1. Comprehensive description of GPIO
Each GPIO port of stm32 has two 32-bit configuration registers (GPIOx_CRL, GPIOx_CRH), two 32-bit data registers (GPIOx_IDR, GPIOx_ODR), one 32-bit set/reset register (GPIOx_BSRR), one 16-bit reset register (GPIOx_BRR) and one 32-bit lock register (GPIOx_LCKR).
1. Each IO pin can be configured into the following modes using software:
1. Floating input
2. With pull-up input
3. With pull-down input
4. Analog Input
5. Open-drain output - (this mode can achieve hotpower's true bidirectional IO)
6. Push-pull output
7. Push-pull output of multiplexed functions
8. Open-drain output of multiplexed function
Mode 7 and Mode 8 need to be determined according to the specific multiplexing function.
Each IO pin can be programmed individually, but each IO register can only be accessed by 32 bits (half-word or byte access is prohibited).
(ii) Special registers (GPIOx_BSRR and GPIOx_BRR) implement atomic operations on GPIO ports, which avoids the "read-modify-write" operation when setting or clearing I/O ports, so that the operation of setting or clearing I/O ports will not be interrupted by interrupt processing and cause malfunctions.
(iii) Each GPIO port can be used as an external interrupt input, facilitating flexible system design.
(iv) In the output mode of the I/O port, there are three output speeds to choose from (2MHz, 10MHz and 50MHz), which is beneficial to noise control.
5. All I/O ports are compatible with CMOS and TTL, and most I/O ports are compatible with 5V level.
(VI) High current driving capability: The GPIO port can provide or absorb 8mA current when the high and low levels are 0.4V and VDD-0.4V respectively; if the input and output levels are relaxed to 1.3V and VDD-1.3V respectively, it can provide or absorb 20mA current.
(VII)It has an independent wake-up I/O port.
8. The multiplexing functions of many I/O ports can be remapped.
(IX) The GPIO port configuration has a locking function. After the GPIO port is configured, the configuration combination can be locked by the program until the next chip reset. This function is very helpful in protecting other devices in the system in the event of a program failure, and will not be damaged due to the configuration change of some I/O ports - such as an input port becoming an output port and outputting current.
2. GPIO Configuration
(I) GPIO mode selection and speed matching
(1) Floating input_IN_FLOATING——Floating input, can be used for KEY identification, RX1.
(2) With pull-up input_IPU——IO internal pull-up resistor input.
(3) Input with pull-down_IPD——IO internal pull-down resistor input.
(4) Analog Input_AIN - Use ADC analog input or save power in low power mode.
(5) Open-drain output_OUT_OD - IO output 0 is connected to GND, IO output 1 is left floating, and an external pull-up resistor is required to achieve a high output level. When the output is 1, the state of the IO port is pulled high by the pull-up resistor, but because it is an open-drain output mode, the IO port can also be changed to a low level or unchanged by an external circuit. The IO input level change can be read to realize the IO bidirectional function of C51.
(6) Push-pull output_OUT_PP - IO output 0 - connected to GND, IO output 1 - connected to VCC, the read input value is unknown.
(7) Multiplexed function push-pull output _AF_PP - on-chip peripheral function (SCL, SDA of I2C)
(8) Multiplexed function open-drain output _AF_OD - on-chip external functions (TX1, MOSI, MISO.SCK)
Speed matching of GPIO outputs:
GPIO_Speed_10MHz Maximum output rate 10MHz
GPIO_Speed_2MHz Maximum output rate 2MHz
GPIO_Speed_50MHz Maximum output rate 50MHz
In the output mode of the I/O port, there are 3 output speeds to choose from (2MHz, 10MHz and 50MHz). This speed refers to the response speed of the I/O port drive circuit rather than the speed of the output signal. The speed of the output signal is related to the program (the chip has arranged multiple output drive circuits with different response speeds in the output part of the I/O port, and users can choose the appropriate drive circuit according to their needs). By selecting different output drive modules by selecting the speed, the best noise control and power consumption reduction can be achieved. The high-frequency drive circuit also has high noise. When a high output frequency is not required, please choose a low-frequency drive circuit, which is very helpful to improve the EMI performance of the system. Of course, if you want to output a higher frequency signal, but choose a lower frequency drive module, you may get a distorted output signal.
