*——————————————————————
〖Description〗SPI bus driver includes the standard mode of ordinary package and the standard mode of special package,
and the default crystal oscillator is 11.0592MHz.
〖File〗93CXX.C ﹫2003/5/12
〖Version〗V3.00A Build 0803
———————————————————————*/
/*General 93c06-93c86 series instructions
93c06=93c46 93c56=93c66 93c76=93c86
DIPx can be defined by yourself*/
#include "reg51.h"
#include "intrins.h"
/*-----------------------------------------------------
SPI93cXX series timing function call (ordinary package)
Calling method: self-define ﹫2001/05/12
Function description: Private function, encapsulates various interface definitions
-----------------------------------------------------*/
#define di_93 dip3
#define sk_93 dip2
#define cs_93 dip1
#define do_93 dip4
#define gnd_93 dip5
#define org_93 dip6
sbit cs_93=P1^0;
sbit sk_93=P1^1;
sbit di_93=P1^2;
sbit do_93=P1^3;
sbit org_93=P0^4;
/*-----------------------------------------------------
SPI93cXX series timing function call (normal package)
Calling method: void high46(void) ---high 8-bit function call
void low46(void) ---low 8-bit function call ﹫2001/05/12
Function description: Private function, SPI dedicated 93c46 normal package driver program
-----------------------------------------------------*/
void high46(void)
{
di_93=1;
sk_93=1; _nop_();
sk_93=0;_nop_();
}
void low46(void)
{
di_93=0;
sk_93=1;_nop_();
sk_93=0;_nop_();
}
void wd46(unsigned char dd)
{
unsigned char i;
for (i=0;i<8;i++)
{
if (dd>=0x80) high46();
else low46();
dd=dd<<1;
}
}
unsigned char rd46(void)
{
unsigned char i,dd;
do_93=1;
for (i=0;i<8;i++)
{
dd<<=1;
sk_93=1;_nop_();
sk_93=0;_nop_();
if (do_93) dd|=1;
}
return(dd);
}
/*-----------------------------------------------------
SPI93cXX series timing function call (special package)
Calling method: self-defined﹫2001/05/12
Function description: private function, special package interface definition
-----------------------------------------------------*/
#define di_93a dip5
#define sk_93a dip4
#define cs_93a dip3
#define do_93a dip6
#define gnd_93a dip7
#define v CC _93a out_vcc(2)
sbit cs_93a=P1^0;
sbit sk_93a=P1^1;
sbit di_93a=P1^2;
sbit do_93a=P1^3;
/*-----------------------------------------------------
SPI93cXX series timing function call (special package)
Calling method: void high46a(void) --- High 8-bit function call
void low46a(void) --- Low 8-bit function call ﹫2001/05/12
Function description: Private function, SPI dedicated 93c46 special package driver program
-----------------------------------------------------*/
void high46a(void)
{
di_93a=1;
sk_93a=1;_nop_();
sk_93a=0;_nop_();
}
void low46a(void)
{
di_93a=0;
sk_93a=1;_nop_();
sk_93a=0;
_nop_ ();
}
void wd46a(unsigned char dd)
{
unsigned char i;
for (i=0;i<8;i++)
{
if (dd>=0x80) high46a();
else low46a();
dd=dd<< 1;
}
}
unsigned char rd46a(void)
{
unsigned char i,dd;
do_93a=1;
for (i=0;i<8;i++)
{
dd<<=1;
sk_93a=1;_nop_();
sk_93a=0;_nop_();
if (do_93a) dd|=1;
}
return(dd);
}
/*------------------------ -----------------------------
SPI93c46 series function call (example)
Calling method: bit write93c56_word(unsigned int address,unsigned int dat) ﹫2001/05/12
Function description: Private function, SPI only
------------------------------------ -----------------*/
void ewen46(void)
{
_nop_();
cs_93=1;
high46();
wd46(0x30);
cs_93=0;
}
unsigned int read93c46_word (unsigned char address)
{
unsigned int dat;
unsigned char dat0,dat1;
gnd_93a=0;
gnd_93=0;
cs_93=sk_93=0;
org_93=1;
cs_93=1;_nop_();
address=address>>1;
address=address|0x80;
address=address|0x80;
high46();
wd46(address);
dat1=rd46();
dat0=rd46( );
cs_93=0;
dat=dat1*256+dat0;
return(dat);
}
bit write93c46_word(unsigned char address,unsigned int dat)
{
unsigned char e,temp=address;
e=0;
while (e<3)
{
gnd_93a=0;
gnd_93=0;
cs_93=sk_93=0;
org_93=1;
cs_93=1;
ewen46();
_nop_();
cs_93=1;
_nop_();
high46();
address|=0x80;
address>>=1;//??
