--------------------------------------------------------------------------------
; RAM used by LCD part 0200H~~~~021FH Rn used are R15/R14
; LCD reset subroutine (LCD_REST) does not need to be set in advance and can be called directly
; Half-screen display subroutine (LCD) must be sent to the page address before calling, for example: MOV.B #0B8H,LCD_PAGE_BRAM
; CALL #LCD display upper screen
; :MOV.B #0BAH,LCD_PAGE_BRAM
; CALL #LCD display lower screen
; LCDUP_WORD0_BRAM~~~LCDUP_WORD6_RAM is the code register of the upper screen display word, one screen displays 7 words
; LCDDOWN_WORD0_BRAM~~~LCDDOWN_WORD6_RAM is the code register of the lower screen display word, one screen displays 7 words
; Each screen display is based on the middle as the reference to display evenly on both sides The column address of LCD is automatically calculated when calling the half-screen display subroutine
. The screen clearing instruction can be divided into full-screen clear (LCD_CLEAR_ALL) and half-screen clear (LCD_CLEAR)
. Note that the full-screen clearing subroutine can be called directly without an entry, while the half-screen clearing subroutine has the same entry as the half-screen display subroutine before calling.
#include " MSP 430x14x.h"
NAME LCD
MODULE LCD
PUBL IC LCD
RSEG PROM
;========LCD=====================================
; A0=1 is data, A0=0 is command
#define A0 04H
#define E1 02H
#define E2 01H
#define BIT_OUT P4OUT
#define DATA_BUS P2OUT
;==============LCD____8RAM bit definition================
#define LCD_COM_BRAM 0200H
#define LCD_DATA_BRAM 0201H
#define LCD_LIE_BRAM 0202H
#define LCD_PAGE_BRAM 0203H
;=============LCD_UP_RAM==========================
#define LCDUP_WO RD0_BRAM 0204H
#define LCDUP_WORD1_BRAM 0205H
#define LCDUP_WORD2_BRAM 0206H
#define LCDUP_WORD3_BRAM 0207H
#define LCDUP_WORD4_BRAM 0208H
#define LCDUP_WORD5_BRAM 0209H
#define LCDUP_WORD6_BRAM 020AH
;==========The following RAM is only used in test state=====
#define LCDUP_WORD7_BRAM 020BH
;==============LCD_DOWN_RAM====================
#define LCDDOWN_WORD0_BRAM 020CH
#define LCDDOWN_WORD1_BRAM 020DH
#define LCDDOWN_WORD2_BRAM 020EH
#define LCDDOWN_WORD3_BRAM 020FH
#define LCDDOWN_WORD4_BRAM 0210H
#define LCDDOWN_WORD5_BRAM 0211H
#define LCDDOWN_WORD6_BRAM 0212H
;==========The following RAM is only used in test state=====
#define LCDDOWN_WORD7_BRAM 0213H
;=============LCD_DATA_REGISTER_RAM================
#define LCD_R0_BRAM 0214H
#define LCD_R1_BRAM 0215H
;==============LCD____16RAM bit definition==============
#define LCD_COUNT_WRAM 021CH
#define LCD_WORD_WRAM 021EH
;==============LCD____BIT bit definition==============
#define LCD_BIT_RAM 0220H
#define LCD_E1_E2 0000H
; LCD left and right screen selection: BIT=1 selects the left side, BIT=0 selects the right side
#define LCD_INV 02H
; High level displays inverted
;---------------------------------------------------
;========================================================
