The application of learning serial communication is mainly to realize the exchange of information between the microcontroller and the computer. The computer can control some information of the microcontroller, and some information status of the microcontroller can be sent to the software on the computer. The following is a simple routine to realize the data sent by the microcontroller serial port debugging assistant and display it on the digital tube on the development board.
#include
sbit ADDR3 = P1^3; //LED select address line 3
sbit ENLED = P1^4; //LED total enable pin
unsigned char code LedChar[] = { // Digital tube display character conversion table
0xC0, 0xF9, 0xA4, 0xB0, 0x99, 0x92, 0x82, 0xF8,
0x80, 0x90, 0x88, 0x83, 0xC6, 0xA1, 0x86, 0x8E
};
unsigned char LedBuff[6] = { //digital tube
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF
};
unsigned char T0RH = 0; //high byte of T0 reload value
unsigned char T0RL = 0; //Low byte of T0 reload value
unsigned char RxdByte = 0; //Byte received by the serial port
void ConfigTimer0(unsigned int ms);
void ConfigUART(unsigned int baud);
void main ()
{
P0 = 0xFF; //P0 port initialization
ADDR3 = 1; //Select digital tube
ENLED = 0; //LED is always enabled
EA = 1; // Enable general interrupt
ConfigTimer0(1); //Configure T0 timing 1ms
ConfigUART(9600); //Configure the baud rate to 9600
while(1)
{ //Display the received byte in hexadecimal format on the digital tube
LedBuff[0] = LedChar[RxdByte & 0x0F];
LedBuff[1] = LedChar[RxdByte >> 4];
}
}
void ConfigTimer0(unsigned int ms) //T0 configuration function
{
unsigned long tmp;
tmp = 11059200 / 12; //Timer counting frequency
tmp = (tmp * ms) / 1000; //Calculate the required count value
tmp = 65536 - tmp; //Calculate the timer reload value
tmp = tmp + 31; //Correct the error caused by interrupt response delay
T0RH = (unsigned char)(tmp >> 8); //Timer reload value is split into high and low bytes
T0RL = (unsigned char)tmp;
TMOD &= 0xF0; // Clear the control bit of T0
TMOD |= 0x01; //Configure T0 to mode 1
TH0 = T0RH; //Load T0 reload value
TL0 = T0RL;
ET0 = 1; // Enable T0 interrupt
TR0 = 1; //Start T0
}
void ConfigUART(unsigned int baud) //Serial port configuration function, baud is the baud rate
{
SCON = 0x50; //Configure the serial port to mode 1
TMOD &= 0x0F; // Clear the control bit of T1
TMOD |= 0x20; //Configure T1 to mode 2
TH1 = 256 - (11059200/12/32) / baud; //Calculate T1 reload value
TL1 = TH1; //initial value equals reload value
ET1 = 0; //Disable T1 interrupt
ES = 1; // Enable serial port interrupt
TR1 = 1; //Start T1
}
void LedScan() //LED display scanning function
{
static unsigned char index = 0;
P0 = 0xFF; // Turn off all segment selection bits and display blanking
P1 = (P1 & 0xF8) | index; //Assign the bit selection index value to the lower 3 bits of port P1
P0 = LedBuff[index]; //Assign the corresponding display buffer value to P0 port
if (index < 5) //Bit selection index 0-5 loop, because there are 6 digital tubes
index++;
else
index = 0;
}
void InterruptTimer0() interrupt 1 //T0 interrupt service function
{
TH0 = T0RH; //Timer reloads the reload value
TL0 = T0RL;
LedScan(); //LED scan display
}
void InterruptUART() interrupt 4
{
if (RI) // Byte received
{
RI = 0; //Manually clear the receive interrupt flag
RxdByte = SBUF; //The received data is saved in the received byte variable
SBUF = RxdByte; //The received data is sent back directly, which is called "echo" to prompt the user whether the information entered has been received correctly
}
if (TI) //bytes sent
{
TI = 0; //Manually clear the transmit interrupt flag
}
}
When you do this experiment, there is a small problem that you should pay attention to. Because the STC89C52RC download program uses the UART serial port to download, after downloading the program, the program runs, but the download software will send some additional data through the serial port at the end, so the program does not display 00 when it is just downloaded, but may display other data. Restart and open it once.
Commonly used characters include numbers from 0 to 9, letters from A to Z/a to z, and various punctuation marks, etc. So how do we represent them in the microcontroller system?
ASCII code (American Standard Code for Information Interchange) can accomplish this mission: in a single-chip microcomputer, a byte of data can have 256 values from 0 to 255. We take 128 values from 0 to 127 and give it another meaning, that is, let them represent a commonly used character respectively. The specific correspondence is shown in the following table.
Table 1-1 ASCII table
In this way, a one-to-one correspondence is established between commonly used characters and byte data. Now a byte can represent both an integer and a character, but it is essentially just a byte of data, which can be given different meanings. When to give it that meaning depends on the programmer's intention. ASCII code is widely used in microcontroller systems. Let's have an intuitive understanding of it and make sure to deeply understand its essence.
