When learning about various hardware, the serial port is an essential and first peripheral to learn, because with the serial port, you can interact with the hardware in a simple way, and it is also easier to verify the correctness of the written program.
STM32 has multiple USARTs and supports multiple modes of configuration. For details, please refer to the relevant manual of STM32 for learning.
Let's take a look at the functional block diagram of USART:
Before using any peripheral function, you should look at its corresponding functional block diagram, which will help you understand the use of the function.
Next, take a look at the relevant registers and operable library functions of USART.
Finally, we need to look at the pin multiplexing of GPIO and USART:
Because USART communicates with the outside world using the multiplexing function of the controller's GPIO pins, you need to configure GPIO to the corresponding input and output mode before using the USART function.
At the same time, if you need to enable interrupts, you also need to enable the interrupt channel and set the priority grouping.
#include "stm32f10x.h"
/**
* @brief Initialize GPIO, the default speed is GPIO_Speed_50MHz;
*
* @param GPIO group
* @param GPIO pin
* @param GPIO pin mode
* @retval None
*/
void GPIO_init(GPIO_TypeDef * GPIOx,u16 GPIO_Pin,GPIOMode_TypeDef Mode)
{
GPIO_InitTypeDef GPIO_InitStruct;
GPIO_InitStruct.GPIO_Mode=Mode;
GPIO_InitStruct.GPIO_Pin=GPIO_Pin;
GPIO_InitStruct.GPIO_Speed=GPIO_Speed_50MHz;
GPIO_Init(GPIOx,&GPIO_InitStruct);
}
/**
* @brief Initialize USART
*
* @param baud rate, number of data bits, stop bits, send/receive mode
* @retval None
*/
void UART_init(int BaudRate,u16 WordLength,u16 StopBits,u16 Parity,u16 Mode)
{
USART_InitTypeDef USART_InitStruct;
USART_InitStruct.USART_BaudRate=BaudRate;
USART_InitStruct.USART_HardwareFlowControl=USART_HardwareFlowControl_None;
USART_InitStruct.USART_Mode=Mode;
USART_InitStruct.USART_Parity=Parity;
USART_InitStruct.USART_StopBits=StopBits;
USART_InitStruct.USART_WordLength=WordLength;
USART_Init(USART1,&USART_InitStruct);
USART_ITConfig(USART1,USART_IT_RXNE,ENABLE);
USART_Cmd(USART1,ENABLE);
}
/**
* @brief Initialize interrupt vector
*
* @param interrupt channel, preemption priority, corresponding priority, enable/disable
* @retval None
*/
void NVIC_init(u8 NVIC_IRQChannel,u8 NVIC_IRQChannelPreemptionPriority,u8 NVIC_IRQChannelSubPriority,u8 NVIC_IRQChannelCmd)
{
NVIC_InitTypeDef NVIC_InitStrue;
NVIC_InitStrue.NVIC_IRQChannel=NVIC_IRQChannel;
NVIC_InitStrue.NVIC_IRQChannelCmd=NVIC_IRQChannelCmd;
NVIC_InitStrue.NVIC_IRQChannelPreemptionPriority=NVIC_IRQChannelPreemptionPriority;
NVIC_InitStrue.NVIC_IRQChannelSubPriority=NVIC_IRQChannelSubPriority;
NVIC_Init(&NVIC_InitStrue);
}
int main()
{
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA,ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1,ENABLE);
NVIC_PriorityGroupConfig(NVIC_PriorityGroup_2);
GPIO_init(GPIOA,GPIO_Pin_9,GPIO_Mode_AF_PP);
GPIO_init(GPIOA,GPIO_Pin_10,GPIO_Mode_IN_FLOATING);
NVIC_init(USART1_IRQn,1,1,ENABLE);
GPIO_init(GPIOA,GPIO_Pin_0,GPIO_Mode_Out_PP);
GPIO_ResetBits(GPIOA,GPIO_Pin_0);
UART_init(115200,USART_WordLength_8b,USART_StopBits_1,USART_Parity_No,USART_Mode_Tx|USART_Mode_Rx);
while(1);
}
void USART1_IRQHandler(void)
{
u8 res;
if(USART_GetITStatus(USART1,USART_IT_RXNE))
{
res=USART_ReceiveData(USART1);
USART_SendData(USART1,res);
}
}
The last function is the response function of the serial port receive interrupt. When the serial port receives data and the response flag is set to 1, it enters the interrupt function, provided that the serial port receive interrupt is enabled.
The above program realizes the reception and transmission of the serial port, sending and receiving the incoming data.
Previous article:STM32 - printf redirection to USART
Next article:STM32-light up the LED (GPIO configuration)
Recommended ReadingLatest update time:2024-11-16 15:36
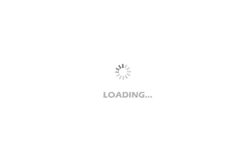
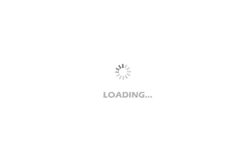
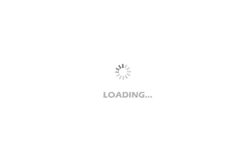
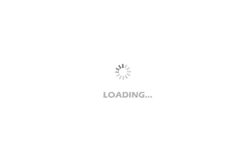
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Msp430 contains ADC12 module
- A wrong partner will bury your genius ideas
- Automatic air conditioning comes in
- Solution to CCS8.1.0 not finding .h files
- EEWorld invites you to attend the 2019 STM32 Summit and Fan Carnival!
- Ask a question about the LM35CZ temperature sensor
- Functions of vent valve and drain valve of magnetic flap level gauge
- FatFs transplantation based on SPI for MSP430F5438A microcontroller
- Problem with STM32F767 timer overflow interrupt flag
- Comparison of the advantages of digital cameras and analog cameras in machine vision system design