address|=0x40;
wd46(address);
wd46(dat/256);
wd46(dat%256);
cs_93=0;
_nop_();
cs_93=1 ;
time=0;do_93=1;
while (1)
{
if (do_93==1) break;
if (time>20) break;
}
cs_93=0;
if (read93c46_word(temp)==dat)
{
return(0 );
}
e++;
}
return(1);
}
/*------------------------------------ -----------------
SPI93c57 series function call (example)
Calling method: bit write93c57_word(unsigned int address,unsigned int dat) ﹫2001/05/12
Function description: Private function, SPI dedicated
-----------------------------------------------------*/
void ewen57(void)
{
_nop_();
cs_93=1;
dip7=0;
high46();
low46();
wd46(0x60);
cs_93=0;
}
unsigned int read93c57_word(unsigned int address)
{
unsigned int dat;
unsigned char dat0,dat1;
gnd_93=0;
cs_93=sk_93=0;
org_93=1;
cs_93=1;
address=address>>1;
high46();
high46();
wd46(address);
dat1=rd46();
dat0=rd46();
cs_93=0;
dat=dat1*256+dat0;
return(dat);
}
bit write93c57_word(unsigned int address,unsigned int dat)
{
unsigned char e;
unsigned int temp=address;
e=0;
while (e<3)
{
gnd_93=0;
cs_93=sk_93=0;
org_93= 1;
cs_93=1;
ewen57();
cs_93=1;
_nop_();
high46();
low46();
address>>=1;
address|=0x80;
wd46(address);
wd46(dat/256);
wd46(dat%256); cs_93=
0; _nop_
() ; 3=1; while (1) { if (do_93==1) break; if (time>20) break; } cs_93=0; if (read93c57_word(temp)==dat) { return(0); } e++; } return(1); } /*----------------------------------------------------- SPI93c56 series function call (example) Calling method: bit write93c56_word(unsigned int address,unsigned int dat) ﹫2001/05/12 Function description: Private function, SPI dedicated -----------------------------------------------------*/ void ewen56(void) { _nop_(); cs_93=1; high46(); low46(); low46(); wd46(0xc0); cs_93=0; } unsigned int read93c56_word(unsigned char address) { unsigned int dat; unsigned char dat0,dat1; gnd_93=0; cs_93=sk_93=0; org_93=1; cs_93=1; address=address>>1; high46(); high46(); low46(); wd46(address); dat1=rd46() ; dat0 = rd46() ; return (dat); } bit write93c56_word(unsigned char address,unsigned int dat) { unsigned char e; unsigned int temp=address; e=0;
while (e<3)
{
gnd_93=0;
cs_93=sk_93=0;
org_93=1;
cs_93=1;
ewen56();
_nop_();
cs_93=1;
_nop_();
high46();
low46();
high46 ();
address>>=1;
wd46(address);
wd46(dat/256);
wd46(dat%256);
cs_93=0;
_nop_();
cs_93=1;
TH0=0;
time=0;
do_93= 1;
while (1)
{
if (do_93==1) break;
if (time) break;
}
cs_93=0;
if (read93c56_word(temp)==dat)
{
return(0);
}
e++;
}
return(1 );
}
/*------------------------------------------------ --------
SPI93c76 and SPI93c86 series function call (example)
Calling method: bit write93c76_word (unsigned int address, unsigned int dat) ﹫2001/05/12
Function description: Private function, SPI dedicated
------------- ----------------------------------------*/
void ewen76(void)
{
_nop_( );
cs_93=1;
dip7=1;
high46();
low46();
low46();
high46();
high46();