LCD_E1_COM
BIC.B #A0,&BIT_OUT ;Set A0 low as command channel
MOV.B LCD_COM_BRAM,&LCD_BUS ;Output command
BIS.B #E1,&BIT_OUT ;Turn on E1
BIC.B #E1,&BIT_OUT ;Turn off E1
RET ;Return
LCD_E2_COM
BIC.B #A0,&BIT_OUT ;Set A0 low as command channel
MOV.B LCD_COM_BRAM,&LCD_BUS ;Output command
BIS.B #E2,&BIT_OUT ;Turn on E2
BIC.B #E2,&BIT_OUT ;Turn off E2
RET ;Return
LCD_E1_DATA
BIS.B #A0,&BIT_OUT ;A0 is set high as command channel
MOV.B LCD_DATA_BRAM,&LCD_BUS ;Output command
BIS.B #E1,&BIT_OUT ;Open E1
BIC.B #E1,&BIT_OUT ;Close E1
RET ;Return
LCD_E2_DATA
BIS.B #A0,&BIT_OUT ;A0 is set high as data channel
MOV.B LCD_DATA_BRAM,&LCD_BUS ;Output command
BIS.B #E2,&BIT_OUT ;Open E2
BIC.B #E2,&BIT_OUT ;Close E2
RET ;Return
;================CLEAR_LCD_DISPALY==============
;You can clear the full screen display by calling the program directly each time without the entry
LCD_CLEAR_ALL
CLR R15
CLR R14
LCD_CLEAR_ALL_1
BIS.B #0B8H,R15
MOV.B R15,LCD_COM_BRAM
CALL #LCD_E1_COM
CALL #LCD_E2_COM
MOV.B #00H,LCD_COM_BRAM
CALL #LCD_E1_COM
CALL #LCD_E2_COM
MOV.B #61D,R14
LCD_CLEAR_ALL_2
MOV. B #000H,LCD_DATA_BRAM
CALL #LCD_E1_DATA
CALL #LCD_E2_DATA
CLRZ
DEC.B R14
JNZ LCD_CLEAR_ALL_2
INC.B R15
CLRZ
CMP.B #0BCH,R15
JNE LCD_CLEAR_ALL_1
RET
;================CLEAR_LCD_DISPALY==============
; Each time you call the program directly, you can clear half of the screen display
; Before calling, you must send the page address to LCD_PAGE_RAM (page address register)
; For example: MOV.B #0B8H,LCD_PAGE_BRAM
; :CALL #LCD_CLEAR is to clear the upper half of the screen display
; :MOV.B #0BAH,LCD_PAGE_BRAM
; :CALL #LCD_CLEAR is to clear the lower half of the screen display
LCD_CLEAR
CLR R15
MOV.B LCD_PAGE_BRAM,LCD_LIE_BRAM
ADD.B #2D,LCD_LIE_BRAM
LCD_CLEAR_1
BIS.B LCD_PAGE_BRAM,R15
MOV.B R15,LCD_COM_BRAM CALL
#LCD_E1_COM
CALL #LCD_E2_COM
MOV.B #00H,LCD_COM_BRAM
CALL #LCD_E1_COM
CALL #LCD_E2_COM
MOV #61D,R14
LCD_CLEAR_2
MOV.B #00H,LCD_DATA_BRAM
CALL #LCD_E1_DATA
CALL #LCD_E2_DATA
CLRZ
DEC.B R14
JNZ LCD_CLEAR_2
INC.B R15
CLRZ
CMP.B LCD_LIE_BRAM,R15
JNZ LCD_CLEAR_1
RET
;===============RESETTING_LCD_MODE=============
;Call this program to reset the LCD and enter the working state
LCD_REST
MOV.B #0E2H,LCD_COM_BRAM ;Reset
CALL #LCD_E1_COM
CALL #LCD_E2_COM
MOV.B #0A4H,LCD_COM_BRAM ; Turn off idle mode
CALL #LCD_E1_COM
CALL #LCD_E2_COM
MOV.B #0A9H,LCD_COM_BRAM ; Set 1/32 duty cycle
CALL #LCD_E1_COM
CALL #LCD_E2_COM
MOV.B #0A0H,LCD_COM_BRAM ; Forward sort setting
CALL #LCD_E1_COM
CALL #LCD_E2_COM
MOV.B #0C0H,LCD_COM_BRAM ; Set the display start line to the first line
CALL #LCD_E1_COM
CALL #LCD_E2_COM
MOV.B #0AFH,LCD_COM_BRAM ; Turn on display setting
CALL #LCD_E1_COM
CALL #LCD_E2_COM
RET
; Check LCD PAGE #0B8H.#0B9H/#0BAH.#0BBH The number of words that need to be displayed on the screen
; This program has been tested 03-3-12 9:55
;R15/R14/R13
LCD_CHK
CLR LCD_COUNT_RAM
