Let's take a look at the simplest experiment of lighting a lamp. The experiment requires you to use KEY4*4 buttons. Press the button to turn on the light, and lift the button to turn off the light, and repeat this cycle:
The idea of the program is as follows:
1. The first thing you need to set is the clock: you turn on the clock of the port to which you connect the pin of the lamp. For example, if the lamp is connected to a pin of port A, you need to turn on the clock of port A.
2. Secondly, set the pins (we need to set the pins of the LED and a key in the matrix keyboard): We need to initialize the GPIO. We only need to call the GPIO_InitTypeDef structure and set each member. We need to set the position of the PIN pin GPIO_Pin, the speed of the pin GPIO_Speed, and the working state of the pin GPIO_Mode. Finally, call the initialization function GPIO_Init.
3. Then start writing our main function: If I want to set the pin of the LED lamp to a high level, I only need to call the GPIO_SetBits function, which sets the pin to a high level. If I want to set it to a low level, I just call the GPIO_ResetBits function.
4. Finally, we need to make it light up when pressed and go out when lifted, so we need an if statement
——————————————————————————————————————————————————-
Below is the program: The chip used is STM32F103C8T6, the LED control pin is C13, and the matrix keyboard is B9
The first step is to control the small light to be a push-pull output
void LED_Init (void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOC,ENABLE); //Initialize GPIOC clock
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_13;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(GPIOC, &GPIO_InitStructure);
}
Then configure the matrix keyboard
void KEY_Init (void)
{
GPIO_InitTypeDef GPIO_InitStructure; //Configure PB9 pull-up input
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB,ENABLE); //Initialize GPIOB clock
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(GPIOB, &GPIO_InitStructure);
}
Next is the main function
int main()
{
LED_Heat();
KEY_Init();
while(1)
{
LED = 1;
if(KEY == 0)
{
LED = 0;
}
}
}
Previous article:STM32 learning notes: key query method to control the on and off of LED lights
Next article:STM32_button interrupt
Recommended ReadingLatest update time:2024-11-16 22:40
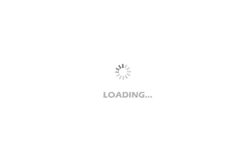
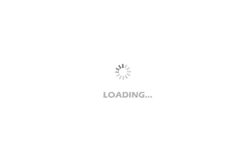
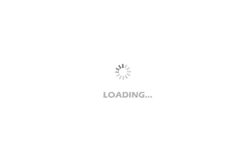
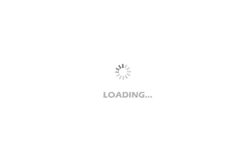
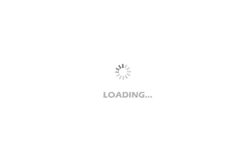
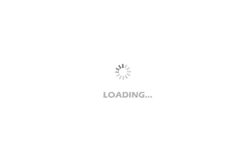
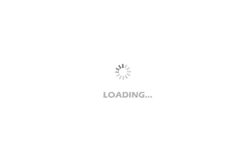
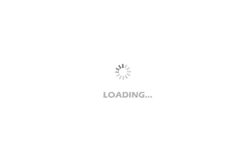
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- FAQ_How to fill in the BQB certification application form
- [2022 Digi-Key Innovation Design Competition] Material Unboxing Raspberry Pi 400
- HC6800 EM3 V2.0 CD-ROM
- The gas discharge tube keeps glowing. Is it broken?
- How to test the withstand voltage characteristics of general ceramic capacitors
- EEWORLD University Hall ---- CMOS Analog Integrated Circuit Design Wu Jin, Southeast University
- What is the function of decoupling capacitor in microcontroller?
- [Shanghai Hangxin ACM32F070 development board + touch function evaluation board]——GPIO routine (edited with Sphinx document)
- Classic time series
- TI - Delivering Ultra-High Power Density for 100W USB Power Delivery Adapters