MSP 430 MCU key program
#include
#include "key.h"
void Init_Port(void)
{
//Set all pins of P1 port to input mode during initialization
P1DIR = 0;
//Set all pins of P1 port as general I/O ports
P1SEL = 0;
//Set P1.4 P1.5 P1.6 P1.7 as output direction
P1DIR |= BIT4;
P1DIR |= BIT5;
P1DIR |= BIT6;
P1DIR |= BIT7;
//Output low level
P1OUT = 0x00;
//Clear the interrupt register
P1IE = 0;
P1IES = 0;
P1IFG = 0;
//Turn on the interrupt function of the pin
//The corresponding pin jumps from high to low level to set the corresponding flag
P1IE |= BIT0;
P1IES |= BIT0;
P1IE |= BIT1;
P1IES |= BIT1;
P1IE |= BIT2;
P1IES |= BIT2 ;
P1IE |= BIT3;
P1IES |= BIT3;
_EINT();//Turn on interrupt
return;
}
void Delay(void)
{
int i;
for(i = 100;i > 0;i--); //delay a little time
}
int KeyProcess(void)
{
int nP10;
int nP11;
int nP12;
int nP13;
int nRes = 0;
//P1.4 outputs low level
P1OUT &= ~(BIT4);
nP10 = P1IN & BIT0;
if (nP10 == 0) nRes = 13;
nP11 = (P1IN & BIT1) >> 1;
if (nP11 == 0) nRes = 14;
nP12 = (P1IN & BIT2) >> 2;
if (nP12 == 0) nRes = 15;
nP13 = (P1IN & BIT3) >> 3;
if (nP13 == 0) nRes = 16;
//P1.5 outputs low level
P1OUT &= ~(BIT4);
nP10 = P1IN & BIT0;
if (nP10 == 0) nRes = 9;
nP11 = (P1IN & BIT1) >> 1;
if (nP11 == 0) nRes = 10;
nP12 = (P1IN & BIT2) >> 2;
if (nP12 == 0) nRes = 11;
nP13 = (P 1IN & BIT3) >> 3;
if (nP13 == 0) nRes = 12;
//P1.6 outputs low level
P1OUT &= ~(BIT4);
nP10 = P1IN & BIT0;
if (nP10 == 0) nRes = 5;
nP11 = (P1IN & BIT1) >> 1;
if (nP11 == 0) nRes = 6;
nP12 = (P1IN & BIT2) >> 2;
if (nP12 == 0) nRes = 7;
nP13 = (P1IN & BIT3) >> 3;
if (nP13 == 0) nRes = 8;
//P1. 7 Output low level
P1OUT &= ~(BIT4);
nP10 = P1IN & BIT0;
if (nP10 == 0) nRes = 1;
nP11 = (P1IN & BIT1) >> 1;
if (nP11 == 0) nRes = 2;
nP12 = (P1IN & BIT2) >> 2;
if (nP12 == 0) nRes = 3;
nP13 = (P1IN & BIT3) >> 3;
if (nP13 == 0) nRes = 4;
P1OUT = 0x00;//Restore the previous value.
//Read the status of each pin
nP10 = P1IN & BIT0;
nP11 = (P1IN & BIT1) >> 1;
nP12 = (P1IN & BIT2) >> 2;
nP13 = (P1IN & BIT3) >> 3;
for(;;)
{
if(nP10 == 1 && nP11 == 1 && nP12 == 1 && nP13 == 1)
{
//Wait for the key to be released
break;
}
}
return nRes;
}
//Handle interrupt from port 1
#if __VER__ < 200
interrupt [PORT1_VECTOR] void PORT_ISR(void)
#else
#pragma vector=PORT1_VECTOR
__interrupt void PORT_ISR(void)
#endif
{
Delay();
KeyProcess();
if(P1IFG & BIT0)
{
P1IFG &= ~(BIT0);// Clear interrupt flag
}
if(P1IFG & BIT1)
{
P1IFG &= ~(BIT1);// Clear interrupt flag
}
if(P1IFG & BIT2)
{
P1IFG &= ~(BIT2);// Clear interrupt flag
}
if(P1IFG & BIT3)
{
P1IFG &= ~(BIT3);// Clear interrupt flag
}
}
void Init_CLK(void)
{
unsigned int i;
BCSCTL1 = 0X00; // Clear the contents of the register
// XT2 oscillator is turned on
// LFTX1 works in low frequency mode // The division
factor of ACLK is 1 do { IFG1 &= ~OFIFG; // Clear OSCFault flag for (i = 0x20; i > 0; i--); } while ((IFG1 & OFIFG) == OFIFG); // If OSCFault = 1 BCSCTL2 = 0X00; // Clear the contents of the register BCSCTL2 += SELM1; // The clock source of MCLK is TX2CLK, and the division factor is 1 BCSCTL2 += SELS; //The clock source of SMCLK is TX2CLK, and the division factor is 1 }
key.h
void Init_CLK(void);
int KeyProcess(void);
void Delay(void);
int KeySCAN(void);
void Init_Port(void);
Previous article:MSP430 MCU key interrupt program
Next article:Image acquisition solution based on TMS320C6x11 series DSP
Recommended ReadingLatest update time:2024-11-24 18:42
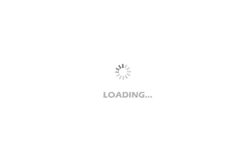
- Popular Resources
- Popular amplifiers
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- MSP430F5529 generates PWM waves with CCS
- Discover Schottky diodes in daily life
- Freescale i.MX Series Products
- Integrated RF power amplifier and filter front end for wireless handsets
- Build Zedboard cross-compilation environment
- TCP protocol requires restarting the circuit board for each connection
- Taiwan's semiconductor manufacturers' performance will grow slowly from July to September due to an increase in PC-related inventory
- Qt Learning Road 45 Model
- EETALK: What products might be reshaped in the 5G era? (Give away 10-100 Chip Coins)
- Hardware/software model and debugging based on embedded ARM processor design