The circuit diagram is as follows:
//************************************************************
// Function implemented: Digital tube displays real-time temperature, supports negative temperature
// Chip PIC16F877
// XT:4MHZ
//************************************************************
#include #define LVP 0x3f39 // Crystal: XT; Code: No code protection; Power-on delay timer off; //Low voltage reset disabled; watchdog disabled; low voltage programming disabled __CONFIG (XT & UNPROTECT & PWRTDIS & BORDIS & WDTDIS & LVP); #define uch unsigned char //Give unsigned char an alias name of uch #define DQ RA2 //Define 18B20 data port #define DQ_DIR TRISA2 //Define 18B20D port direction register #define DQ_HIGH() DQ_DIR =1 //Set the data port to input #define DQ_LOW() DQ_DIR = 0; DQ = 0 //Set the data port to output const unsigned char ledcode[12]={0x3F,0x06,0x5B,0x4F,0x66,0x6D,0x7D,0x07,0x7F,0x6F,0x00,0x40}; //Common cathode digital tube 0123456789 segment code without decimal point, positive and negative sign bit const unsigned char ledcode1[12]={0xBF,0x86,0xDB,0xCF,0xE6,0xED,0xFD,0x87,0xFF,0xEF,0x00,0x40}; //Common cathode digital tube with decimal point 0123456789 segment code, positive and negative sign bit void init_port(void); void delay(char x,char y); void delay_1ms(void); void delay_ms(unsigned int time); void interrupt dealtime(); void tmint(void); void timetoseg(uch fh_temp,uch bai_temp,uch shi_temp,uch ge_temp,uch sf_temp,uch bf_temp,uch qf_temp,uch wf_temp); void binary_temp(uch TL , signed char TH); void reset(void); void write_byte(uch val); uch read_byte(void); void get_temp(void); unsigned char display_data[8]; unsigned char intcount=0; uch TLV=0 ; //The collected temperature is 8 bits high uch THV=0; //The collected temperature is 8 bits lower union temp //define a union { int T; uch TV[2]; }temp; signed char TZ=0; //The integer part of the converted temperature value, with a sign bit uch TX=0; //The decimal part of the converted temperature value unsigned int wd; //BCD code format of the converted temperature value unsigned char fh; //sign bit unsigned char bai; // integer hundreds place unsigned char shi; // tens digit unsigned char ge; // integer digit unsigned char shifen; //tenths place unsigned char baifen; //percentile unsigned char qianfen; //thousandths unsigned char wanfen; //ten thousandth place //************************************************************ // Main program //************************************************************ void main(void) { init_port(); tmint(); while(1) { get_temp(); timetoseg(fh,bai,shi,ge,shifen,baifen,qianfen,wanfen); } } //************************************************************ //Port initialization // PORTD is used as digital tube segment driver (high effective) //PORTE is used as digital tube bit selection driver (low effective) //************************************************************ void init_port(void) { RBPU=0; // PORTB = 0xFF; TRISB=0xFF; PORTD=0x00; // TRISC=0x00; //Port C controls the LED indicator, set to output TRISD=0; //Port D is used as a digital tube segment and is set to output ADCON1=0x07; // Make port A and port E all digital I/O ports TRISE=0x00; //Port E is used as the digital tube position selection control pin, set to output PORTE=0x00; } //************************************************************ // Delay procedure //************************************************************ void delay(char x,char y) { char z; do{ z=y; do{;}while(--z); }while(--x); } //The instruction time is: 7+(3*(Y-1)+7)*(X-1). If we add the 7 instructions for calling the function, setting