By referring to the above table, you can realize the conversion between characters and numbers. For example, still using this program, we change the sending format to character format, and still use hexadecimal receiving, so that it is easier to compare the receiving and digital tube.
Sending a lowercase a in character format returns a hexadecimal 0x61, and the digital tube also displays 61. The decimal corresponding to the character a in the ASCII code table is 97, which is equal to the hexadecimal 0x61; sending a number 1 in character format returns a hexadecimal 0x31, and the digital tube also displays 31. The decimal corresponding to the character 1 in the ASCII table is 49, which is equal to the hexadecimal 0x31. Now everyone should be clear: the so-called hexadecimal sending and receiving are both carried out according to the real value of the byte data; while the character format sending and receiving are carried out according to the character form in the ASCII code table, but it actually transmits a byte of data in the end. Of course, you don't need to remember and understand this table, just check it when you need it.
The study of communication is not as intuitive as the previous control part. Our program can only get a result in the communication part, but we cannot directly see the process. So slowly you may know that there are measuring instruments such as oscilloscopes and logic analyzers. If there are instruments such as oscilloscopes or logic analyzers in the school laboratory or company, you can use them to capture the serial port waveform and understand it intuitively. If you don’t have these instruments yet, you should know what it is first and then talk about it when you have the conditions. Because some tools are relatively expensive, you can try to use the ones in school or company if you have the conditions. Here I use a simple logic analyzer to capture the waveform of serial communication for everyone to see, and you can understand it, as shown in Figure 1-1.
Figure 1-1 Schematic diagram of logic analyzer serial port data
The role of the analyzer and oscilloscope is to capture the waveform of the communication process for analysis. Let's first briefly explain the meaning of the waveform. The left side of the waveform is the low bit, and the right side is the high bit. The waveform on the top is sent by the computer to the microcontroller, and the waveform on the bottom is sent back by the microcontroller to the computer. Take the waveform above as an example. The first bit on the left is the start bit 0. From low to high, it is 10001100. If the order is reversed, it is data 0x31, which is '1' in the ASCII code table. You can notice that the analyzer marks a white dot on each data bit, indicating that it is data. There is no white dot when it is the start bit or no data. The difference between the time mark T1 and T2 is shown on the right as 0.102ms, which is about 1 in 9600. There is a slight deviation, which is within the allowable range. Through Figure 1-1, we can clearly understand the detailed process of serial communication.
Now let's learn more about this. If we use the serial port debugging assistant to directly send a "12" in character format, what should be displayed on our digital tube? What should the serial port debugging assistant return? After testing, we found that our digital tube displays 32, while the serial port debugging assistant returns two hexadecimal data, 31 and 32, as shown in Figure 1-2.
Figure 1-2 Serial port debugging assistant data display
We use a logic analyzer to capture this data, as shown in Figure 1-3.
Figure 1-3 Logic analyzer captures data
For the ASCII code table, the numbers themselves are characters rather than data, so if "12" is sent, it actually sends two characters "1" and "2" separately. The microcontroller receives the first character "1" first, and the corresponding number 31 is displayed on the digital tube, but it immediately receives the character "2" and the digital tube instantly changes from 31 to 32. Visually, we have no way of detecting this rapid change, so we feel that the digital tube directly displays 32.
Previous article:Serial communication between 51 microcontroller and PC
Next article:51 MCU Study Notes [VI] - Serial Communication Experiment
Recommended ReadingLatest update time:2024-11-23 07:41
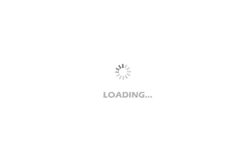
![[Self-study 51 single-chip microcomputer] 11 -- UART serial communication](https://6.eewimg.cn/news/statics/images/loading.gif)
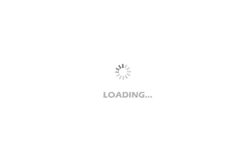
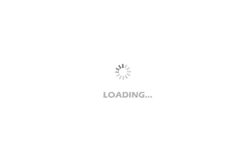
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- Hello everyone, newcomer report
- A Brief Discussion on Synchronization of Multiple Asynchronous Clock Design
- Google deep learning plugin tensorflow
- [GD32F350 Urban Youth Home Security Guard] Part 9: How to perform image processing with GD32
- SG3824 is a push-pull switching power supply without dual PWM output. Can you help me?
- [Sipeed LicheeRV 86 Panel Review] VII. Compiling d1-h_nezha-...
- Optimal control of solar panel position based on GD32E231
- STM32F103C8T6 low power consumption problem
- Counting TI's star products in T-BOX: Automotive Ethernet | Section 1 DP83TC811S-Q1: Automotive Ethernet makes your T-BOX...
- ADAFRUIT PYBADGE LC CAN RUNNING MAKECODE ARCADE, CIRCUITPYTHON AND ARDUINO