Use the configured GPIO of stm32 to control the LED display status, and use ODR, BSRR, and BRR to directly control the pin output status.
The ODR register is readable and writable: it can control the pin to a high level or a low level.
For the pin, writing 1 to the gpio pin is high level, and writing 0 is low level.
BSRR Write-only register: [color=Red] can control the pin to be high or low.
Writing 1 to the upper 16 bits of the register will cause the corresponding pin to be low, and writing 1 to the lower 16 bits of the register will cause the corresponding pin to be high. Writing 0, no action
BRR Write-Only Register: It can only change the pin status to low level. If you write 1 to the corresponding bit of the register pin, the corresponding pin will be low level. If you write 0, there will be no action.
At the beginning, you may have the following doubts like me:
1. Since ODR can control the high and low levels of the pin, why do we need BSRR and SRR registers?
2. Since BSRR can achieve all the functions of BRR, why do we need SRR registers?
For question 1 ------ STMicroelectronics' answer is ---
“This way, there is no risk that an IRQ occurs between the read and the modify access.”
What does this mean? It means that when you use BSRR and BRR to change the pin state, there is no risk of being interrupted by an interrupt. There is no need to disable interrupts.
The pseudo code for operating GPIO with ODR is as follows:
disable_irq()
save_gpio_pin_sate = read_gpio_pin_state();
save_gpio_pin_sate = xxxx;
chang_gpio_pin_state(save_gpio_pin_sate);
enable_irq();
Disabling interrupts will obviously delay or lose the capture of an event, so it is best to use SBRR and BRR to control the state of GPIO.
Regarding question 2 ------- Based on my personal experience, STMicroelectronics probably did this just to make it easier for programmers to operate.
Because the lower 16 bits of BSRR happen to be the set operation, while the upper 16 bits are the reset operation and the lower 16 bits of BRR are the reset operation.
Simply put, the upper 16 bits of GPIOx_BSRR are called the clear register, and the lower 16 bits of GPIOx_BSRR are called the set register.
Another register GPIOx_BRR has only the lower 16 bits valid and has the same function as the upper 16 bits of GPIOx_BSRR.
Here is an example to illustrate how to use these two registers and the advantages they provide.
For example, the 16 IOs of GPIOE are all set as outputs, and each operation only requires
Change the lower 8 bits of data while keeping the upper 8 bits unchanged. Assuming the new 8 bits of data are in the variable Newdata,
This requirement can be achieved by operating these two registers. There are two functions in the STM32 firmware library
GPIO_SetBits() and GPIO_ResetBits() use these two registers to operate the port.
The above requirements can be achieved as follows:
GPIO_SetBits(GPIOE, Newdata & 0xff);
GPIO_ResetBits(GPIOE, (~Newdata & 0xff));
You can also directly operate these two registers:
GPIOE->BSRR = Newdata & 0xff;
GPIOE->BRR = ~Newdata & 0xff;
Of course, you can also complete the operation of 8 bits at a time:
GPIOE->BSRR = (Newdata & 0xff) | ( (~Newdata & 0xff)<<16 );
Of course, you can also complete the operation of 16 bits at a time:
GPIOE->BSRR = (Newdata & 0xffff) | ( (~Newdata )<<16 );
From the last operation, you can see that using the BSRR register, you can implement the simultaneous modification of 8 port bits.
Some people ask whether the upper 16 bits of BSRR are redundant. Please see the following example:
If you want to set bit 7 of GPIOE to '1' and bit 6 to '0' in one operation, it is very convenient to use BSRR:
GPIOE->BSRR = 0x400080;
If there is no upper 16 bits of BSRR, the operation will be divided into two times, resulting in the asynchronous changes of bit 7 and bit 6!
GPIOE->BSRR = 0x80;
GPIOE->BRR = 0x40;
Another feature of BSRR is that the level of Set is higher than that of Reset.
That is to say, the same bit is set and reset, and the final result is Set
To synchronize the changes just simply GPIOx->BSRR = 0xFFFF0000 | PATTEN;
No need to consider which ones need to be set to 1 and which ones need to be cleared to 0.
From the last operation, we can see that using the BSRR register, the 8 port bits can be modified simultaneously.
Previous article:About BSRR and BRR registers of STM32_GPIO
Next article:STM32 study notes - GPIO from library functions to registers
Recommended ReadingLatest update time:2024-11-16 16:17
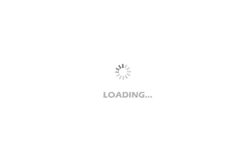
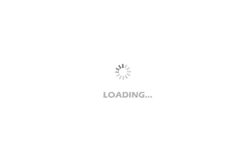
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Initialization GPIO steps of TMS320C6748
- The microchip Harmony interface is different from the tutorial
- Automotive Ethernet (2nd) from BMW Kirsten Matheus
- Regarding 24V/1.5A power input, what kind of interface should be used on the product?
- FAQ_How to convert Tmall Genie triples to the format used by ST mesh code
- EEWORLD University Hall----What can be easily charged?
- Share the steps of DSP development
- The long-awaited Digi-Key STM32F7508-DK and MAiX Bit unboxing notes
- It is customary to connect a 120Ω resistor between RS485 interface AB and CAN interface H and L. I only have 100Ω in stock. Can it be replaced?
- [NXP Rapid IoT Review] Online IDE Experience