Interrupt Classification
The STM32 EXTI controller supports 19 external interrupt/event requests. Each interrupt has a status bit, and each interrupt/event has independent trigger and mask settings.
The 19 external interrupts of STM32 correspond to 19 interrupt lines, namely EXTI_Line0-EXTI_Line18:
Lines 0~15: Corresponding to the input interrupt of the external IO port.
Line 16: Connect to PVD output.
Line 17: Connected to the RTC alarm event.
Line 18: Connected to USB wakeup event.
Trigger mode: The external interrupt of STM32 is triggered by edge and does not support level trigger.
External interrupt grouping: Each GPIO of STM32 can be configured as an external interrupt trigger source. STM32 divides many interrupt trigger sources into different groups according to the pin numbers. For example, PA0, PB0, PC0, PD0, PE0, PF0, PG0 are the first group, and so on. We can conclude that there are 16 groups in total. STM32 stipulates that only one interrupt trigger source can work at the same time in each group, so the maximum number of external interrupts that can work is 16.
Register Group
There are 4 EXTICR registers in total. Since the compiler registers are numbered starting from 0, EXTICR[0]~EXTICR[3] correspond to EXTICR1~EXTICR 4 in the STM32 Reference Manual (it took me a long time to figure out the meaning of this array!!). Each EXTICR only uses its lower 16 bits.
The allocation of EXTICR[0] ~EXTICR[3] is as follows:
The structure of the EXTI register:
typedef struct
{
vu32 IMR;
vu32 EMR;
vu32 RTSR;
vu32 FTSR;
vu32 HEAVY;
vu32 PR;
} EXIT_TypeDef;
IMR: Interrupt Mask Register
This is a 32-bit register. But only the first 19 bits are valid. When bit x is set to 1, the interrupt on this line is enabled, otherwise the interrupt on this line is disabled.
EMR: Event Mask Register
Same as IMR, except that this register is used to mask and enable events.
RTSR: rising edge trigger selection register
This register is the same as IMR, and is also a 32-bit register, with only the first 19 bits being valid. Bit x corresponds to the rising edge trigger on line x. If set to 1, rising edge trigger interrupts/events are allowed. Otherwise, it is not allowed.
FTSR: Falling Edge Trigger Selection Register
Same as PTSR, but this register is set to the falling edge. The falling edge and the rising edge can be set at the same time, so it becomes an arbitrary level trigger.
SWIER: Software Interrupt Event Register
By writing 1 to bit x of this register, the corresponding bit in PR will be set to pending when IMR and EMR are not set. If IMR and EMR are set, an interrupt will be generated. The set SWIER bit will be cleared after the corresponding bit in PR is cleared.
PR: Pending Register
0, indicating that no trigger request occurs on the corresponding line.
1, indicating that the selected edge event has occurred on the external interrupt line. This bit can be cleared by writing 1 to the corresponding bit of this register.
In the interrupt service function, it is often necessary to write 1 to the corresponding bit of this register to clear the interrupt request.
Interrupt Configuration Steps
Each IO port of STM32 can be used as an interrupt input, which is very useful. To use an IO port as an external interrupt input, there are several steps:
1) Initialize the IO port as input.
In this step, you set the state of the IO port you want to use as the external interrupt input. You can set it to pull-up/pull-down input or floating input, but when floating, you must use an external pull-up or pull-down resistor. Otherwise, the interrupt may be triggered continuously. In places with large interference, even if you use pull-up/pull-down, it is recommended to use an external pull-up/pull-down resistor, which can prevent the influence of external interference to a certain extent.
2) Enable the IO port multiplexing clock and set the mapping relationship between the IO port and the interrupt line.
The correspondence between the STM32 IO port and the interrupt line needs to configure the external interrupt configuration register EXTICR, so we need to first turn on the multiplexed clock, and then configure the correspondence between the IO port and the interrupt line. Only then can the external interrupt be connected to the interrupt line.
3) Enable the online interrupt/event corresponding to the IO port and set the trigger condition.