MOV.B #8,LCD_R0_RAM ; Check the number of words that need to be displayed on the screen. A screen can display up to 7 16X16
CLR.B LCD_LIE_BRAM ; Clear the screen check result register
MOV LCD_WORD_RAM,R14 ; Put the first 16-bit address of the word to be checked into R14.LCD_WORD_RAM
; It must be a 16-bit address when defined
LCD_CHK_0
DEC.B LCD_R0_RAM
JZ LCD_CHK_1 ; Test completed and exit
CLRZ
MOV.B @R14+,LCD_COUNT_RAM
TST.B LCD_COUNT_RAM ;Test each word register to see if it is zero
JZ LCD_CHK_0 ;When it is zero, transfer to
INC.B LCD_LIE_BRAM ;If it is greater than zero, check the result register and add one
JMP LCD_CHK_0 ;Return test
LCD_CHK_1
CLR LCD_COUNT_RAM
MOV.B LCD_LIE_BRAM,LCD_COUNT_RAM
MOV LCD_COUNT_RAM,&MPY ;Multiply the test result by 16
MOV #16D,&OP2
MOV &RESLO,LCD_COUNT_RAM ;Put the multiplication result back into the register
MOV.B LCD_COUNT_RAM,LCD_LIE_BRAM
RRA.B LCD_LIE_BRAM ;Divide the result by 2 and put it into the register of the upper screen page
MOV.B #61D,LCD_COUNT_RAM
SUB.B LCD_LIE_BRAM,LCD_COUNT_RAM
MOV.B LCD_COUNT_RAM,LCD_LIE_BRAM
RET
;Before calling, the address of the first word to be displayed on the screen must be placed in R15
; Column: MOV #LCDUP_WORD0_BRAM,LCD_WORD_RAM
;MOV #LCDDOWN_WORD0_BRAM,LCD_WORD_RAM
;Registers used R15/R14
LCD
CLRZ
CMP.B #0B8H,LCD_PAGE_BRAM
JNE LCD_1
MOV #LCDUP_WORD0_BRAM,LCD_WORD_RAM
JMP LCD_0
LCD_1 MOV #LCDDOWN_WORD0_BRAM,LCD_WORD_RAM
LCD_0 CALL #LCD_CHK
BIS.B #BIT0,LCD_BIT_RAM
MOV.B #7D,LCD_R1_RAM
;Each call displays 7 16X16 words in succession. When encountering a word register,
MOV LCD_WORD_RAM,R15 ;Zero is returned because there is no zero in the word table
LCD_DISPALY
CLR R14
MOV.B @R15+,R14
TST R14 ;R15 is the first address register of the display word, which is used for indirect addressing
JZ LCD_RET ;If the word register is zero, return it
MOV #32D,&MPY ;Look up the table and send the result to the register
MOV R14,&OP2
MOV &RESLO,LCD_COUNT_RAM
ADD #LCD_DB,LCD_COUNT_RAM
MOV LCD_COUNT_RAM,R14
MOV.B #32D,LCD_R0_RAM ;R14 is used to store the number of calculated search data
LCD_PAGE
MOV.B LCD_PAGE_BRAM,LCD_COM_BRAM;Send the page address to the command register
CLRZ
BIT.B #BIT0,LCD_BIT_RAM
;When BIT0 of LCD_BIT_RAM=LCD_E1_E2 is high, go to E1 to send, the first is E2
JZ LCD_PAGE_E2 ;If Z is zero, go to E2, otherwise work downward
LCD_PAGE_E1
CALL #LCD_E1_COM ;Send page address to E1
JMP LCD_LIE ;Jump to column to send
LCD_PAGE_E2
CALL #LCD_E2_COM ;Send page address to E2
LCD_LIE
MOV.B LCD_LIE_BRAM,LCD_COM_BRAM;Send column address to command register
CLRZ
BIT.B #BIT0,LCD_BIT_RAM
;When LCD_BIT_RAM is high, go to E1 to send, the first is E2
JZ LCD_LIE_E2 ;If Z is zero, go to E2, otherwise work downward
LCD_LIE_E1
CALL #LCD_E1_COM ;Send column address to E1
JMP LCD_DATA
LCD_LIE_E2
CALL #LCD_E2_COM ;Send column address to E2
LCD_DATA
CALL #LCD_FIND_DATA ;Call data sending command
CLRZ
BIT.B #BIT0,LCD_PAGE_BRAM ;Test BIT0 of page register
JNZ LCD_PAGE_0 ;If it is greater than zero (i.e. BIT0=1), transfer to
