virt_net.c driver:
// Reference drivers\net\cs89x0.c
#include "linux/module.h"
#include "linux/errno.h"
#include "linux/netdevice.h"
#include "linux/etherdevice.h"
#include "linux/kernel.h"
#include "linux/types.h"
#include "linux/fcntl.h"
#include "linux/interrupt.h"
#include "linux/ioport.h"
#include "linux/in.h"
#include "linux/skbuff.h"
#include "linux/slab.h"
#include "linux/spinlock.h"
#include "linux/string.h"
#include "linux/init.h"
#include "linux/bitops.h"
#include "linux/delay.h"
#include "linux/ip.h"
#include "asm/system.h"
#include "asm/io.h"
#include "asm/irq.h"
static struct net_device *vnet_dev;
static void emulator_rx_packet(struct sk_buff *skb, struct net_device *dev)
{
// Refer to LDD3
unsigned char *type;
struct iphdr *ih;
__be32 *saddr, *daddr, tmp;
unsigned char tmp_dev_addr[ETH_ALEN];
struct ethhdr *ethhdr;
struct sk_buff *rx_skb;
// Read/save data from hardware
// Swap the mac addresses of "source/destination"
ethhdr = (struct ethhdr *)skb->data;
memcpy(tmp_dev_addr, ethhdr->h_dest, ETH_ALEN);
memcpy(ethhdr->h_dest, ethhdr->h_source, ETH_ALEN);
memcpy(ethhdr->h_source, tmp_dev_addr, ETH_ALEN);
// Swap the "source/destination" IP addresses
ih = (struct iphdr *)(skb->data + sizeof(struct ethhdr));
saddr = &ih->saddr;
daddr = &ih->daddr;
tmp = *saddr;
*saddr = *daddr;
*daddr = tmp;
//((u8 *)saddr)[2] ^= 1; // change the third octet (class C)
//((u8 *)daddr)[2] ^= 1;
type = skb->data + sizeof(struct ethhdr) + sizeof(struct iphdr);
//printk("tx package type = x\n", *type);
// Modify the type, originally 0x8 means ping
*type = 0; // 0 means reply
ih->check = 0; // and rebuild the checksum (ip needs it)
ih->check = ip_fast_csum((unsigned char *)ih,ih->ihl);
//Construct a sk_buff
rx_skb = dev_alloc_skb(skb->len + 2);
skb_reserve(rx_skb, 2); // align IP on 16B boundary
memcpy(skb_put(rx_skb, skb->len), skb->data, skb->len);
// Write metadata, and then pass to the receive level
rx_skb->dev = dev;
rx_skb->protocol = eth_type_trans(rx_skb, dev);
rx_skb->ip_summed = CHECKSUM_UNNECESSARY; // don't check it
dev->stats.rx_packets++;
dev->stats.rx_bytes += skb->len;
// Submit sk_buff
netif_rx(rx_skb);
}
static int virt_net_send_packet(struct sk_buff *skb, struct net_device *dev)
{
static int cnt = 0;
printk("virt_net_send_packet cnt = %d\n", ++cnt);
// For the real network card, send the data in skb through the network card
netif_stop_queue(dev); // Stop the queue of the network card
// ...... // Write skb data to the network card
//Construct a fake sk_buff and report it
emulator_rx_packet(skb, dev);
dev_kfree_skb (skb); // release skb
netif_wake_queue(dev); //After all data is sent out, wake up the queue of the network card
// Update statistics
dev->stats.tx_packets++;
dev->stats.tx_bytes += skb->len;
return 0;
}
static const struct net_device_ops virt_netdev_ops = {
.ndo_start_xmit = virt_net_send_packet,
};
static int virt_net_init(void)
{
// 1. Allocate a net_device structure
vnet_dev = alloc_netdev(0, "vnet%d", ether_setup); // alloc_etherdev
// 2. Setup
vnet_dev->netdev_ops = &virt_netdev_ops;
// Set the MAC address
vnet_dev->dev_addr[0] = 0x08;
vnet_dev->dev_addr[1] = 0x89;
vnet_dev->dev_addr[2] = 0x89;
vnet_dev->dev_addr[3] = 0x89;
vnet_dev->dev_addr[4] = 0x89;
vnet_dev->dev_addr[5] = 0x11;
// Set the following two items to ping successfully
vnet_dev->flags |= IFF_NOARP;
vnet_dev->features |= NETIF_F_NO_CSUM;
// 3. Registration
//register_netdevice(vnet_dev);
register_netdev(vnet_dev);
return 0;
}
static void virt_net_exit(void)
{
unregister_netdev(vnet_dev);
free_netdev(vnet_dev);
}
module_init(virt_net_init);
module_exit(virt_net_exit);
MODULE_AUTHOR("thisway.diy@163.com,17653039@qq.com");
MODULE_LICENSE("GPL");
Previous article:Tiny210 IIC driver at24cxx access
Next article:USB device driver for Tiny210
Recommended ReadingLatest update time:2024-11-16 12:25
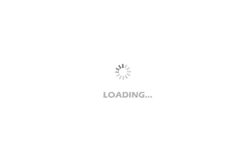
- Popular Resources
- Popular amplifiers
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
Modern Product Design Guide
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- 【New Year's Festival Competition】+ Watching Lanterns on the Lantern Festival (multiple photos)
- 5G small base stations are under the spotlight: now is the eve of the outbreak
- 【CH579M-R1】+ PWM breathing light
- [Experience] [Free trial of Letuo USB oscilloscope] Comparison test of Letuo USB oscilloscope
- Wi-Fi 6E scarcity causes enterprises to delay upgrades until Wi-Fi 7 devices ship
- [RISC-V MCU CH32V103 Evaluation] W25Q16 Reading, Writing and Application
- 【GD32E231 DIY Contest】1. USART0
- When a plane crashes, is there any technology that can completely guarantee the safety of passengers?
- STMicroelectronics launches the second generation multi-zone time-of-flight sensor VL53L8
- EEWORLD University - Wildfire FreeRTOS Kernel Implementation and Application Development Practical Guide