In this step, we need to configure the conditions for interrupt generation. STM32 can be configured as rising edge trigger, falling edge trigger, or any level change trigger, but it cannot be configured as high level trigger or low level trigger. Configure according to your actual situation. At the same time, you need to enable the interrupt on the interrupt line. It should be noted that if you use an external interrupt and set the EMR bit of the interrupt, the software simulation will not be able to jump to the interrupt, but the hardware can. Without setting EMR, the software simulation can enter the interrupt service function, and the hardware can also do so. It is recommended not to configure the EMR bit.
4) Configure the interrupt group (NVIC) and enable interrupts.
In this step, we configure the interrupt grouping and enable it. For STM32 interrupts, only when the NVIC settings are configured and enabled can they be executed, otherwise they will not be executed into the interrupt service function. For a detailed introduction to NVIC, please refer to the previous chapter.
5) Write the interrupt service function.
This is the last step of interrupt setting. The interrupt service function is essential. If the interrupt is enabled in the code but the interrupt service function is not written, it may cause a hardware error, which may cause the program to crash! So after enabling an interrupt, you must remember to write a service function for the interrupt. Write the operation you want to perform after the interrupt in the interrupt service function.
The procedure is as follows:
void EXIT8_IPRT()
{
RCC->APB2ENR |= RCC_APB2ENR_AFIOEN;
EXTI->IMR = EXTI_IMR_MR8;
EXTI->RTSR = EXTI_RTSR_TR8;
AFIO->EXTICR[2] = AFIO_EXTICR3_EXTI8_PA;
NVIC_EnableIRQ(EXTI9_5_IRQn);
}
void GPIOA8_Init()
{
/* 1. ENABLE GPIOA CLOCK */
RCC->APB2ENR |= RCC_APB2ENR_IOPAEN;
/* 2. CONFIG PA8*/
GPIOA->CRH &= ~(GPIO_CRH_MODE8 | GPIO_CRH_CNF8);
GPIOA->CRH |= GPIO_CRH_CNF8_1;
EXIT8_IPRT();
while(1)
{
}
}
Debug as follows
1) Initialize the IO port as input.
2) Enable the IO port multiplexing clock and set the mapping relationship between the IO port and the interrupt line.
3) Enable the online interrupt/event corresponding to the IO port and set the trigger condition.
4) Configure the interrupt group (NVIC) and enable interrupts.
5) Write the interrupt service function.
void EXTI9_5_IRQHandler(void)
{
Delay_ms(10);
if((OUTPUT->PR) & OUTPUT_PR_PR8)
{
EXTI->PR = EXTI_PR_PR8;
printf("Monitor rising\n");
}
}
Previous article:How to solve the problem that the STM32F407 serial port cannot send the first byte
Next article:【stm32f103】USART TX transmission implementation (register version)
Recommended ReadingLatest update time:2024-11-16 13:32
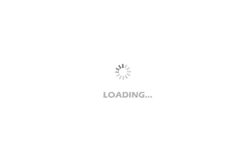
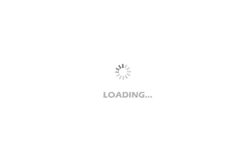
![Write STM32 OS step by step [IV] OS basic framework](https://6.eewimg.cn/news/statics/images/loading.gif)
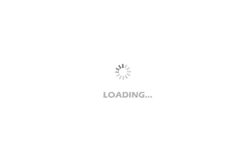
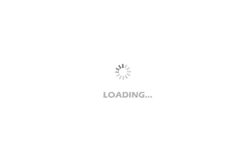
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- An article to understand the analysis and calculation of electrolytic capacitor life
- FreeRTOS in the JIHAI APM32E103VET6 review
- Wu Jianying's live video sharing of microcontroller lectures
- ESP32-C3 Debug Diary
- LPS28DFW driver and related information
- Battery Test Equipment --- Signal Chain
- Summary of basic issues of ADC
- Share: Debugging of SIM7020 and NB-IOT
- mini risc mcu source code
- The design application of TGA2509 is not particularly ideal