This article uses ADC to convert the voltage value output by the potentiometer, and uses DMA mode to transmit the conversion result. The average value is taken every 8 sampling conversions to perform a simple digital filter.
Detailed configuration and use of ADC
See the previous diary on the use of ADC in STM32 , just add a step to configure DMA at the end:
DMA for ADC channels features configuration
To enable the DMA mode for ADC channels group, use the ADC_DMACmd() function.
To configure the DMA transfer request, use ADC_DMAConfig() function.
DMA Configuration
(摘自STM32F3官方用户手册UM1581 User manual)
1. Enable The DMA controller clock using
RCC_AHBPeriphClockCmd(RCC_AHBPeriph_DMA1, ENABLE) function for DMA1 or using RCC_AHBPeriphClockCmd(RCC_AHBPeriph_DMA2, ENABLE) function for DMA2.
2. Enable and configure the peripheral to be connected to the DMA channel (except for internal SRAM / FLASH memories: no initialization is necessary).
3. For a given Channel, program the Source and Destination addresses, the transfer Direction, the Buffer Size, the Peripheral and Memory Incrementation mode and Data
Size, the Circular or Normal mode, the channel transfer Priority and the Memory-to-Memory transfer mode (if needed) using the DMA_Init() function.
4. Enable the NVIC and the corresponding interrupt(s) using the function
DMA_ITConfig() if you need to use DMA interrupts.
5. Enable the DMA channel using the DMA_Cmd() function.
6. Activate the needed channel Request using PPP_DMACmd() function for any PPP peripheral except internal SRAM and FLASH (ie. SPI, USART ...) The function allowing this operation is provided in each PPP peripheral driver (ie. SPI_DMACmd for SPI peripheral).
7. Optionally, you can configure the number of data to be transferred when the channel
is disabled (ie. after each Transfer Complete event or when a Transfer Error occurs) using the function DMA_SetCurrDataCounter(). And you can get the number of remaining data to be transferred using the function DMA_GetCurrDataCounter() at run time (when the DMA channel is enabled and running).
8. To control DMA events you can use one of the following two methods:
a. Check on DMA channel flags using the function DMA_GetFlagStatus().
b. Use DMA interrupts through the function DMA_ITConfig() at initialization phase and DMA_GetITStatus() function into interrupt routines in communication phase. After checking on a flag you should clear it using DMA_ClearFlag() function. And after checking on an interrupt event you should clear it using DMA_ClearITPendingBit()function.
Important code:
#include "ADC_DMA.h"
#define DATANUM 8
uint8_t FLAG = 0; //Conversion times flag
uint16_t CONV_RESULTS[DATANUM];
void ADC_Config(void)
{
ADC_InitTypeDef ADC_InitStructure;
ADC_CommonInitTypeDef ADC_CommonInitStructure;
RCC_ADCCLKConfig(RCC_ADC12PLLCLK_Div2);
RCC_AHBPeriphClockCmd(RCC_AHBPeriph_ADC12,ENABLE);
//GPIO_Config(); //Configure the sampling input pin to analog input mode
//......other
ADC_CommonInitStructure.ADC_DMAAccessMode = ADC_DMAAccessMode_2;
ADC_CommonInitStructure.ADC_DMAMode = ADC_DMAMode_Circular;
//.......other
ADC_CommonInit(USING_ADC,&ADC_CommonInitStructure);
//ADC Init,ADC_Init(USING_ADC,&ADC_InitStructure);
ADC_ITConfig(USING_ADC, ADC_IT_EOC, ENABLE); //Enable interrupt
ADC_DMAConfig(USING_ADC, ADC_DMAMode_Circular); //Configure ADC_DMA, very important
ADC_DMACmd(USING_ADC, ENABLE);//打开ADC_DMA
DMA_Config();
ADC_Cmd(USING_ADC,ENABLE);
while(!ADC_GetFlagStatus(USING_ADC, ADC_FLAG_RDY));
ADC_StartConversion(USING_ADC);
}
void GPIO_Config(void)
{
//.......
