*************************************************** *************************************************** *************************************
Development board: STM32
CPU: STM32F103
Development environment: keil uVsion4
*************************************************** *************************************************** ************************************
Preface: This article mainly uses key interrupts to achieve that when the key is pressed, the LED light goes off, and when the key is released, the LED light turns on. (However, I set the initial state of the LED to be on, that is, the LED light turns on when the power is turned on.)
The schematic diagram of the GPIO pins corresponding to the buttons and LEDs on my development board is as follows:
The function of the key interrupt program I wrote is: when the key K2 is pressed, LED3 turns from bright to dark, and when the key is released, LED3 turns bright again. (Key K2 corresponds to the PC2 pin, and LED3 corresponds to the PD3 pin).
Test code:
#include "misc.h"
#include "stm32f10x_gpio.h"
#include "stm32f10x_exti.h"
#include "stm32f10x_rcc.h"
#define LED_ON GPIO_SetBits(GPIOD, GPIO_Pin_3);
//unsigned char flag=0;
//unsigned char num=0;
void LED_Config(void);
void EXTI_PC2_Config(void);
void RCC_Config(void);
//void KEY_Dither(void);
//void delay_nms(u16 time);
int main(void)
{
// unsigned char a = 0;
RCC_Config();
LED_Config();
EXTI_PC2_Config();
LED_ON;
while(1)
{
//KEY_Dither();
//if(num==1&&a==0){GPIO_ResetBits(GPIOD,GPIO_Pin_6);a=1;}
//else if(num==1&&a==1){GPIO_SetBits(GPIOD,GPIO_Pin_6);a=0;}
}
}
/*void KEY_Dither(void)
{
num=0;
if(flag==1)
{
delay_nms(12000);
if(GPIO_ReadInputDataBit(GPIOD,GPIO_Pin_6)==0)
{
delay_nms(12000);
if(GPIO_ReadInputDataBit(GPIOD,GPIO_Pin_6)==0)
{
while(GPIO_ReadInputDataBit(GPIOD,GPIO_Pin_6)==0)
num=1;
goto n_exit;
}
}
else ;
n_exit:;
flag=0;
}
}
void delay_nms(u16 time)
{
u16 i=0;
while(time--)
{
i=12000; //self-defined
while(i--) ;
}
}
*/
void RCC_Config(void)
{
RCC_APB2PeriphClockCmd(RCC_APB2Periph_AFIO, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB |RCC_APB2Periph_GPIOD,ENABLE);
SystemInit();
}
void LED_Config(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
/*led config*/
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_3;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOD, &GPIO_InitStructure);
}
void EXTI_PC2_Config(void)
{
NVIC_InitTypeDef NVIC_InitStructure;
EXTI_InitTypeDef EXTI_InitStructure;
GPIO_InitTypeDef GPIO_InitStructure;
/*config for NVIC*/
NVIC_PriorityGroupConfig(NVIC_PriorityGroup_1);
NVIC_InitStructure.NVIC_IRQChannel = EXTI2_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
/* key for exti */
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_2;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(GPIOC, &GPIO_InitStructure);
GPIO_EXTILineConfig(GPIO_PortSourceGPIOC, GPIO_PinSource2);
/*EXIT line(PC2) mode config */
EXTI_InitStructure.EXTI_Line = EXTI_Line2;
EXTI_InitStructure.EXTI_Mode = EXTI_Mode_Interrupt;
EXTI_InitStructure.EXTI_Trigger = EXTI_Trigger_Falling;
EXTI_InitStructure.EXTI_LineCmd = ENABLE;
EXTI_Init(&EXTI_InitStructure);
}
void EXTI2_IRQHandler(void)
{
if (EXTI_GetITStatus(EXTI_Line2) != RESET)
{
// flag = 1; // button pressed flag
/*LED inversion*/
GPIO_WriteBit(GPIOD, GPIO_Pin_3,
(BitAction)((1-GPIO_ReadOutputDataBit(GPIOD, GPIO_Pin_3))));
EXTI_ClearITPendingBit(EXTI_Line2);
}
}
*************************************************** *************************************************** *********************************
Note: This program I wrote only realizes the simple function of turning the light from on to off when the button is pressed. However, the test found that the button jitters too much. When the button is touched lightly, the light will flash. Sometimes
When I touch the button, the LED goes out before I press it. So I use the delay_nms() delay function to debounce. The commented-out code in the code is for debounce. However, the debounce effect is not very good. There is still jitter, but it is better than before! However, the official has provided a delay function, which can be called by the official delay function. Generally, 10ms can be used for debounce.
