Let's take a look at our time.c file. The code of the timer.c file is as follows:
//General timer 3 interrupt initialization
//arr: automatic reload value. psc: clock pre-division number
//Timer overflow time calculation method: Tout=((arr+1)*(psc+1))/Ft us.
//Ft=timer operating frequency, unit: Mhz
//Timer 3 is used here!
void TIM3_Int_Init(u16 arr,u16 psc)
{
TIM_TimeBaseInitTypeDef TIM_TimeBaseInitStructure;
NVIC_InitTypeDef NVIC_InitStructure;
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM3,ENABLE); //Enable TIM3 clock
TIM_TimeBaseInitStructure.TIM_Period = arr; //Automatically reload value
TIM_TimeBaseInitStructure.TIM_Prescaler=psc; //Timer frequency division
TIM_TimeBaseInitStructure.TIM_CounterMode=TIM_CounterMode_Up; //Upward counting mode
TIM_TimeBaseInitStructure.TIM_ClockDivision=TIM_CKD_DIV1;
TIM_TimeBaseInit(TIM3,&TIM_TimeBaseInitStructure); // Initialize timer TIM3
TIM_ITConfig(TIM3,TIM_IT_Update,ENABLE); //Enable timer 3 update interrupt
NVIC_InitStructure.NVIC_IRQChannel=TIM3_IRQn; //Timer 3 interrupt
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority=0x01; //Preemption priority 1
NVIC_InitStructure.NVIC_IRQChannelSubPriority=0x03; //Response priority 3
NVIC_InitStructure.NVIC_IRQChannelCmd=ENABLE;
NVIC_Init(&NVIC_InitStructure); // Initialize NVIC
TIM_Cmd(TIM3,ENABLE); //Enable timer 3
}
//Timer 3 interrupt service function
void TIM3_IRQHandler(void)
{
if(TIM_GetITStatus(TIM3,TIM_IT_Update)==SET) // Overflow interrupt
{
LED1=!LED1;
}
TIM_ClearITPendingBit(TIM3,TIM_IT_Update); //Clear interrupt flag
}
This file contains an interrupt service function and a timer 3 interrupt initialization function. The interrupt service function is relatively simple. After each interrupt, the interrupt type of TIM3 is determined. If the interrupt type is correct, the flip of LED1 (DS1) is executed. The TIM3_Int_Init function executes the 5 steps we introduced above to make TIM3 start working and enable interrupts. Here we use the label ~ to mark the five steps of timer initialization. The 2 parameters of this function are used to set the overflow time of TIM3. Because the APB1 clock has been initialized to 4 divisions in the system initialization SystemInit function, the APB1 clock is 42M. From the internal clock tree diagram of STM32F4 (Figure 4.3.1.1), we know that when the APB1 clock division number is 1, the clocks of TIM2~7 and TIM12~14 are the clocks of APB1. If the APB1 clock division number is not 1, the clock frequencies of TIM2~7 and TIM12~14 will be twice the APB1 clock. Therefore, the clock of TIM3 is 84M. Based on the values of arr and psc we designed, we can calculate the interrupt time. The calculation formula is as follows:
Tout= ((arr+1)*(psc+1))/Tclk;
in:
Tclk: Input clock frequency of TIM3 (in MHz).
Tout: TIM3 overflow time (in us).
The content of the timer.h header file is relatively simple, so we will not explain it here.
Finally, let's look at the main function code as follows:
int main(void)
{
NVIC_PriorityGroupConfig(NVIC_PriorityGroup_2); //Set system interrupt priority group 2
delay_init(168); //Initialize delay function
LED_Init(); //Initialize LED port
TIM3_Int_Init(5000-1,8400-1); //Timer clock 84M, frequency division factor 8400, so 84M/8400=10Khz
//Counting frequency, counting 5000 times is 500ms
while(1)
{
LED0=!LED0;
delay_ms(200); //delay 200ms
};
}
The code here is similar to the previous one. After initializing TIM3, this code enters an infinite loop waiting for the TIM3 overflow interrupt. When the value of TIM3_CNT is equal to the value of TIM3_ARR, a TIM3 update interrupt is generated, and then LED1 is negated in the interrupt, and TIM3_CNT starts counting from 0 again.
Here, the timer timing duration of 500ms is calculated as follows: the timer clock is 84Mhz, and the division factor is 8400, so the counting frequency after division is 84Mhz/8400=10KHz, and then it counts to 5000, so the duration is 5000/10000=0.5s, which is 500ms.
Previous article:STM32f4 PWM output experimental code
Next article:STM32 program transplantation_internal flash boot times management lib library establishment
Recommended ReadingLatest update time:2024-11-16 14:51
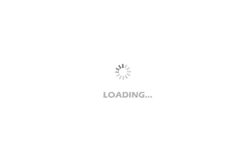
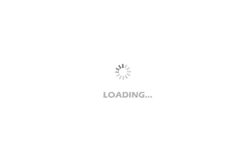
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Share my fish farming process
- Let's unbox the LAUNCHXL-F28379D
- 【McQueen Trial】Python Programming (1)
- 9 tests and methods that must be performed on switching power supplies
- This week's topic: Let's talk about PID and share your experience. Two pieces of exquisite PID materials are included
- Shanghai is urgently recruiting FPGA development engineers with generous benefits! ! ! !
- Motor control circuit, MOS burnt out
- Design of filter module based on combination of single chip microcomputer and FPGA
- How to dissipate heat as a whole on the circuit board while ensuring that it is waterproof as a whole? The main reason is that the MOS tube of the patch generates the most heat and steps up and down the voltage.
- How to solve the problem that H file cannot be opened in IAR