1.warning: #550-D: variable "d" was set but never used
Description: The variable 'd' is defined but never used, or, although you use this variable, the compiler thinks that the statement where the variable d is located is meaningless, and the compiler optimizes it.
Solution: Carefully consider whether the defined variable d is useful. If you believe that the statement where the variable d is located is meaningful, try to modify the variable d with the volatile keyword. If it is really useless, delete it to release possible memory.
2.warning: #1-D: last line of file ends without a newline
Description: The last line of the file is not a new line. The compiler requires that the last line of the program file must be a blank line. I have been thinking about it for a long time but I can't figure out why it is like this.
Solution: You can ignore it. If you feel uncomfortable with the warning, then press Enter on the last line of the file where the warning appears to leave a blank line.
3. warning: #111-D: statement is unreachable
Description: The statement is impossible to reach. It often appears in this situation:
int main(void)
{
...
while(1) // infinite loop, which is most common in programs that do not use the operating system
{
...
}
return 0; // This statement is impossible to execute under normal circumstances, and the compiler issues a warning
}
Solution: Ignore it.
4. warning: C3017W: data may be used before being set
Description: The variable 'data' is not explicitly assigned a value before use. For example:
uint8 i,data; //Define variables i and data, neither of which is explicitly assigned a value
for ( i = 0; i < 8; i++) // variable 'i' is assigned 0 in the statement
{
if ( IO1PIN & SO_CC2420 )
data |= 0x01; // variable 'data' is not explicitly assigned before use, compiler issues a warning
else
data &= ~0x01;
}
Solution: Carefully consider whether the initial value of the variable is 0. If so, you can ignore this warning, because the MDK compiler will initialize the used data area to 0 before the program is executed. However, if the initial value of the variable should not be 0, ignoring this warning may cause a fatal error. This warning should be taken seriously. You should develop the habit of assigning initial values to variables, fortunately, the compiler will check it.
5. warning: #177-D: variable "temp" was declared but never referenced
Description: The variable 'temp' was declared but not referenced. This usually happens when a variable is declared but not used. The difference between it and warning: #550-D: variable "temp" was set but never used is that temp has never been used.
Solution: If the defined variable is really useless, delete it; if it is useful, use it in the program.
Similar to this warning is warning: #177-D: function "MACProcessBeacon" was declared but never referenced
6. warning: #940-D: missing return statement at end of non-void function "DealwithInspect2"
Description: The function "DealwithInspect2" that returns non-void value is missing the return value statement at the end. For example:
int DealwithInspect2(uint32 test)
{
...
...
...
//This should be return x; return an int data type. If there is no return value, the compiler will generate a warning
}
7..warning: #1295-D: Deprecated declaration lcd_init - give arg types
Description: When defining a function, if you write function parameters, this warning will appear, such as void timer_init(); there is no formal parameter here, if this happens, the compiler will give a warning.
1. error: #65: expected a ";"
Description: Missing semicolon. Most of the time, it is because the ';' is forgotten.
Solution: Double-click the error line, find the statement without the ';' sign near the error point, and add a semicolon. The semicolon is not necessarily missing in the error line, it may be the previous line or the next line.
2. error: #65: expected a ";" and error: #20: identifier "xxxx" is undefined appear together, and the following error: #20 may have a lot of
descriptions: This error is definitely a nightmare for those who encounter it for the first time. When the error appears, you double-click the error prompt with hope, but when you come to the error line, you are shocked to find that the error line is absolutely correct. So you look for the previous line and the next line of the error line, and there is no error. Then you look for the previous line and the next line... Something very depressing has happened: all the error lines in the compilation prompt cannot have errors. In fact, this is most likely because you did not add a semicolon at the end of the declaration statement when declaring external variables or functions in the .h file! If you have many modules, such as main.c, lcd.c, key.c... and many header files, such as lcd.h, key.h, if you do not add a semicolon when declaring a function in the lcd.h file, then this error may be determined in main.c, so you need to check all header files.