//......
}
void DMA_Config(void)
{
DMA_InitTypeDef DMA_InitStructure;
RCC_AHBPeriphClockCmd(RCC_AHBPeriph_DMA1, ENABLE);
DMA_DeInit(DMA1_Channel1);
DMA_InitStructure.DMA_PeripheralBaseAddr = ADC_DATA_ADDR; //Address of ADC data register DR
DMA_InitStructure.DMA_MemoryBaseAddr = (u32)&CONV_RESULTS; //Address to store conversion results
DMA_InitStructure.DMA_DIR = DMA_DIR_PeripheralSRC;
DMA_InitStructure.DMA_BufferSize = DATANUM;
//.....
DMA_Init(DMA1_Channel1, &DMA_InitStructure);
DMA_Cmd(DMA1_Channel1, ENABLE);
}
void ADC1_2_IRQHandler (void)
{
FLAG++; // Check FLAG in the main function and wake up the process
// Calculate the number of converted data
if(FLAG>DATANUM-1)
{
FLAG=0;
}
ADC_ClearITPendingBit(USING_ADC, ADC_IT_EOC);
}
//Get the average value of the conversion result and do filtering
uint16_t GetAverage(void)
{
u8 i=0;
u16 average=0;
u32 sum=0,voltage=0;
for(i=0;i
{
sum += CONV_RESULTS[i];
}
average = sum/data;
voltage = average*3300/0xFFF;
return voltage;
}
//Abort placement
void NVIC_Config(void)
{
NVIC_InitTypeDef NVIC_InitStruct;
NVIC_InitStruct.NVIC_IRQChannel =ADC1_IRQn;
//........
NVIC_Init(&NVIC_InitStruct);
}
Source code download:
http://download.csdn.net/detail/wind4study/8674381
Previous article:STM32 key scan/key interrupt/external interrupt
Next article:STM32 asymmetric PWM mode realizes dynamic phase shift
Recommended ReadingLatest update time:2024-11-15 18:00
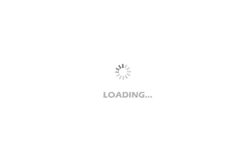
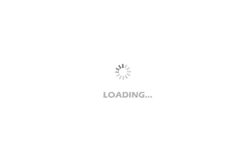
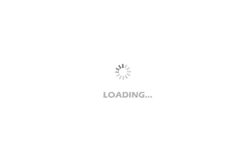
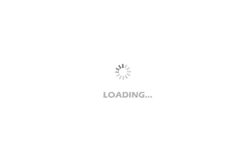
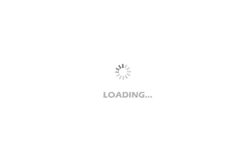
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Keysight Technologies Helps Samsung Electronics Successfully Validate FiRa® 2.0 Safe Distance Measurement Test Case
- Innovation is not limited to Meizhi, Welling will appear at the 2024 China Home Appliance Technology Conference
- Innovation is not limited to Meizhi, Welling will appear at the 2024 China Home Appliance Technology Conference
- Huawei's Strategic Department Director Gai Gang: The cumulative installed base of open source Euler operating system exceeds 10 million sets
- Download from the Internet--ARM Getting Started Notes
- Learn ARM development(22)
- Learn ARM development(21)
- Learn ARM development(20)
- Learn ARM development(19)
- Learn ARM development(14)
- 【Short-term weather forecast system】Basic functions of indoor devices are realized
- [GD32E503 Review] Part 6: FreeRTOS and RTC screen display
- [Synopsys IP Resources] Automotive cybersecurity starts with chips and IP
- ARM MCU and ARM Core
- BMS Rapid Prototyping Platform
- The most basic IO configuration of MSP430
- In AD, if the circle on the surface of the circuit board is not painted with green paint, should a solid circle or a ring be drawn on the TOPSOLDER layer?
- Regarding the question of whether the GND level is stable
- AD19 installation package always reports errors
- Tektronix offers free trials for more than 100 advanced application functions!