#include "iom16v.h"
/*I2C bus master mode error handling*/
void error(unsigned char type) {
switch (type & 0xF8) {
case 0x20: /*Address write failed*/
/*stop*/
TWCR = (1 << TWINT) | (1 << TWEN) | (1 << TWSTO);
break;
case 0x30: /*Data write failed*/
/*stop*/
TWCR = (1 << TWINT) | (1 << TWEN) | (1 << TWSTO);
break;
case 0x38: /*Arbitration failed*/
break;
case 0x48: /*Address read failed*/
/*stop*/
TWCR = (1 << TWINT) | (1 << TWEN) | (1 << TWSTO);
break;
}
}
/*I2C bus single byte write*/
unsigned char twi_write(unsigned char addr, unsigned char dd) {
TWBR = 2;
/*start*/
TWCR = (1 << TWINT) | (1 << TWSTA) | (1 << TWEN);
while (!(TWCR & (1 << TWINT)));
if ((TWSR & 0xF8) != 0x08) {
error(TWSR);
return 0;
}
/*SLA_W chip address*/
TWDR = 0xA0;
TWCR = (1 << TWINT) | (1 << TWEN);
while (!(TWCR & (1 << TWINT)));
if ((TWSR & 0xF8) != 0x18) {
error(TWSR);
return 0;
}
/*addr operation address*/
TWDR = addr;
TWCR = (1 << TWINT) | (1 << TWEN);
while (!(TWCR & (1 << TWINT)));
if ((TWSR & 0xF8) != 0x28) {
error(TWSR);
return 0;
}
/*dd write data*/
TWDR = dd;
TWCR = (1 << TWINT) | (1 << TWEN);
while (!(TWCR & (1 << TWINT)));
if ((TWSR & 0xF8) != 0x28) {
error(TWSR);
return 0;
}
/*stop*/
TWCR = (1 << TWINT) | (1 << TWEN) | (1 << TWSTO);
return 1;
}
/*I2C bus single byte read*/
unsigned char twi_read(unsigned char addr, unsigned char *dd) {
TWBR = 2;
/*start*/
TWCR=(1< error(TWSR); return 0; } /*SLA_W chip address*/ TWDR = 0xA0; TWCR = (1 << TWINT) | (1 << TWEN); while (!(TWCR & (1 << TWINT))); if ((TWSR & 0xF8) != 0x18) { error(TWSR); return 0; } /*addr operation address*/ TWDR = addr; TWCR = (1 << TWINT) | (1 << TWEN); while (!(TWCR & (1 << TWINT))); if ((TWSR & 0xF8) != 0x28) { error(TWSR); return 0; } /*start*/ TWCR = (1 << TWINT) | (1 << TWSTA) | (1 << TWEN); while (!(TWCR & (1 << TWINT))); if ((TWSR & 0xF8) != 0x10) { error(TWSR); return 0; } /*SLA_R chip address*/ TWDR = 0xA1; TWCR = (1 << TWINT) | (1 << TWEN); while (!(TWCR & (1 << TWINT))); if ((TWSR & 0xF8) != 0x40) { error(TWSR); return 0; } /*Read data*/ TWCR = (1 << TWINT) | (1 << TWEN); while (!(TWCR & (1 << TWINT))); if ((TWSR & 0xF8) != 0x58) { error(TWSR); return 0; } *dd = TWDR; /*stop*/ TWCR = (1 << TWINT) | (1 << TWSTO) | (1 << TWEN); return 1; }
Previous article:Atmega16 based voltage meter production program + schematic diagram
Next article:4-bit independent keyboard control program of Atmega128a
Recommended ReadingLatest update time:2024-11-16 13:28
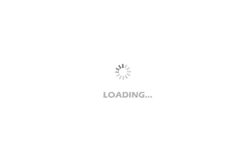
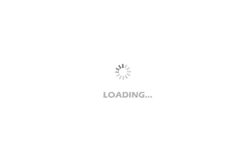
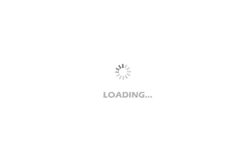
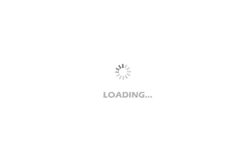
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Problems encountered in debugging the Southchip SC8721 high-efficiency synchronous buck-boost converter
- (A-Current signal detection device) Chongqing "TI Cup" Award_Chongqing University of Posts and Telecommunications
- This appears and I can't choose it. It's grayed out.
- 【AT-START-F403A Review】3. Try USB Composite_Device
- Communication system cannot do without filter series 2
- [Sipeed LicheeRV 86 Panel Review] 10. LVGL Timer
- Today at 10:00 AM, live broadcast with prizes: Protecting clean water sources - ADI water quality monitoring solutions
- [Ateli Development Board AT32F421 Review] + Unboxing and Learning Plan
- What are risc-v chips?
- sensortile box source code