General register access needs to be implemented through the three steps of read-modify-write and bit operations of clearing and setting. However, in the programming of STM32, by utilizing some of its excellent features such as port bit set/reset register BSRR, bit binding, etc., we can greatly improve the access speed of registers and simplify the operation of registers.
//General register operations:
GPIOx->ODR |= 0x10; //Pin4 set to 1
GPIOx->ODR &= ~0x10; //Pin4 cleared to 0
BSRR/BRR Register
GPIOx->BSRR //Write 1 to set the lower 16 bits of BSRR, and write 1 to clear the upper 16 bits of BSRR
GPIOx->BRR //Write 1 to clear the lower 16 bits of BRR, and keep the upper 16 bits of BRR
It can be seen that operating the ODR register through the BSRR/BRR register does not require the three steps of read-modify-write. It can be done in one step by just writing, which is much more convenient.
Bit Binding
Of course, stm32 has an even more powerful feature - bit binding, which can realize single bit operation in just one clock cycle. Bit binding is to map a bit in a register to a storage unit in memory through a simple address conversion. In this way, a bit of the corresponding register can be indirectly accessed by reading and writing a memory unit. Of course, at this time, only the lowest bit of the 32-bit memory unit is valid!
However, the entire M3 core does not allow bit binding, only two areas do, namely
SARM: 0x20000000~0x2000FFFF 1M size
The address bound to this area starts from 0x22000000;
PERIPHERALS: 0x40000000~0x4000FFFFF 1M size
The address bound to this area starts from 0x42000000;
The corresponding bit binding formula is:
SRAM:AliasADDr = 0x22000000+((A-0x20000000)*32+n*4)
Where A: 0x20000000~0x2000FFFF n: 0~31
PERIPHERALS: AliasADDr = 0x42000000+((A-0x40000000)*32+n*4)
Where A: 0x40000000~0x4000FFFFF n: 0~31
Now we can quickly implement bit operations through bit binding
#define GPIOA_ODR_ADDR (GPIOA_BASE + 0x0C)
#define GPIOA_IDR_ADDR (GPIOA_BASE + 0x08)
#define BitBind(Addr, bitNum) (*(volatile unsigned long *)((Addr&0xF0000000)+0x2000000+((Addr&0xFFFFF)<<5)+(bitNum<<2)))
//Addr&0xF0000000 is to distinguish SRAM or PERIPHERALS
//Addr&0xFFFFF is equivalent to (A-0x20000000) or (A-0x40000000)
//Left shift is to achieve fast multiplication operation: left shift n bits is equivalent to multiplying by 2^n
#define PAout(n) BitBind(GPIOA_ODR_ADDR, n)
#define PAin(n) BitBind(GPIOA_IDR_ADDR, n)
This implements an operation similar to the 51 microcontroller access to I/O
sbit P10 = P1^0
P10 = 0; or P10 = 1;
PAout(3) = 1; or PAout(3) = 0;
Pretty cool, huh!
Previous article:Comparison of library functions and register operations under STM32
Next article:Some good programming ideas, methods and good programs in the STM32 library
Recommended ReadingLatest update time:2024-11-16 19:48
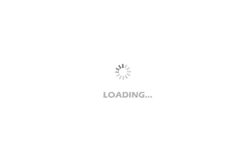
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- mcgs Kunlun Tongtai Modbus RTU, Modbus TCP communication method Modicon Modbus communication configuration steps
- Proficient in hardware and familiar with software
- [Nucleo G071 Review] SYSTICK & Comparison of Two Commonly Used Low Power Modes
- [Top Micro Smart Display Screen Review] 3. Run SciTE Lua script editor to test the screen alarm function
- Material problem of wifi gun
- The processing of instantaneous signals, the signal is -50mV-0V, the time length is 16us, such instantaneous signals need to be extracted and processed...
- Can adding a resistor in parallel to the load effectively prevent voltage fluctuations?
- 【TGF4042 signal generator】+Sweep application in issue 7
- Why does the microcontroller stop working after the DIP switch circuit is powered on?
- Download and receive gifts | The era of artificial intelligence and the Internet of Things is coming, are you ready?