This digital clock can achieve a variable precision clock with an accuracy error of ≤ 1S/day, and can easily adjust the clock, time, timing time, etc.
LED1 bit p1.0; LED definition
led2 bit p1.1
led3 bit p1.2
led4 bit p1.3
led5 bit p1.4
led6 bit p1.5
led7 bit p1.6
led8 bit p1.7
s1 bit p0.0 ;digit definition of digital tube digits
s2 bit p0.1
s3 bit p0.2
s4 bit p0.3
s5 bit p0.4
s6 bit p0.5
s7 bit p0.6
s8 bit p0.7
led_data equ p2 ;digit definition of digital tube
key1 bit p3.5 ;key definition
key2 bit p3.6
key3 bit p3.7
key equ 56h
time_h equ 57h ;timing initial value high bit
time_l equ 58h ;low bit
t_h equ 60h ;address corresponding to hours, minutes and seconds
t_s equ 61h
t_m equ 62h
time equ 63h ;clock counting unit
timer_h equ 64h ;timing unit
timer_m equ 65h ; Timing sub-unit
timset bit 00h ; Set time mark
disstart equ 70h ; Display unit first address
int_data equ 45h ; Interrupt data address
count_data equ 44h ; Counting unit address
timer_data equ 43h ; Timing address
; ************** The above is the predefined part
; ************** The following program starts
org 00h ; Program start address
jmp main ; Jump to the beginning of the code
org 1bh ; Timer T1 interrupt service program entry
jmp tim1
org 030h ; Main program start address 30H
main: MOV sp,#30h ; First define the stack
lcall rest ; Initialize
lcall pro_set ; Set the timer to start working
lpp: lcall time_set ; Accept the user set time
lcall timer ; Clock processing
lcall time_pro ; Time format processing, code type change, etc.
lcall time_display ; Display
jmp lpp
; ************* Initialization program ***************************
rest: MOV a,#00h ; Accumulator cleared
MOV b,#00h
MOV p0,#0 ; Digital tube disabled
MOV t_h,#0 ; Hour unit
MOV t_m,#0 ; Minute unit
MOV t_s,#0 ; Second unit
MOV time,#00h ; Count overflow times, 20 overflows for one second
clr timset ; Timing set flag, 0-> increase 1-> decrease
MOV timer_h,#12 ; Timer hour unit, set timing to 12:00
MOV timer_m,#00h ; Timer minute unit
MOV p2,#255 ; Disable digital tube
clr beep ; Disable buzzer
ret ; Return
; *************** Timer T1 interrupt service program ****
tim1:clr tr1 ; First stop timing operation
MOV th1,time_h ;
MOV tl1,time_l
inc time ; self-increment
MOV a,time ; get the overflow count
cjne a,#20,retend ; if it reaches 20, it means 1 second
cpl p1.0 ; negate p1.0, p1.1 to indicate seconds
cpl p1.1
MOV time,#00h ;Restart to wait for 1 second
inc t_s ;Increase the second unit by 1
retend:setb tr1 ;Start timing
reti ;Return from interruption
; ***************** Set the timer initialization, the timing time is 50ms ****
pro_set: MOV dptr,#0000h ;Clear the data pointer
MOV tmod,#10h ;Set timer 1 to work in mode 1
MOV time_h,#3ch ;Calculate the initial value required for timing 50ms
MOV th1,time_h ;Save the high bit
MOV time_l,#0c1h ;Low bit
MOV tl1,time_l ;Save the low bit
setb ea ;Total interrupt enable
setb et1 ;Timer 1 enable
setb tr1 ;Timer 1 starts running
ret ;Return
; ****************** timer program mainly completes data processing **********
timer: MOV a,t_s ;Get the second unit data
cjne a,#60,tend ;If the seconds are less than 60, return
MOV t_s,#00h ;Clear the seconds unit
inc t_m ;Add 1 to the minute unit
MOV a,t_m ;Get the minute unit data
cjne a,#60,tend ;If the minute is not equal to 60, return
MOV t_m,#00h ;Clear the minute unit
setb beep ;Short beep reminder on the hour
lcall delay ;Beep delay
clr beep ;Stop the buzzer
inc t_h ;Add 1 to the time unit
MOV a,t_h ;Get the time unit data
cjne a,#24,timetest ;If it is not equal to 24, check the timing
MOV t_h,#00h ;Clear the time unit
jmp tend ;Return
timetest:cjne a,timer_h,tend ;If it is not equal to the time unit of the timing, return
MOV a,t_m ;Get the minute unit of the timing
cjne a,timer_m,tend ;If the current minute is not equal to the timed minute unit, return
setb beep ;When the time is up, beep to remind
lcall delay
clr beep
lcall delay
setb beep
lcall delay
clr beep ;Continuous short sound reminder
tend:ret ;Return
; ************ time_display program is mainly used to display time value *************
time_display: MOV r0,#disstart ;Get the first address of the display unit
MOV r1,#01h ;Start from the first digital tube
MOV r2,#06h ;Total 6 digital tubes
dislp: MOV led_data,@r0 ;Get the current unit data