*************************************************** *************************************************** *********************************************
The main purpose of writing this program is to complete the corresponding configuration work. The steps are as follows:
(1) Initialize the system clock and
Initialize external clock (clock configuration)
void RCC_Config(void)
{
RCC_APB2PeriphClockCmd(RCC_APB2Periph_AFIO, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB |RCC_APB2Periph_GPIOD,ENABLE);
SystemInit(); //System clock initialization
}
*************************************************** *************************************************** *************************************************** ***********************
Note: We don't need to add SystemInit(); it doesn't matter if we don't add this function, because the startup_stm32f10x_hd.s file has already done these things for us.
*************************************************** *************************************************** *************************************************** ***********************
(3) Configure LED
void LED_Config(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
/*led config*/
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_3;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP; //Push-pull output
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOD, &GPIO_InitStructure);
}
(4) Configure interrupt priority
NVIC_PriorityGroupConfig(NVIC_PriorityGroup_1);
NVIC_InitStructure.NVIC_IRQChannel = EXTI2_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
*************************************************** *************************************************** *************************************************** ***************************
Note: The interrupt priority setting can be set arbitrarily. The reason is that the project I created only uses the key interrupt, and there are no other interrupts. Of course, if there are multiple interrupts, you have to follow the interrupt vector table.
And the interrupt priority setting rules are set. For specific rules, you can refer to the information on the Internet. There are many, you can take a look, I will not explain it here. Here I set the first group of interrupts, the preemption priority is
0, the response priority is also 0.
*************************************************** *************************************************** *************************************************** ****************************
(5) Configure external interrupt line
/*EXIT line(PC2) mode config */
EXTI_InitStructure.EXTI_Line = EXTI_Line2;
EXTI_InitStructure.EXTI_Mode = EXTI_Mode_Interrupt; //Interrupt mode
EXTI_InitStructure.EXTI_Trigger = EXTI_Trigger_Falling; //Falling edge trigger
EXTI_InitStructure.EXTI_LineCmd = ENABLE;
EXTI_Init(&EXTI_InitStructure);
*************************************************** *************************************************** *************************************************** *******************************
Note: There are two interrupt modes: Event and Interrupt. Since this is an interrupt, we set it to
Interrupt, because it is Key2, the interrupt line here is EXTI_Line2. If the key is 5-9, it should be written as EXTI9_5_IRQn.
*************************************************** *************************************************** *************************************************** *********************************
(6) Interrupt handling function
void EXTI2_IRQHandler(void)
{
if (EXTI_GetITStatus(EXTI_Line2) != RESET) //Judge whether the button is pressed
{
/*LED inversion*/
GPIO_WriteBit(GPIOD, GPIO_Pin_6,
(BitAction)((1-GPIO_ReadOutputDataBit(GPIOD, GPIO_Pin_6))));
EXTI_ClearITPendingBit(EXTI_Line2); //Clear interrupt flag
}
}
*************************************************** *************************************************** *************************************************** **********************************
Note: It is usually best to put the interrupt handling function here in the file stm32f10x_it.c. This is an official file used to store all external interrupt handling functions. When the main program is executing the program, if an interrupt occurs, the cpu will immediately jump here to execute the interrupt handling function. Because there is only a key interrupt here, the benefits of doing so are not reflected. If it is a large project and multiple interrupts are used, putting the interrupt functions in this file can greatly optimize the code.
*************************************************** *************************************************** *************************************************** *********************************
Previous article:STM32 actual combat button lights up LED (interrupt)
Next article:STM32 (II) GPIO Operation (2) - Control the switch of LED light by button
Recommended ReadingLatest update time:2024-11-17 00:04
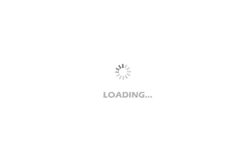
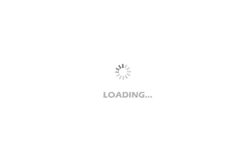
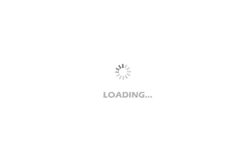
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- EEWORLD University ---- Basic knowledge of TID
- Design of filter circuit
- How to use a touch switch to make a circuit that turns on when pressed once and turns off when pressed again
- Meng Wanzhou is about to return to China
- Equivalent circuits of components such as resistors, capacitors, inductors, and thyristors
- Want to learn power supply design
- SI4463 wireless wake-up principle
- [Prize Download] Infineon "Selection Guide for Power Devices for Fashionable Small Home Appliances"
- [Rawpixel RVB2601 development board trial experience] 2. Temperature and humidity sensor DHT11 test, serial port print results
- After drawing the PCB with Kicad, the following error occurs during the rule check: the pads are too close to each other. How can I solve this problem?