Solution: Check the .h file carefully and add the semicolon.
3. Error: L6200E: Symbol flagu multiply defined (by uart0.o and main.o).
Description: The variable (also a symbol) flagu is defined in multiple places (in uart0.c and main.c). This is usually caused by repeated global variable definitions. For example, in main.c, define the global variable flagu:
uint8 flag=0;
This variable is also used in uart0.c, so to declare this variable, I usually copy the defined variable first and then add the keyword extern in front of the variable:
extern uint8 flagu=0;
Then compile, the above connection error will appear. The reason is that I defined another variable in uart0.c instead of declaring the variable, because I assigned the initial value "flagu=0" to the variable, which repeated the definition of the variable flag. The correct declaration method is to remove the assignment part:
extern uint8 flagu;
Solution: Find the duplicate defined variables and modify one of them depending on the situation.
4.error: #159: declaration is incompatible with previous "wr_lcd" (declared at line 40)
Description: The wr_lcd function was used before it was declared. This often occurs in two situations: First, the wr_lcd function body has not been written yet, but it has been used. This often occurs when writing the general structure of a program, where just a simple framework is written. The second situation is more common, where function a calls function b, but the body of function b is below function a:
void a(void) //the entity of function a
{
b(); //call function b
}
void b(void) //Entity of function b
{
...
}
If you compile this, error #159 will be generated, because when function a calls function b, it is found that there is no declaration of function b before it.
Solution: Before function a calls function b, declare function b, such as:
void b(void); //Declare function b
void a(void) //Entity of function a
{
b(); //Call function b
}
void b(void) //Entity of function b
{
...
}
5. error: #137: expression must be a modifiable lvalue
Description: The expression must be a modifiable left value. It mainly appears in this phenomenon:
a=NUM;
NUM is a value or expression, and a is a variable, but a is defined as an immutable type like const, which means that NUM cannot be assigned to variable a.
Solution: Either give up the assignment or modify the variable properties.
6.error: #18: expected a ")"
If it appears in a c file, it is probably because a ")" is missing, or the error line contains characters that the compiler does not recognize.
If it appears in a header file, and the error line is a function declaration, it is probably because there are characters in the function declaration that the compiler does not recognize.
7.error: #7: unrecognized token
Unrecognized marks are mostly switched to Chinese punctuation.
Previous article:STM32 uses SWD to connect and report errors
Next article:Macro definition problem of STM32
Recommended ReadingLatest update time:2024-11-16 11:40
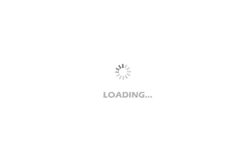
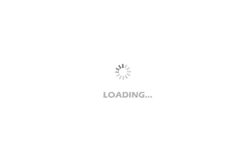
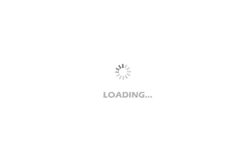
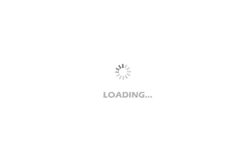
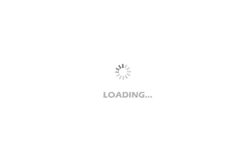
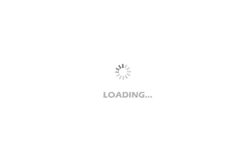
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- [Atria AT32WB415 Series Bluetooth BLE 5.0 MCU] WDT Watchdog Analysis and Application
- C program about low power consumption msp430PID control motor speed
- My Journey of MCU Development (Part 3)
- Design of Programmable SOC System Based on AXI4
- Universal control board based on 3U PXIe ZU11EG
- No more looking down! ADAS gives rise to head-up display technology
- 11 Questions That Can Commonly Stuck in Hardware Engineer Interviews
- About the startup problem of ZVS high voltage resonant circuit
- Data acquisition based on LIS25BA bone vibration sensor of NUCLEO-767ZI
- BLE RF Related FAQs