inc r0 ;Point to the next unit
MOV p0,r1 ;Digital display
MOV a,r1 ;Prepare for the next number
rl a ;Next unit
MOV r1,a ;Save
lcall delay5ms ;In order to ensure the brightness of the digital tube,
;but to prevent flickering, delay 5ms
djnz r2,dislp ;Repeat display until all data are refreshed
ret ;Return
; ******* time_pro time processing, mainly BCD code conversion, table lookup ***********
time_pro:lcall bcd ;BCD code conversion
MOV r0,#disstart ;Get the first address of the display unit
MOV r2,#06h ;The number of units to be converted
prlp: MOV a,@r0 ;Get the data that needs to be converted
MOV dptr,#tab_nu ;Get the table header
MOV c a,@a+dptr ;Get the converted data
MOV @r0,a ;Save it back
inc r0 ;Point to the next
djnz r2,prlp ;Repeat the conversion until all 6 are completed
ret ;Return
; **************** Code conversion ****************************************
bcd: MOV r0,#disstart ;Get the first address
MOV a,t_s ;Get the low bit to be converted
MOV b,#10 ;Convert the base. If you want to convert the base to decimal, change it to 10
div ab ;Calculate A/B
MOV @r0,b ;The first bit is converted. Save the converted low bit data
inc r0 ;Self-increment
MOV @r0,a ;Save the high bit
inc r0 ;Get the second data address
MOV a,t_m ;Get the second data to be converted
MOV b,#10 ;hexadecimal
div ab ;calculate
MOV @r0,b ;store low bit
inc r0
MOV @r0,a ;store high bit
inc r0 ;the third bit
MOV a,t_h ;get data
MOV b,#10 ;hexadecimal
div ab ;calculate
MOV @r0,b ;store low bit
inc r0
MOV @r0,a ;store high bit
ret ;finished, return
;***************************************************************************************
;******** time_set set time ***********************************************************
; * *
; * Detect user key press, 1-> set time unit 2-> set subunit, 3-> set increase and decrease mode*
; * If you need to increase, first set the mode to increase (default is decrease), that is, press KEY3 once, *
; * Then press KEY1 ,If you want to reduce the time unit, you need to press KEY3 again, and then press KEY1; *
; * The same is true for the minute setting. *
;************************************************************************************
time_set: MOV p0,#00h ;Disable the digital tube display
MOV p2,#255 ;Prevent flashing when pressing keys
lcall pro_key ;Search for user key presses
MOV a,key ;Search for key values
jz tsend ;If it is equal to 0, it means that no key is pressed, and it returns directly
cjne a,#1,tset1 ;Is it equal to 1? No next processing procedure
MOV key,#00h ; equal to 1, indicating that the time setting should be zero, otherwise it will cause repeated settings
jb timset,tset10 ; time setting flag, 1 -> decrease, 0 -> increase
MOV a,t_h ; flag 0, increase, get the time unit
cjne a,#23,ts1 ; if the time unit is not equal to 23, transfer to the increase operation
jmp tsend ; equal to 23 directly return
ts1: inc t_h ; the time unit increases by 1
jmp tsend ; return
tset10: MOV a,t_h ; here it is handled as a decrease
jz tsend ; if the time unit is 0, directly return
dec t_h ; otherwise, the data is reduced by 1
jmp tsend ; return, the following settings for points are the same
tset1:cjne a,#2,tset2 ;If the key is not 2, then transfer to the next process
MOV key,#00h ;It is 2, indicating setting
jb timset,tset20 ;The rest is the same as above
MOV a,t_m
cjne a,#59,
ts2 jmp tsend
ts2:inc t_m
jmp tsend
tset20: MOV a,t_m
jz tsend
dec t_m
jmp tsend
tset2:cjne a,#3,tsend ;Equal to 3 means setting the flag
MOV key,#00h ;Clear
cpl timset ;Invert the flag
tsend:ret ;Return
;************ Key processing Read the keyboard *************************
;See the instructions for keyboard query, and the previous related program
pro_key: ;Keyboard query subroutine
setb key1 ;First output high level and detect the arrival of low level
setb key2 ;Different circuits may have different detection methods
setb key3
jb key1,ke1 ;If the user does not press the first key, go to the next processing part,
MOV key,#1 ;Indicates that the user pressed the first key
lcall delay20ms ;Software delay to prevent interference
jmp pro_key ;Re-query until the user releases the key
ke1:jb key2,ke2 ;Process the second key, if not go to the next processing part
MOV key,#2 ;The following is similar to the first processing unit.
