1. Introduction to timers
51 MCU has two 16-bit timers/counters T0 and T1, 52 MCU has three 16-bit timers/counters T0, T1, T2.
As the name implies, timers and counters have the functions of timing and counting. The following mainly introduces timers, and does not discuss counters. The timing function of MCU is widely used in daily MCU programming. At the same time, timing interrupt is also one of the commonly used interrupts of MCU, so it is also very important to learn timing control. If you do not understand the concept of interrupt, please check my article Understanding of Embedded Interrupts http://blog.csdn.net/snyanglq/article/details/50238205
2. Analysis of the internal block diagram of the microcontroller and the working principle of the timer
Please look at the figure above, which is the internal structure of timer T0 and T1. The working relationship between them is marked with lines of different colors.
From the red line in the figure above, we can see that TMOD selects which timer to work and in what mode;
from the blue line in the figure above, we can see that TCON determines whether the timer is started;
from the yellow line in the figure above, we can see that the external technical input is accumulated by TH and TL;
from the purple line in the figure above, we can see that when TH and TL counts overflow, they will apply for a report to TCON;
from the green line in the figure above, we can see that all timer interrupts are applied to the CPU by TCON;
from the black line in the figure above, we can see that the external interrupt directly applies to the CPU;
through the above figure, we have a clear understanding of the internal structure of the CPU's timer interrupt, and now let's take a look at the working principle diagram of the microcontroller.
From the above figure, we can see When used as a timer, the timing pulse comes from the 12-frequency division of the external crystal oscillator frequency. When set as a counter, the external pulse is directly used as the timing pulse. The control of timing or counting is controlled by the C/T bit, and whether the timer is timing is controlled by B. B is the output of an AND gate. The working principle of the AND gate is that 0 outputs 0 and all 1s output 1. It can be seen that TRx and A must be 1 to start the timer, and A is the output of the OR gate. The working principle of the OR gate is that 1 outputs 1 and all 0s output 0, so any bit of the input terminal must be 1. When all conditions are met, TH and TL start timing calculation. When the two counters overflow, TF will be set. Finally, TF will send an interrupt request to the CPU to process the interrupt program. Note that at this time, the counter of the microcontroller will be cleared. This is determined by the internal design of the counter, so the initial value of the counter needs to be reloaded when the timing needs to be used again.
3. Register Introduction
TCON:
TF0 and TF1: timer/counter overflow flag.
When timer/counter 0 (or timer/counter 1) overflows, the hardware automatically sets TF0 (or TF1) to 1 and requests an interrupt to the CPU.
After the CPU responds to an interrupt, TF1 is automatically cleared. TF1 can also be cleared by software.
TR0 and TR1: Timer/Counter start and run control bits.
TR0 (or TR1) = 0, stop Timer/Counter 0 (or Timer/Counter 1).
TR0 (or TR1) = 1, start Timer/Counter 0 (or Timer/Counter 1).
External interrupts are not introduced yet.
TMOD:
GATE: Gate bit.
GATE=0, as long as TR0 (or TR1) is set to 1 by software, timer/counter 0 (or timer/counter 1) can be started;
GATE=1, only when the (or) pin is high level and TR0 (or TR1) is set to 1 by software, timer/counter 0 (or timer/counter 1) can be started. No matter what state GATE is in, as long as TR0 (or TR1)=0, the timer/counter will stop working.
C/T: Timer/counter working mode selection bit.
C/T=0, timing working mode;
C/T=1, counting working mode.
M0, M1: working mode selection bit, determine 4 working modes.
IE:
EA = 1, CPU opens total interrupt;
ET0 = 1, T0 interrupt is allowed;
TR TL: Timer register
Note: Since the reg52.h library defines most of the registers, you can directly assign values to TCON TMOD IE or operate on individual registers.
4. Interrupt program execution method and timing counting formula
There are two methods for interrupt program execution: direct interrupt and query. Query means to query whether the TF bit is set to 1.
The calculation formula for the timing initial value is:
Timing time = (maximum count value - initial count value) × machine cycle
Machine cycle T = 12 / crystal frequency
or modulo
TH0 = (maximum count value - timing time) / 256;
TL0 = (maximum count value - timing time) % 256;
First of all, why do we need to set the initial value?
When the timer is set to a 16-bit counter, the maximum count value is 2^16 = 65536;
TH and TL are 8 bits respectively, that is, TL advances by one bit every 256, so dividing 256 can show how many bits TH has advanced, and modulo is how many bits are left.
For example, the maximum count of a 2-bit counter is 4. To divide the initial value 6 into two 2-bit counters, since the low bit advances by 1 every four bits, there are 2 bits left.
That is, TL = 2 and the high bit is 1, that is, TH = 1. According to the above method: 2^4 = 16, 2^2 = 4; TH = (16-(16-6))/4=1 (integer does not count decimals);
TL = (16-(16-6))%4=2; it can be seen that the results are the same.
5. Programming
example: Use digital tube to display 0 to 9 statically, add one every 1S, add 1 to 9 and return to 0, use interrupt program to realize.
Analysis: Adding one every second means that it takes 1S to start the action. Since the maximum timing of the single-chip microcomputer is only 65536ms,
So we need to accumulate. For the convenience of calculation, we accumulate 20 times of 50ms.
#include
#define uchar unsigned char
#define uint unsigned int
uchar code smg_du[]={0x3f,0x06,0x5b,0x4f,0x66,0x6d,0x7d,0x07,0x7f,0x6f,0x77,0x7c,0x39,0x5e,0x79,0x71,0x00};
uchar num,a;
void main()
{
TMOD=0x01; //Set timer 0 to work mode 1 [direct assignment]
TH0=(65536-50000)/256; //Use the modulo method to set the initial value
TL0=(65536-50000)%256;
EA=1; //Turn on the general interrupt switch [directly operate the register]
ET0=1; //Enable timer interrupt
a = 0; // Initialize a and num. You can also remove them for program rigor.
num = 0;
TR0=1; //Start the timer
while(1)
{
if(a==20)
{
a=0;
P1=smg_du[num];
num++;
if(num==10)
{
num=0;
}
}
}
}
void timer0 () interrupt 1
{
TH0=(65536-50000)/256; //Reload the initial value, otherwise the next timing will be 65535us instead of 50ms
TL0=(65536-50000)%256;
a++; //50ms interrupt accumulation
}
Previous article:MCU controls serial communication
Next article:Single chip microcomputer control independent key reading
Recommended ReadingLatest update time:2024-11-16 21:33
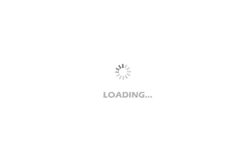
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Is there such a pressure sensor?
- Thank you for being there + thank you for persisting
- Embedded OTA air download technology
- How to improve EMI of circuit boards by placing components?
- Altera IP Core User Manual
- How to use xilinx primitives.pdf
- All useful information! Detailed explanation of the 51 single-chip microcomputer minimum system
- 6-pin power chip personal summary information
- BQ21040 single-cell lithium battery
- 【RPi PICO】Use SPI to drive WS2812