the page, and passing parameters, the instruction time is: 7+(3*(Y-1)+7)*(X-1). //Then it is: 14+(3*(Y-1)+7)*(X-1). //************************************************************ // Delay procedure //************************************************************ void delay_1ms(void) { unsigned int n; for(n=0;n<50;n++) { NOP(); } } //************************************************************ void delay_ms(unsigned int time) { for(;time>0;time--) { delay_1ms(); } } //----------------------------------------------- //Reset DS18B20 function void reset(void) { uch presence=1; while(presence) { DQ_LOW(); //Host pulls to low level delay(2,90); //delay>480503us DQ_HIGH(); //Release the bus and wait for the resistor to pull the bus high and keep it for 15~60us delay(2,8); //delay>60us if(DQ==1) presence=1; //No response signal received, continue to reset else presence=0; //Receive response signal delay(2,70); //delay>240us } } //----------------------------------------------- //Write 18b20 write byte function void write_byte(uch val) { uch i; uch temp; for(i=8;i>0;i--) { temp=val&0x01; //lowest bit shifted out DQ_LOW(); NOP(); NOP(); NOP(); NOP(); NOP(); //Pull from high level to low level to generate write time gap if(temp==1) DQ_HIGH(); //If write 1, pull the level high delay(2,7); //delay 63us DQ_HIGH(); NOP(); NOP(); val=val>>1; //Move right one bit } } //------------------------------------------------ //18b20 read byte function uch read_byte(void) { uch i; uch value=0; //Read the temperature static bit j; for(i=8;i>0;i--) { value>>=1; DQ_LOW(); //Each read time slot is initiated by the host, pulling the bus low for at least 1μs. NOP(); NOP(); NOP(); NOP(); NOP(); NOP(); //6us DQ_HIGH(); //Release the bus within 15μs after the start of the read time slot, pull it to a high level, and prepare to sample the bus. NOP(); NOP(); NOP(); NOP(); NOP(); //5us j=DQ; //sampling bus if(j) value|=0x80; //Put the sampled data into value delay(2,7); //All read time slots are at least 60μs, here about 63us } return(value); } //------------------------------------------------- //Start the temperature conversion function void get_temp() { int i; DQ_HIGH(); reset(); //Reset and wait for slave response write_byte(0XCC); //Ignore ROM match write_byte(0X44); //Send temperature conversion command for(i=10;i>0;i--) { delay(201,132); } reset(); //Reset again and wait for the slave to respond write_byte(0XCC); //Ignore ROM match write_byte(0XBE); //Send the temperature read command TLV=read_byte(); //Read the lower 8 bits of temperature THV=read_byte(); //Read the high 8 bits of temperature DQ_HIGH(); //Release the bus TZ=(TLV>>4)|(THV<<4); //Temperature integer part TX=TLV<<4; //Temperature decimal part, note that the last four digits of TX are invalid binary_temp(TX, TZ ); //Convert the corresponding temperature binary value into a decimal number } //Convert the binary values of the corresponding temperature integer and decimal parts into decimal numbers void binary_temp(char TL , signed char TH) { if(TH>=0) //If it is positive temperature { fh=0x0A; //positive number sign bit bai=TH/100; //hundreds digit of integer shi=(TH%100)/10; //Tens digit //Tens digit ge=(TH%100)%10; //unit digit //unit digit of integer part wd=0; if (TL & 0x80) wd=wd+5000; if (TL & 0x40) wd=wd+2500; if (TL & 0x20) wd=wd+1250; if (TL & 0x10) wd=wd+625; //The above 4 instructions convert the decimal part into BCD code shifen=wd/1000; //tenth place baifen=(wd%1000)/100; //percentile qianfen=(wd%100)/10; //thousandths wanfen=wd%10; //ten thousandth place NOP(); } else //Otherwise, it is a negative temperature, requiring