lcall delay20ms
jmp pro_key
ke2:jb key3,ke3
MOV key,#3
lcall delay20ms
jmp pro_key
ke3:ret
;**************************************
;******** Timer T0 setting **************
;The timer works in mode 1. To improve the accuracy, the total timing time is 50ms.
;The timer overflows 20 times for 1 second.
pro_timer: MOV tmod,#01h ;Set the timer to timing mode 1
MOV th0,#0ffh ;Initialize the initial value of the timing
MOV tl0,#0a1h ;
setb ea ;Total interrupt enable
setb et0 ;Timer 0 enable
setb tr0 ;Start timing
ret ;Return
; *********************************************
; *************** Software delay *************
delay:push psw ;Save the original register content
clr psw.3 ;
clr psw.4 ;Set a new register group
MOV r0,#2 ;Delay parameter 1
MOV r1,#250 ;Delay parameter 2
MOV r2,#2 ;Delay parameter 3
dl1:djnz r0,dl1 ;Delay loop 1
MOV r0,#250 ;
dl2:djnz r1,dl1 ;Delay loop 2
MOV r0,#240 ;
MOV r1,#248 ;
dl3:djnz r2,dl1 ;Delay loop 3
nop ;Timing accuracy adjustment
pop psw ;Restore the original register
ret ;Return
;********************************************
;*************** Keyboard delay ***************
delay20ms:push psw
clr psw.3
clr psw.4
MOV r0,#250
MOV r1,#40
d20:djnz r0,d20
MOV r0,#250
djnz r1,d20
pop psw
ret
;**************************************
;*********** Delay 5ms ****************
delay5ms:push psw
clr psw.3
setb psw.4
MOV r0,#250
MOV r1,#10
d5:djnz r0,d5
MOV r0,#250
djnz r1,d5
pop psw
ret
;************************************************************************************
;This is a digital display table, in which the numbers with decimal points are 16 larger than the numbers without decimal points
; For example, the display code of 0 is 0; then the display code of 0 is 16; and so on
tab_nu:db 0c0h, 0f9h, 0a4h, 0b0h, 99h , 92h , 82h, 0f8h ; digits 0-7 without decimal point codedb
80h , 90h, 88h , 83h , 0c6h, 0a1h, 86h, 8eh ; digits 8-f without decimal point codedb
40h , 79h, 24h , 30h , 19h , 12h , 02h, 78h ; digits 0-7 with decimal point codedb
00h , 10h, 08h , 03h , 46h , 21h , 06h, 0eh ; digits 8-f with decimal point code
Previous article:Seconds timer program
Next article:10-bit temperature sensor AD7416 driver
Recommended ReadingLatest update time:2024-11-16 13:56
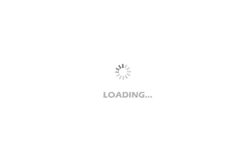
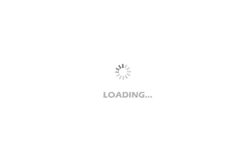
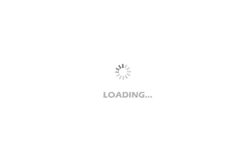
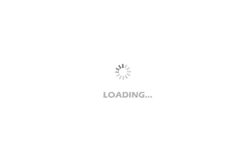
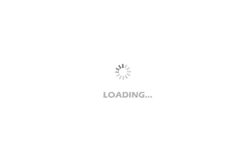
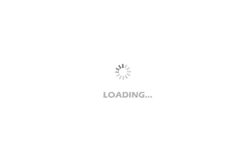
- Popular Resources
- Popular amplifiers
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Matter and Wi-Fi 6: An ideal combination
- Creativity + Focus | ADI Model of “Garage Innovation”
- 【XMC4800 Relax EtherCAT Kit Review】+ Getting started with DAVE, simple application of ADC module
- Half a year has passed, let’s share what we have gained in EEWORLD!
- MSP430F5529 generates PWM waves with CCS
- FPGA Multiplier
- Quartus2 simulation can not produce waveform
- 2. GD32L233C-START environment construction
- AI automatically generates Spring Festival couplets to warm up for FZ5
- STM32F407VG mount SD card