The basic timer only has the most basic timing function, that is, when the accumulated number of clock pulses exceeds the preset value, it can trigger an interrupt or trigger a DMA request.
The following settings are required to use the timing function of the basic timer:
1) Enable the timer clock.
2) Set the pre-scaling number.
3) Set the counter value.
4) Set the sampling frequency division number.
5) Set the counting mode, up or down. TIM6 and TIM7 only have up counting function.
6) Enable interrupts, configure interrupt groups and interrupt service functions.
The configuration function is as follows:
/**************************************************************
** Function name: TIM6_Config
** Function description: Basic timer configuration
** Input parameters: None
** Output parameters: None
** Note: Timing time = (prescaler number + 1) * (count value + 1) / TIM6 clock (72MHz), unit (s)
Here the overflow time t = (7200*10000) / 72000000s = 1s
*******************************************************************/
void TIM6_Config(void)
{
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure;
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM6, ENABLE); // Enable TIM6 clock
/* Basic settings */
TIM_TimeBaseStructure.TIM_Period = 10000-1; // Count value 10000
TIM_TimeBaseStructure.TIM_Prescaler = 7200-1; //Pre-division, this value + 1 is the divisor of the division
TIM_TimeBaseStructure.TIM_ClockDivision = 0x0; //Sampling division
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up; //Count up
TIM_TimeBaseInit(TIM6, &TIM_TimeBaseStructure);
TIM_ITConfig(TIM6,TIM_IT_Update, ENABLE); //Enable TIM6 interrupt
TIM_Cmd(TIM6, ENABLE); //Enable timer 6
}
/******************************************************************
** Function name: NVIC_Config
** Function description: Interrupt priority configuration
** Input parameters: None
** Output parameters: None
*****************************************************************/
void NVIC_Config(void)
{
NVIC_InitTypeDef NVIC_InitStructure;
NVIC_PriorityGroupConfig(NVIC_PriorityGroup_2); //Use group 2
NVIC_InitStructure.NVIC_IRQChannel =TIM6_IRQn;//TIM6 interrupt
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;//Preemptive priority is set to 0
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0; //Sub-priority is set to 0
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;//Interrupt enable
NVIC_Init(&NVIC_InitStructure);//Interrupt initialization
}
The interrupt service function is as follows:
/**********************************************************
** Function name: TIM6_IRQHandler
** Function description: Timer 6 update interrupt service routine
** Input parameters: None
** Output parameters: None
**************************************************************/
void TIM6_IRQHandler(void)
{
if (TIM_GetITStatus(TIM6, TIM_IT_Update) != RESET)
{
TIM_ClearITPendingBit(TIM6,TIM_IT_Update);//Clear update interrupt flag
GPIOB->ODR^=GPIO_Pin_0;//Reverse PB0 level
}
}
The usage of TIM7 is similar. The timing interrupt function of the general timer is also used in this way. Just modify TIM6.
Previous article:Internal peripheral resources of different stm32f103 chips
Next article:Register configuration of stm32 IO port mode
Recommended ReadingLatest update time:2024-11-16 12:45
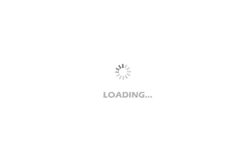
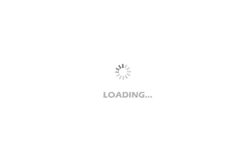
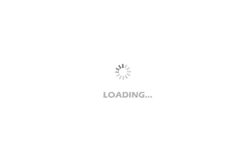
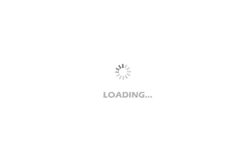
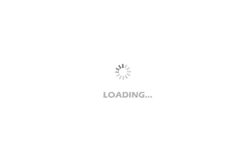
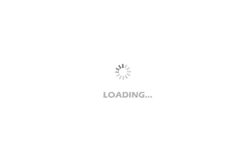
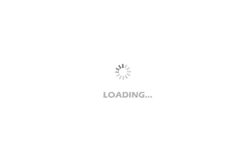
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- EEWORLD University ---- Digi-Key: Accelerated Application of Graph Algorithms in Deep Learning
- Echo Cancellation - How to solve the serious problem of sound swallowing during dual talk in WebRTC AEC algorithm
- A constant and adjustable output power electronic cigarette power supply solution based on TPS61022
- Sales of new energy vehicles have skyrocketed, how should we deal with used batteries?
- E-ON China Online Cloud Sharing Laboratory——"Microcomputer Principles and Applications"
- Improving Power Supply Network Efficiency Using Fixed Ratio Converters
- Large amplitude sine wave signal frequency acquisition circuit
- 【Share】Output adjustable
- [N32L43x Review] 6. Software and Hardware I2C Driver for 0.96-inch OLED
- GD32E231 DIY Competition (2) - Unboxing and Testing