//===================================================================
// File Name : 2440lib.c
// Function : S3C2410 PLL,Uart, LED, Port Init
// Date : March 20, 2002
// Version : 0.0
// History
// 0.0 : Programming start (February 20,2002) -> SOP
//===================================================================
#include "def.h"
#include "option.h"
#include "2440addr.h"
#include "2440lib.h"
#include "2440slib.h"
#include #include #include #include #include extern char Image$RW$Limit[]; void *mallocPt=Image$RW$Limit; //***************************[ SYSTEM ]*************************************************** void Delay(int time) { U32 val = (PCLK>>3)/1000-1; rTCFG0 &= ~(0xff<<8); rTCFG0 |= 3<<8; //prescaler = 3+1 rTCFG1 &= ~(0xf<<12); rTCFG1 |= 0<<12; //mux = 1/2 rTCNTB3 = val; rTCMPB3 = val>>1; // 50% rTCON &= ~(0xf<<16); rTCON |= 0xb<<16; //interval, inv-off, update TCNTB3&TCMPB3, start timer 3 rTCON &= ~(2<<16); //clear manual update bit while(time--) { while(rTCNTO3>=val>>1); while(rTCNTO3 }; } //***************************[ PORTS ]**************************************************** void Port_Init0(void) { //CAUTION:Follow the configuration order for setting the ports. // 1) setting value(GPnDAT) // 2) setting control register (GPnCON) // 3) configure pull-up resistor(GPnUP) //32bit data bus configuration //*** PORT A GROUP //Ports : GPA22 GPA21 GPA20 GPA19 GPA18 GPA17 GPA16 GPA15 GPA14 GPA13 GPA12 //Signal : nFCE nRSTOUT nFRE nFWE ALE CLE nGCS5 nGCS4 nGCS3 nGCS2 nGCS1 //Binary : 1 1 1 , 1 1 1 1 , 1 1 1 1 //Ports : GPA11 GPA10 GPA9 GPA8 GPA7 GPA6 GPA5 GPA4 GPA3 GPA2 GPA1 GPA0 //Signal : ADDR26 ADDR25 ADDR24 ADDR23 ADDR22 ADDR21 ADDR20 ADDR19 ADDR18 ADDR17 ADDR16 ADDR0 //Binary : 1 1 1 1 , 1 1 1 1 , 1 1 1 1 rGPACON = 0x7fffff; //**** PORT B GROUP //Ports : GPB10 GPB9 GPB8 GPB7 GPB6 GPB5 GPB4 GPB3 GPB2 GPB1 GPB0 //Signal : nXDREQ0 nXDACK0 nXDREQ1 nXDACK1 nSS_KBD nDIS_OFF L3CLOCK L3DATA L3MODE nIrDATXDEN Keyboard //Setting: INPUT OUTPUT INPUT OUTPUT INPUT OUTPUT OUTPUT OUTPUT OUTPUT OUTPUT OUTPUT //Binary : 00 , 01 00 , 01 00 , 01 01 , 01 01 , 01 01 rGPBCON = 0x044555; rGPBUP = 0x7ff; // The pull up function is disabled GPB[10:0] //*** PORT C GROUP for youlong //Ports : GPC15 GPC14 GPC13 GPC12 GPC11 GPC10 GPC9 GPC8 GPC7 GPC6 GPC5 GPC4 GPC3 GPC2 GPC1 GPC0 //Signal : VD7 VD6 VD5 VD4 VD3 VD2 VD1 VD0 LCDVF2 LCDVF1 LCDVF0 VM VFRAME VLINE VCLK LEND //Binary : 10 10 , 10 10 , 10 10 , 10 10 , 10 10 , 10 10 , 10 10 , 10 10 rGPCCON = 0xaaaaaaaa; rGPCUP = 0xffff; // The pull up function is disabled GPC[15:0] //*** PORT D GROUP //Ports : GPD15 GPD14 GPD13 GPD12 GPD11 GPD10 GPD9 GPD8 GPD7 GPD6 GPD5 GPD4 GPD3 GPD2 GPD1 GPD0 //Signal : VD23 VD22 VD21 VD20 VD19 VD18 VD17 VD16 VD15 VD14 VD13 VD12 VD11 VD10 VD9 VD8 //Binary : 10 10 , 10 10 , 10 10 , 10 10 , 10 10 , 10 10 , 10 10 ,10 10 rGPDCON = 0xaaaaaaaa; rGPDUP = 0xffff; // The pull up function is disabled GPD[15:0] //*** PORT E GROUP //Ports : GPE15 GPE14 GPE13 GPE12 GPE11 GPE10 GPE9 GPE8 GPE7 GPE6 GPE5 GPE4 //Signal : IICSDA IICSCL SPICLK SPIMOSI SPIMISO SDDATA3 SDDATA2 SDDATA1 SDDATA0 SDCMD SDCLK IN //Binary : 10 10 , 10 10 , 10 10 , 10 10 , 10 10 , 10 00 , //------------------------------------------------------------------------------------------------------- //Ports : GPE3 GPE2 GPE1 GPE0 //Signal : IN IN IN IN //Binary : 00 00 , 00 00 //rGPECON = 0xaaaaaaaa; //rGPEUP = 0xffff; // The pull up function is disabled GPE[15:0] rGPECON = 0xaaaaa800; // For added AC97 setting rGPEUP = 0xffff; //*** PORT F GROUP //Ports : GPF7 GPF6 GPF5 GPF4 GPF3 GPF2 GPF1 GPF0 //Signal : nLED_8 nLED_4 nLED_2 nLED_1 nIRQ_PCMCIA EINT2 KBDINT EINT0 //Setting: Output Output Output Output EINT3 EINT2 EINT1 EINT0 //Binary : 01 01 , 01 01 , 10 10 , 10 10 rGPFCON = 0x55aa; rGPFUP = 0xff; // The pull up function is disabled GPF[7:0] //*** PORT G GROUP //Ports : GPG15 GPG14 GPG13 GPG12 GPG11 GPG10 GPG9 GPG8 GPG7 GPG6 //Signal : nYPON YMON nXPON XMON EINT19 DMAMODE1 DMAMODE0 DMASTART KBDSPICLK KBDSPIMOSI //Setting: nYPON YMON nXPON XMON EINT19 Output Output Output SPICLK1 SPIMOSI1 //Binary : 11 11 , 11 11 , 10 01 , 01 01 , 11 11 //----------------------------------------------------------------------------------------- //Ports : GPG5 GPG4 GPG3 GPG2 GPG1 GPG0 //Signal : KBDSPIMISO LCD_PWREN EINT11 nSS_SPI IRQ_LAN IRQ_PCMCIA //Setting: SPIMISO1 LCD_PWRDN EINT11 nSS0 EINT9 EINT8 //Binary : 11 11 , 10 11 , 10 10 rGPGCON = 0xff95ffba; rGPGUP = 0xffff; // The pull up function is disabled GPG[15:0] //*** PORT H GROUP //Ports : GPH10 GPH9 GPH8 GPH7 GPH6 GPH5 GPH4 GPH3 GPH2 GPH1 GPH0 //Signal : CLKOUT1 CLKOUT0 UCLK nCTS1 nRTS1 RXD1 TXD1 RXD0 TXD0 nRTS0 nCTS0 //Binary : 10 , 10 10 , 11 11 , 10 10 , 10 10 , 10 10 rGPHCON = 0x2afaaa; rGPHUP = 0x7ff; // The pull up function is disabled GPH[10:0] // Added for S3C2440X, DonGo //*** PORT J GROUP //Ports : GPJ12 GPJ11 GPJ10 GPJ9 GPJ8 GPJ7 GPJ6 GPJ5 GPJ4 GPJ3 GPJ2 GPJ1 GPJ0 //Signal : CAMRESET CAMPCLKOUT CAMHREF CAMVSYNC CAMPCLKIN CAMDAT[7] CAMDAT[6] CAMDAT[5] CAMDAT[4] CAMDAT[3] CAMDAT[2] CAMDAT[1] CAMDAT[0] //Binary : 10 10 10 10 10 10 10 10 10 10 10 10 10 rGPJCON = 0x02aaaaaa; rGPJUP = 0x1fff; // The pull up function is disabled GPH[10:0] //External interrupt will be falling edge triggered. rEXTINT0 = 0x22222222; // EINT[7:0] rEXTINT1 = 0x22222222; // EINT[15:8] rEXTINT2 = 0x22222222; // EINT[23:16] } void Port_Init(void) { //CAUTION:Follow the configuration order for setting the ports. // 1) setting value(GPnDAT) // 2) setting control register (GPnCON) // 3) configure pull-up resistor(GPnUP) //32bit