complement { temp.TV[0]=TL;temp.TV[1]=TH; temp.T=(~temp.T)+0x0010; //complement code, add 1 TL=temp.TV[0]; TH=temp.TV[1]; fh=0x0B; //Negative sign bit bai=TH/100; //hundreds digit of integer shi=(TH%100)/10; //Tens digit //Tens digit ge=(TH%100)%10; //unit digit //unit digit of integer part wd=0; if (TL & 0x80) wd=wd+5000; if (TL & 0x40) wd=wd+2500; if (TL & 0x20) wd=wd+1250; if (TL & 0x10) wd=wd+625; //The above 4 instructions convert the decimal part into BCD code shifen=wd/1000; //tenth place baifen=(wd%1000)/100; //percentile qianfen=(wd%100)/10; //thousandths wanfen=wd%10; //ten thousandth place NOP(); } } // Convert each temperature value into segment code //************************************************************ void timetoseg(uch fh_temp,uch bai_temp,uch shi_temp,uch ge_temp,uch sf_temp,uch bf_temp,uch qf_temp,uch wf_temp) { display_data[0] = ledcode[wf_temp]; display_data[1] = ledcode[qf_temp]; display_data[2] = ledcode[bf_temp]; display_data[3] = ledcode[sf_temp]; display_data[4] = ledcode1[ge_temp]; display_data[5] = ledcode[shi_temp]; display_data[6] = ledcode[bai_temp]; display_data[7] = ledcode[fh_temp]; } //************************************************************ // Timer interrupt initialization (OPTION_REG) //************************************************************ void tmint(void) { T0CS=0; //Clock source is internal instruction cycle PSA=0; //The divider is assigned to TMR0 // PS2=0; //The division ratio of TMR0 is 1:16 PS1=1; PS0=1; // GIE=1; //Enable general interrupt T0IE=1; //Enable timer 0 overflow interrupt T0IF=0; // Clear timer 0 interrupt flag TMR0=0X06; //Preset initial value T=(256-6)x16=4000uS } //************************************************************ void interrupt dealtime() //Interrupt entry, this interrupt completes the dynamic scanning of the digital tube { //Each interruption lasts for 4 milliseconds T0IF=0; TMR0=0X06; PORTD = 0x00; //Turn off the display first if(intcount==0) { PORTD = display_data[0]; PORTE=0x00; intcount+=1; } else if(intcount==1) { PORTD = display_data[1]; PORTE=0x01; intcount+=1; } else if(intcount==2) { PORTD = display_data[2]; PORTE=0x02; intcount+=1;
Previous article:PIC microcontroller button controls PWM output LED light brightness C language program
Next article:PIC12F675 MCU LED control program
Recommended ReadingLatest update time:2024-11-16 16:39
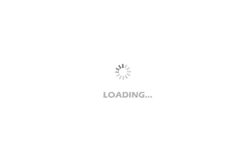
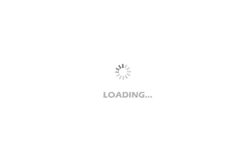
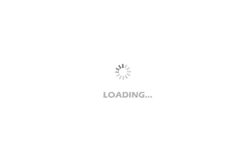
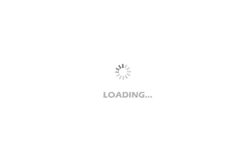
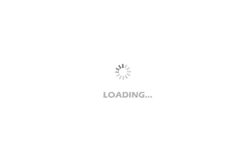
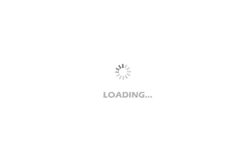
- Popular Resources
- Popular amplifiers
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Single-chip microcomputer technology and application - electronic circuit design, simulation and production (edited by Zhou Runjing)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Communication between Yitong Chuanglian MODBUS to PROFIBUS gateway and Honeywell DCS system
- Let's take a look
- 【TI Wireless】Micro Dual-Mode Wireless Receiver
- PWM and PFM
- Let’s talk about whether Huawei can survive in the end.
- Can the network cable be directly soldered to the PCB without a crystal plug or can the network cable be plugged into the circuit board with terminals?
- [Revenge RVB2601 creative application development] helloworld_beginner's guide to debugging methods and processes
- What changes will occur if RFID technology is applied to clothing production?
- EEWORLD University Hall----30 Case Studies of MATLAB Intelligent Algorithms
- Flyback Power Supply Magnetic Core Calculation Method