The key point is that the GPIO pin speed should match the application (10 times or more is recommended). For example:
1) For the serial port, if the maximum baud rate is only 115.2k, then a 2M GPIO pin speed is sufficient, which saves power and reduces noise.
2) For the I2C interface, if you use a 400k baud rate, if you want to leave more margin, then the 2M GPIO pin speed may not be enough. In this case, you can choose a 10M GPIO pin speed.
3) For the SPI interface, if you use 18M or 9M baud rate, the 10M GPIO pin speed is obviously not enough, and you need to use a 50M GPIO pin speed.
(II) How to configure IO ports used by on-chip peripherals in STM32
①Configure the input clock; ②It is activated (turned on) after initialization; ③If the input and output pins of the peripheral are used, the corresponding GPIO port needs to be configured (otherwise the corresponding input and output pins of the peripheral can be used as ordinary GPIO pins); ④Then configure the peripheral in detail.
There are three situations corresponding to the input and output functions of peripherals:
① The corresponding pin of the peripheral is output: the corresponding pin needs to be selected as the push-pull output of the multiplexing function or the open-drain output of the multiplexing function according to the configuration of the peripheral circuit.
② The corresponding pin of the peripheral is input: you can choose floating input, input with pull-up or input with pull-down according to the configuration of the peripheral circuit.
③ADC corresponding pins: configure the pins as analog input.
If the port is configured as an alternate output function, the pin is disconnected from the output register and connected to the output signal of the on-chip peripheral. After configuring the pin as an alternate output function, if the peripheral is not activated, its output will be undefined.
(III) General purpose IO port (GPIO) initialization:
1. RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | B | C, ENABLE): Enable the APB2 bus peripheral clock;
2. RCC_APB2PeriphResetCmd (RCC_APB2Periph_GPIOA | B | C, DISABLE): release GPIO reset;
3. Configure each PIN port (analog input_AIN, input floating_IN_FLOATING, input pull-up_IPU, input pull-down_IPD, open-drain output_OUT_OD, push-pull output_OUT_PP, push-pull multiplexed output_AF_PP, open-drain multiplexed output_AF_OD) and matching speed.
4. GPIO initialization completed
Note 1: PLL (Phase Locked Loop): A phase-locked loop or phase-locked loop, which is used to unify and integrate clock signals so that the memory can access data correctly. PLL is used as a feedback technology in oscillators. For many electronic devices to work properly, they usually need the external input signal to be synchronized with the internal oscillation signal, and this purpose can be achieved using a phase-locked loop.
Note 2: Output of STM32 GPIO port: open-drain output and push-pull output
1. The difference between push-pull output and open-drain output:
>>Push-pull output: can output high and low levels, connect digital devices
>>Open-drain output: The output terminal is equivalent to the collector of the transistor. A pull-up resistor is required to obtain a high-level state. It is suitable for current-type driving and has a relatively strong ability to absorb current (generally within 20ma).
The push-pull structure generally refers to two transistors being controlled by two complementary signals, and one transistor is always turned on while the other is turned off.
To realize line-and, an OC (open collector) gate circuit is required. It is two transistors or MOSFETs with the same parameters, which exist in the circuit in a push-pull manner, each responsible for the waveform amplification task of the positive and negative half cycles. When the circuit is working, only one of the two symmetrical power switch tubes is turned on at a time, so the conduction loss is small and the efficiency is high. The output can both inject current into the load and extract current from the load.
When the port is configured as output:
Open-drain mode: When outputting 0, N-MOS is turned on, P-MOS is not activated, and outputs 0.
When outputting 1, N-MOS is high impedance, P-MOS is not activated, and output is 1 (external pull-up circuit is required). That is to say, in this mode, only N-MOS plays a role in output; in this mode, the port can be used as a bidirectional IO.
Push-pull mode: When output is 0, N-MOS is turned on, P-MOS is high impedance, and output is 0.
When outputting 1, N-MOS is high impedance, P-MOS is turned on, and output is 1 (no external pull-up circuit is required).
Simply put, the open drain is connected to GND when it is 0 and floating when it is 1; the push-pull is connected to GND when it is 0 and connected to VCC when it is 1.
2. Characteristics and applications of open-drain circuit
When designing circuits, we often encounter the concepts of open drain and open collector. The "drain" mentioned in the concept of open drain circuit refers to the drain of MOSFET. Similarly, the "collector" in the open collector circuit refers to the collector of the transistor. An open drain circuit refers to a circuit with the drain of MOSFET as the output. The general usage is to add a pull-up resistor to the circuit outside the drain. A complete open drain circuit should consist of an open drain device and an open drain pull-up resistor.