data bus configuration //*** PORT A GROUP //Ports : GPA22 GPA21 GPA20 GPA19 GPA18 GPA17 GPA16 GPA15 GPA14 GPA13 GPA12 //Signal : nFCE nRSTOUT nFRE nFWE ALE CLE nGCS5 nGCS4 nGCS3 nGCS2 nGCS1 //Binary : 1 1 1 , 1 1 1 1 , 1 1 1 1 //Ports : GPA11 GPA10 GPA9 GPA8 GPA7 GPA6 GPA5 GPA4 GPA3 GPA2 GPA1 GPA0 //Signal : ADDR26 ADDR25 ADDR24 ADDR23 ADDR22 ADDR21 ADDR20 ADDR19 ADDR18 ADDR17 ADDR16 ADDR0 //Binary : 1 1 1 1 , 1 1 1 1 , 1 1 1 1 rGPACON = 0x7fffff; //**** PORT B GROUP //Ports : GPB10 GPB9 GPB8 GPB7 GPB6 GPB5 GPB4 GPB3 GPB2 GPB1 GPB0 //Signal : nXDREQ0 nXDACK0 nXDREQ1 nXDACK1 nSS_KBD nDIS_OFF L3CLOCK L3DATA L3MODE nIrDATXDEN Keyboard //Setting: INPUT OUTPUT INPUT OUTPUT INPUT OUTPUT OUTPUT OUTPUT OUTPUT OUTPUT OUTPUT //Binary : 00 , 01 00 , 01 00 , 01 01 , 01 01 , 01 01 //rGPBCON = 0x000150;(youlong) rGPBCON = 0x015550; rGPBUP = 0x7ff; // The pull up function is disabled GPB[10:0] //*** PORT C GROUP for youlong //Ports : GPC15 GPC14 GPC13 GPC12 GPC11 GPC10 GPC9 GPC8 GPC7 GPC6 GPC5 GPC4 GPC3 GPC2 GPC1 GPC0 //Signal : VD7 VD6 VD5 VD4 VD3 VD2 VD1 VD0 LCDVF2 LCDVF1 LCDVF0 VM VFRAME VLINE VCLK LEND //Binary : 10 10 , 10 10 , 10 10 , 10 10 , 10 10 , 10 10 , 10 10 , 10 10 //rGPCCON = 0xaaaaaaaa; //rGPCUP = 0xffff; // The pull up function is disabled GPC[15:0] //*** PORT C GROUP //Ports : GPC15 GPC14 GPC13 GPC12 GPC11 GPC10 GPC9 GPC8 GPC7 GPC6 GPC5 GPC4 GPC3 GPC2 GPC1 GPC0 //Signal : VD7 VD6 VD5 VD4 VD3 VD2 VD1 VD0 LCDVF2 LCDVF1 LCDVF0 VM VFRAME VLINE VCLK LEND //Binary : 10 10 , 10 10 , 10 10 , 10 01 , 01 01 , 01 10 , 10 10 , 10 10 rGPCCON = 0xaaa956aa; rGPCUP = 0xffff; // The pull up function is disabled GPC[15:0] //*** PORT D GROUP //Ports : GPD15 GPD14 GPD13 GPD12 GPD11 GPD10 GPD9 GPD8 GPD7 GPD6 GPD5 GPD4 GPD3 GPD2 GPD1 GPD0 //Signal : VD23 VD22 VD21 VD20 VD19 VD18 VD17 VD16 VD15 VD14 VD13 VD12 VD11 VD10 VD9 VD8 //Binary : 10 10 , 10 10 , 10 10 , 10 10 , 10 10 , 10 10 , 10 10 ,10 10 rGPDCON = 0xaaaaaaaa; rGPDUP = 0xffff; // The pull up function is disabled GPD[15:0] //*** PORT E GROUP //Ports : GPE15 GPE14 GPE13 GPE12 GPE11 GPE10 GPE9 GPE8 GPE7 GPE6 GPE5 GPE4 //Signal : IICSDA IICSCL SPICLK SPIMOSI SPIMISO SDDATA3 SDDATA2 SDDATA1 SDDATA0 SDCMD SDCLK IN //Binary : 10 10 , 10 10 , 10 10 , 10 10 , 10 10 , 10 00 , //-------------------------------------------------------------------------------------------------------
Previous article:Samsung 2440 ARM initialization
Next article:ARM development steps: After reading this, the development ideas will be clearer
Recommended ReadingLatest update time:2024-11-16 09:50
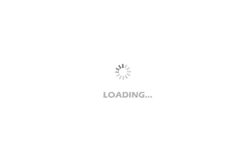
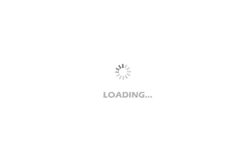
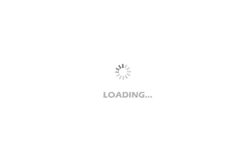
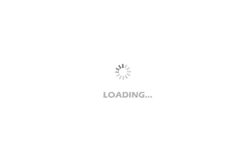
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications