to the 18b20 bus delay_uint(5); // 60us dq = 1; //Release the bus dat >>= 1; } EA=1; } /****************Read the data in 18b20****************/ uchar read_18b20() { uchar i,value; EA=0 ; for(i=0;i<8;i++) { dq = 0; //Put the bus low to start the read time interval value >>= 1; //Read data starting from low bit dq = 1; //Release the bus if(dq == 1) //Start reading and writing data value |= 0x80; delay_uint(7); //60us Reading a time gap requires at least 60us } EA=1 ; return value; //Return data } /****************The temperature value read is a decimal***************/ uint read_temp() { uint value; uchar low; //If the interrupt is too frequent when reading the temperature, the interrupt should be turned off, otherwise it will affect the timing of 18b20 EA=0; init_18b20(); //Initialize 18b20 write_18b20(0xcc); //Skip 64-bit ROM write_18b20(0x44); //Start a temperature conversion command delay_uint(50); //500us init_18b20(); //Initialize 18b20 write_18b20(0xcc); //Skip 64-bit ROM write_18b20(0xbe); //Issue a command to read the temporary register low = read_18b20(); //Read temperature low byte value = read_18b20(); //Read temperature high byte value <<= 8; //Shift the high bit of the temperature left by 8 bits value |= low; //Put the low bit of the temperature read into the low eight bits of value value *=0.625 ; //Convert to decimal temperature value EA=1; return value; //Return the read temperature with decimals } /*************Timer 0 initialization procedure****************/ void time_init() { EA = 1; // Enable general interrupt TMOD = 0X21; //Timer 0, Timer 1 working mode 1 ET0 = 1; // Enable timer 0 interrupt TR0 = 1; // Allow timer 0 to start timing ET1 = 1; // Enable timer 0 interrupt TR1 = 1; // Allow timer 0 to start timing } /************************Independent key program*****************/ uchar key_can; //key value void key() //Independent key program { static uchar key_new; key_can = 20; //key value restoration P1 |= 0xf0; if(key_500ms == 1) //Continuous addition { key_500ms = 0; key_new = 1; } if((P1 & 0xf0) != 0xf0) //button pressed { delay_1ms(1); //key debounce if(((P1 & 0xf0) != 0xf0) && (key_new == 1)) { //Confirm that the button was pressed key_new = 0; switch(P1 & 0xf0) { case 0xe0: key_can = 1; break; //Get the k1 key value case 0xd0: key_can = 2; break; //Get the K2 key value case 0xb0: key_can = 3; break; //Get the k3 key value } flag_lj_en = 1; //Continuous addition enable } } else { if(key_new == 0) { key_new = 1; flag_lj_en = 0; //Disable continuous addition enable flag_lj_3_en = 0; //Enable after 3 seconds of shutdown key_value = 0; // clear key_time = 0; key_500ms = 0; } } } /********************Button display function***************/ void key_with() { if(key_can == 1) //Set key { menu_1++; if(menu_1 >= 3) { menu_1 = 0; } if(menu_1 == 0) { write_com(0x0c); //Close the cursor } } if(menu_1 == 1) //Set high temperature alarm { if(key_can == 2) { if(flag_lj_3_en == 0) t_high ++ ; //The button is pressed but not released, and the value is automatically increased three times for(j=0;j<110;j++);
Previous article:Simple 8-key electronic keyboard program for single chip microcomputer
Next article:Based on the AD conversion program of STC15w microcontroller series
Recommended ReadingLatest update time:2024-11-25 13:30
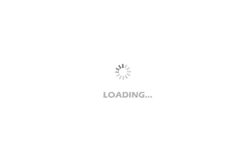
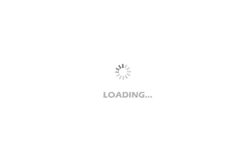
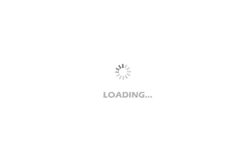
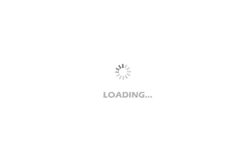
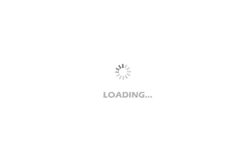
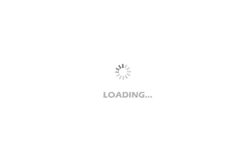
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- Automechanika Shanghai 2024 to feature a record number of concurrent events, bringing together industry wisdom and planning for future development
- Corporate Culture Sharing: How to cultivate scarce silicon IP professionals? SmartDV's journey of personal growth and teamwork
- Corporate Culture Sharing: How to cultivate scarce silicon IP professionals? SmartDV's journey of personal growth and teamwork
- NXP releases first ultra-wideband wireless battery management system solution
- NXP releases first ultra-wideband wireless battery management system solution
- Beijing Jiaotong University undergraduates explore Tektronix's advanced semiconductor open laboratory and experience the charm of cutting-edge high technology
- Beijing Jiaotong University undergraduates explore Tektronix's advanced semiconductor open laboratory and experience the charm of cutting-edge high technology
- New CEO: Dr. Torsten Derr will take over as CEO of SCHOTT AG on January 1, 2025
- How Edge AI Can Improve Everyday Experiences
- How Edge AI Can Improve Everyday Experiences
- ADS1100 16-bit analog-to-digital converter based on I2C bus
- EM78P458 chip
- The role, advantages and disadvantages of solid-state relays
- The Internet of Things helps smart agriculture, and farmers can also become technology workers
- Please tell me how to decode NEC D78F0034AGC
- MicroPython several issues
- ST NUCLEO-H743ZI Review Summary
- Use the RVB2601 to make a high-precision network timing clock
- What issues should be paid attention to when using a wireless bridge for one-to-many connection?
- Forecast of economic contribution of TD-SCDMA commercialization