The circuit that makes up the open-drain form has the following characteristics:
1) Use the driving capability of the external circuit to reduce the internal driving of the IC. When the internal MOSFET of the IC is turned on, the driving current flows from the external VCC through R pull-up, MOSFET to GND. Only a very low gate driving current is required inside the IC.
2) You can connect multiple open-drain output pins to one line to form an "AND logic" relationship. When any one of PIN_A, PIN_B, or PIN_C becomes low, the logic on the open-drain line becomes 0. This is also the principle of I2C, SMBus, and other buses to determine the bus occupancy status.
3) The transmission level can be changed by changing the voltage of the pull-up power supply. The logic level of the IC is determined by the power supply Vcc1, and the output high level is determined by Vcc2. In this way, we can use low-level logic to control the output high-level logic.
4) If the open-drain pin is not connected to an external pull-up resistor, it can only output a low level (therefore, for the P0 port of the classic 51 microcontroller, an external pull-up resistor must be added to perform input and output functions, otherwise it cannot output high-level logic).
5) Standard open-drain pins generally only have output capabilities. Adding other judgment circuits can enable bidirectional input and output capabilities.
Note in application:
1) The principles of open drain and open collector are similar. In many applications, we use open collector circuits instead of open drain circuits. For example, an input pin is required to be driven by an open drain circuit. Then our common driving method is to use a triode to form an open collector circuit to drive it, which is convenient and cost-saving.
2) The resistance value of the pull-up resistor R pull-up determines the speed of the edge of the logic level conversion. The larger the resistance value, the lower the speed and the lower the power consumption. Vice versa.
Push-Pull output is generally called push-pull output. Push-Pull output is more suitable in CMOS circuits because the push-pull output capacity in CMOS cannot be as large as that of bipolar. The output capacity depends on the area of the N-tube and P-tube of the internal output electrode of the IC. Compared with open-drain output, the high and low levels of push-pull are determined by the power supply of the IC, and simple logical operations cannot be performed. Push-pull is the most commonly used output stage design method in CMOS circuits.
3. What are OC and OD?
Open collector gate (open collector OC or open source OD)
Open-drain means open-drain output, which is equivalent to open-collector output, that is, open-collector (OC) output in TTL. It is generally used for line-or, line-and, and some are used for current drive.
Open-drain refers to MOS tubes, and open-collector refers to bipolar tubes. There is no difference in usage.
The open-drain circuit has the following characteristics:
1) Use the driving capability of the external circuit to reduce the internal driving of the IC. Or drive a load higher than the chip power supply voltage.
2) Multiple open-drain output pins can be connected to one line. Through a pull-up resistor, an "AND logic" relationship is formed without adding any devices. This is also the principle of I2C, SMBus and other buses to determine the bus occupancy status. If it is used as a totem output, a pull-up resistor must be connected. When connected to a capacitive load, the falling delay is the transistor in the chip, which is actively driven and has a faster speed; the rising delay is a passive external resistor with a slow speed. If high speed is required, the resistance should be small and the power consumption will be large. Therefore, the selection of load resistance should take into account both power consumption and speed.
3) The transmission level can be changed by changing the voltage of the pull-up power supply. For example, adding a pull-up resistor can provide TTL/CMOS level output.
4) If the open drain pin is not connected to an external pull-up resistor, it can only output a low level. Generally speaking, the open drain is used to connect devices of different levels and match the levels.
5) The normal CMOS output stage consists of two tubes, upper and lower. Removing the upper tube will result in OPEN-DRAIN. This output has two main purposes: level conversion and line-AND.
6) Since the drain is open, the subsequent circuit must be connected to a pull-up resistor. The power supply voltage of the pull-up resistor can determine the output level. In this way, you can perform arbitrary level conversion.
7) The wired-and function is mainly used in situations where multiple circuits pull down the same signal. If this circuit does not want to pull down, it will output a high level. Because the tube on the OPEN-DRAIN is removed, the high level is achieved by an external pull-up resistor. (For a normal CMOS output stage, if one output is high and the other is low, it is equivalent to a power short circuit.)