BIS.B #BIT0,LCD_PAGE_BRAM ;Set BIT0 of page register high (originally low #0B8H/#0BAH)
JMP LCD_RETURN
LCD_PAGE_0
BIC.B #BIT0,LCD_PAGE_BRAM ;Set BIT0 of page register low (originally high #0B9H/#0BBH)
INC.B LCD_LIE_BRAM ;Add column address to one
CLRZ
CMP.B #61D,LCD_LIE_BRAM ;Check if there are more than 61 columns
JNZ LCD_RETURN ;If Z is greater than zero, transfer If it is equal to zero, work downward
CLR.B LCD_LIE_BRAM; clear register
CLRZ
BIT.B #BIT0, LCD_BIT_RAM; test LCD_BIT_RAM bit, if it is E1, switch to E2, if it is E2, immediately return
JNZ LCD_PAGE_1; if Z is zero, switch to E2, otherwise work downward
JMP LCD_RET; switch to subroutine and return command
LCD_PAGE_1
BIC.B #BIT0, LCD_BIT_RAM; set LCD_BIT_RAM bit low and set it to E2 display state
JMP LCD_PAGE; return page transfer command address
LCD_RETURN
CLRZ
DEC.B LCD_R0_RAM; find the number of data register minus 1
JNZ LCD_PAGE; if R14 is greater than zero, switch back to page transfer command address
CLRZ
DEC.B LCD_R1_RAM; decrease the number of words register by 1
BIC.B #BIT0, LCD_PAGE_BRAM
JNZ LCD_DISPALY ;Return to main display calling program
LCD_RET
RET ;Return to main program
;====================================================
LCD_FIND_DATA
MOV.B @R14+,LCD_DATA_BRAM
CLRZ
BIT.B #INV_BIT,LCD_BIT_RAM
JZ NO_INV
INV.B LCD_DATA_BRAM
NO_INV CLRZ
BIT.B #BIT0,LCD_BIT_RAM ;Test LCD_BIT_RAM bit If it is E1, it will turn to E2. If it is E2, it will return to
JZ immediately LCD_FIND_E2 ;If Z is zero, it will turn to E2, otherwise it will work downward
CALL #LCD_E1_DATA ;Call E1 data sending subroutine
JMP LCD_FIND_DATA_RET
LCD_FIND_E2
CALL #LCD_E2_DATA ;Call E2 data sending subroutine
LCD_FIND_DATA_RET
RET
END LCD
Previous article:51 MCU Chinese character LCD subroutine LCD screen is divided into 4 rows * 12 columns of Chinese characters
Next article:Water pump timing control experiment
Recommended ReadingLatest update time:2024-11-16 14:59
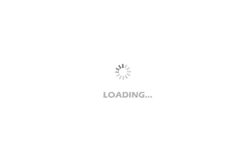
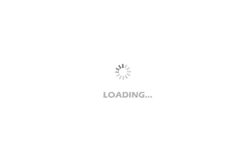
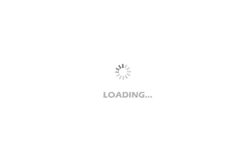
- Popular Resources
- Popular amplifiers
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
Modern Product Design Guide
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Has anyone used ads7844?
- CPU card programming system main frequency setting
- EEWORLD University ---- Automotive eCall Power Solution
- Because he mastered the company's "core technology", the boss hired several strong men to kidnap people on the street after leaving the company
- It's time to test your eyesight
- How to not set a password in AP mode
- LCD Segment Screen Screen Printing Notes
- hfss18 version 3D image setting problem
- The network transformer output does not connect to RJ45, but uses a custom interface!
- EEWORLD University Hall----Live Replay: TI Sitara? Multi-protocol Industrial Communication Optimization Solution, PLC Demo Real-time Demonstration