wd46(0xff);
cs_93=0;
}
unsigned int read93c76_word(unsigned int address)
{
unsigned char dat0,dat1;
gnd_93=0;
cs_93=sk_93=0;
org_93=1;
cs_93=1;
address>>=1;
high46();
high46();
low46();
if((address&0x200)==0x200) high46();
else low46();
if ((address&0x100)==0x100) high46();
else low46();
wd46(address);
dat1=rd46();
dat0=rd46 ();
cs_93=0;
return(dat1*256|dat0);
}
bit write93c76_word(unsigned int address,unsigned int dat)
{
unsigned char e;
unsigned int temp=address;
e=0;
address>>=1;
while (e<3)
{
gnd_93=0;
cs_93=sk_93=0;
org_93=1;
cs_93=1;
ewen76();
_nop_();
cs_93=1;
high46();
low46();
high46();
if((address&0x200)==0x200) high46();
else low46();
if ((address&0x100)==0x100) high46();
else low46();
wd46(address);
wd46(dat/256 );
wd46(dat%256);
cs_93=0;_nop_();cs_93=1;
time=0;do_93=1;
while (1)
{
if (do_93==1) break;
if (time>10) break ;
}
cs_93=0;
e++;
}
return(1);
}
/*--------------------------------- -------------------
Main function call (example)
Calling method: main() ﹫2001/05/12
Function description: Private function, dedicated to SPI
-----------------------------------------------------*/
main()
{ bit b;
unsigned int i;
unsigned int j[32],k;
for(i=0;i<32;i++)
j[i]=read93c56_word(i);
for(i=0;i<32;i++)
write93c56_word(i,0x0909);
i=0;
b=write93c56_word(i,0x0909);
j[i]=read93c56_word(i);
i=1;
b=write93c56_word(i,0x1111);
j[i]=read93c56_word(i);
i=2;
b=write93c56_word(i,0x2222);
j[i]=read93c56_word(i);
}
Previous article:X25045/X5045 standard function set based on C51
Next article:51 MCU IO port simulation serial communication program design example
Recommended ReadingLatest update time:2024-11-16 15:50
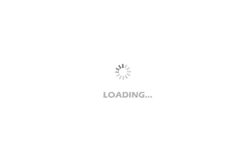
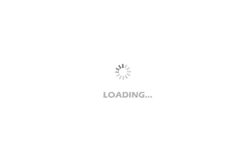
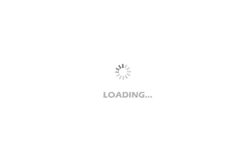
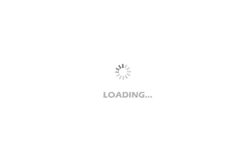
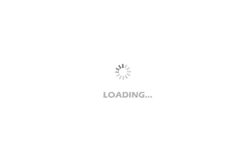
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- UWB can use the frequency band in China,,,,
- What should I do if I have a colleague who is nagging and incompetent?
- A feasible solution to the problem that the USB-Blaster driver cannot be successfully installed during the Quartus II 9 software installation and configuration
- The SGM42507 is a full-bridge driver designed for small motors and electromagnets.
- EEWORLD University ---- HVI Series: Design Considerations for High Power Density and High Efficiency Adapters
- MSP430Ware use notes to initialize XT1
- Open source program for automatic generation of dq current lookup table for permanent magnet synchronous motor of electric vehicle based on weak magnetic field lookup method
- What are the causes of IGBT damage in variable frequency power supply?
- Design and Application of Serial Communication Interface between TMS320F24x and PC
- A new interpretation of the principle of integral circuits: the transformation of amplifiers and capacitors