8) OPEN-DRAIN provides a flexible output mode, but it also has its weakness, which is the delay of the rising edge. Because the rising edge charges the load through an external pull-up passive resistor, when the resistor is small, the delay is small, but the power consumption is large; on the contrary, the delay is large and the power consumption is small. Therefore, if there is a requirement for delay, it is recommended to use the falling edge output.
4. What is Line-OR logic and Line-AND logic?
On a node (line), connect a pull-up resistor to the power supply VCC or VDD and the collector C or drain D of n NPN or NMOS transistors. The emitters E or sources S of these transistors are connected to the ground line. As long as one transistor is saturated, the node (line) is pulled to the ground level.
Because the base of these transistors injects current (NPN) or the gate adds a high level (NMOS), the transistor will be saturated, so the relationship between these bases or gates to this node (line) is NOR logic. If an inverter is added after this node, it is OR logic.
Note: My personal understanding: Line AND, connect the pull-up resistor to the power supply. (~A)&(~B)=~(AB), the origin of the concept of line AND is easier to understand from the formula;
If you use a pull-down resistor and a PNP or PMOS tube, you can form NAND logic, or use negative logic to convert AND/OR logic.
Note: Line OR, connect pull-down resistor to ground. (~A) (~B)=~(AB);
These transistors are often the open collector OC or open source OD output terminals of some logic circuits. This logic is usually called wired AND/wired OR logic. When you see the OC or OD output terminals of some chips connected together and there is a pull-up resistor, this is wired OR/wired AND, but sometimes the pull-up resistor is built into the input terminal of the chip.
By the way, if it is not an OC or OD chip, the output terminals cannot be connected together. The bidirectional output terminals on the bus are managed when connected together. Only one can be used for output at the same time, while the others are in high impedance state and can only be used for input.
5. What is push-pull structure?
Generally, it means that two transistors are controlled by two complementary signals respectively, and one transistor is always turned on while the other is turned off. To realize the line and, an OC (open collector) gate circuit is required. If there are two transistors in the output stage, one is always turned on and the other is turned off, that is, the two transistors are connected in a push-pull manner. Such a circuit structure is called a push-pull circuit or a totem-pole output circuit (unfortunately, the picture cannot be posted). When the output is low, that is, when the lower load gate inputs a low level, the current at the output end will be the lower gate injected into T4; when the output is high, that is, when the lower load gate inputs a high level, the current at the output end will be the lower gate pulled out from the power supply of this level through T3 and D1. In this way, when the output is high or low, T3 and T4 will work alternately, thereby reducing power consumption and improving the bearing capacity of each tube. In addition, no matter which way is taken, the on-resistance of the tube is very small, so the RC constant is very small and the conversion speed is very fast. Therefore, the push-pull output stage not only improves the load capacity of the circuit, but also improves the switching speed. For your reference. The push-pull circuit is a circuit in which two transistors or MOSFETs with the same parameters exist in the circuit in a push-pull manner, each responsible for the waveform amplification task of the positive and negative half cycles. When the circuit is working, only one of the two symmetrical power switch tubes is turned on at a time, so the conduction loss is small and the efficiency is high. The output can either inject current into the load or extract current from the load.
Previous article:STM32 Learning 005_Port Multiplexing and Remapping
Next article:STM32 Learning 006_Systick Usage (I)
Recommended ReadingLatest update time:2024-11-16 21:46
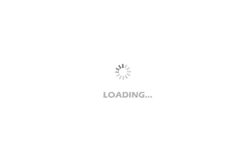
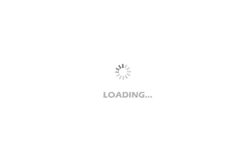
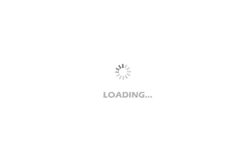
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Example of sending and receiving text messages (AT commands) with MSP430 and TC35I modules
- Which domestic DC-DC and LDO should I choose?
- 【CH579M-R1】+ Turn on timer 0 to control LED flashing and refresh LCD time display
- Xunwei i.MX6ULL development board Platform device driver running test
- Huawei and Alibaba employees who switched to Microsoft were boycotted just because of the crazy overtime?
- [Analysis of the topic of the college electronic competition]——2022 TI Cup Shanghai C topic "Active two-way audio amplifier circuit"
- PYPL programming language popularity ranking in December 2019
- Smart wearable devices based on RSL10
- Soft start control software
- 2006 Germany World Cup knockout stage schedule