The pwm.c source file code is as follows:
//TIM14 PWM initialization
//PWM output initialization
//arr: automatic reload value psc: clock pre-division number
void TIM14_PWM_Init(u32 arr,u32 psc)
{
GPIO_InitTypeDef GPIO_InitStructure;
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure;
TIM_OCInitTypeDef TIM_OCInitStructure;
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM14,ENABLE); //TIM14 clock enable
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOF, ENABLE); //Enable PORTF clock
GPIO_PinAFConfig(GPIOF,GPIO_PinSource9,GPIO_AF_TIM14); //GF9 is multiplexed as TIM14
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9; //GPIOF9
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF; //Multiplexing function
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_100MHz; //速度 50MHz
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP; //Push-pull multiplexing output
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP; //上拉
GPIO_Init(GPIOF,&GPIO_InitStructure); //Initialize PF9
TIM_TimeBaseStructure.TIM_Prescaler=psc; //Timer frequency division
TIM_TimeBaseStructure.TIM_CounterMode=TIM_CounterMode_Up; //Upward counting mode
TIM_TimeBaseStructure.TIM_Period=arr; //Automatically reload value
TIM_TimeBaseStructure.TIM_ClockDivision=TIM_CKD_DIV1;
TIM_TimeBaseInit(TIM14,&TIM_TimeBaseStructure); //Initialize timer 14
//Initialize TIM14 Channel1 PWM mode
TIM_OCInitStructure.TIM_OCMode = TIM_OCMode_PWM1; //PWM modulation mode 1
TIM_OCInitStructure.TIM_OutputState = TIM_OutputState_Enable; //Comparison output enable
TIM_OCInitStructure.TIM_OCPolarity = TIM_OCPolarity_Low; //Output polarity low
TIM_OC1Init(TIM14, &TIM_OCInitStructure); //Initialize peripheral TIM1 4OC1
TIM_OC1PreloadConfig(TIM14, TIM_OCPreload_Enable); //Enable preload register
TIM_ARRPreloadConfig(TIM14,ENABLE);//ARPE enable
TIM_Cmd(TIM14, ENABLE); //Enable TIM14
}
This part of the code contains the first 5 steps of the PWM output settings introduced above. We will not talk about the settings of TIM14 here.
Next, let's look at the main function in the main program as follows:
int main(void)
{
u16 led0pwmval=0;
u8 dir=1;
NVIC_PriorityGroupConfig(NVIC_PriorityGroup_2); //Set system interrupt priority group 2
delay_init(168); //Initialize delay function
uart_init(115200); //Initialize the serial port baud rate to 115200
TIM14_PWM_Init(500-1,84-1); //The timer clock is 84M, the frequency division factor is 84, so the counting frequency
//84M/84=1Mhz, reload value 500, so PWM frequency is 1M/500=2Khz.
while(1)
{
delay_ms(10);
if(dir)led0pwmval++; //dir==1 led0pwmval increments
else led0pwmval--; //dir==0 led0pwmval decrement
if(led0pwmval>300)dir=0; //After led0pwmval reaches 300, the direction is decreasing
if(led0pwmval==0)dir=1; //After led0pwmval decreases to 0, the direction changes to increasing
TIM_SetCompare1(TIM14,led0pwmval); //Modify comparison value and duty cycle
}
}
Here, we can see from the infinite loop function that we set the value of led0pwmval as the PWM comparison value, that is, we control the duty cycle of PWM through led0pwmval, and then control the value of led0pwmval from 0 to 300, and then from 300 to 0, and so on. Therefore, the brightness of DS0 will also change from dark to bright, and then from bright to dark as the duty cycle of the signal changes. As for the value here, why we take 300, because when the output duty cycle of PWM reaches this value, the brightness of our LED will not change much (although the maximum value can be set to 499), so it is unnecessary to design a value that is too large here. At this point, our software design is completed.
Previous article:STM32f4 input capture experimental code
Next article:STM32f4 timer interrupt experimental code
Recommended ReadingLatest update time:2024-11-16 12:42
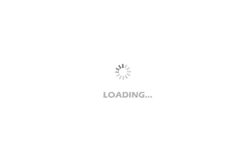
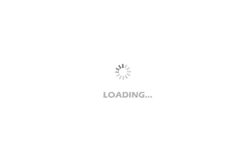
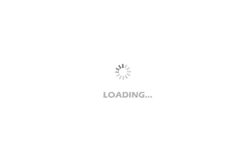
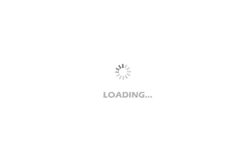
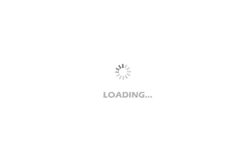
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- CCS import routine for TM4C123x
- EEWORLD University Hall----Signal Integrity and High-Speed Digital Circuit Design
- Competition Schedule [Updated]
- Output impedance problem of parallel reference voltage
- Master Python web crawler core technology, framework and project practice PDF HD full version free download
- position2Go Review 2: Interlude
- Design of AC voltage measurement based on MSP430 microcontroller
- 【Beetle ESP32-C3】4. Luat-OS environment deployment
- A brief discussion on the differences between x86, DSP and SPARC
- ESP32-C3 RISC-V microcontroller information leak