Abstract
This article introduces the design of a circular queue data structure to achieve more stable serial port message reception and effectively prevent packet loss. I
have been studying multi-rotor aircraft and other things during this period. I haven't updated my blog. If I don't stick to it, I'm afraid it will be abandoned.
In the previous article, I simply implemented the parsing of the MAVLink protocol, demonstrated the execution of corresponding event processing according to the designed commands, and added CRC verification to achieve more stable communication. However, at the end of the above article, it was mentioned that when a packet is parsed and the corresponding event is processed, new data cannot be received until the event processing is completed and the Msg_Rev.Get state is set to RECEIVING before new data can be received. At this time, when event processing takes a certain amount of time and new data is continuously sent, it is easy to cause data loss.
How to improve the efficiency of serial port communication and avoid packet loss?
In order to improve efficiency, the first thing to think of is to use DMA. However, after consideration, it is found that the received data packets are not fixed; and even if DMA is used, if the MAVLink receive buffer is still designed to receive only one message size, the packet loss problem will still exist.
So I wonder if there is a way to implement it with software. It is like opening a cache space, putting the continuously received data there, and the packet parsing function can take out certain data from it one by one for processing. In this way, as long as the design is reasonable, the packet loss caused by software blocking can be easily solved. So what kind of cache should be designed? In fact, it is easy to think of a queue (first-in-first-out feature), and in order to use space more effectively and reasonably, the data structure of a circular queue will come to mind.
First of all, let's talk about the data structure design and the insertion and deletion operations. I won't say much. The code is as follows:
#define MAX_QUEUE_LEN (4096) // 4K
#define RW_OK 0
#define FULL_ERROR 1
#define EMPTY_ERROR 2
typedef uint8_t boolean;
typedef struct
{
u16 MemFrontSendIndex ;
u16 MemRearRecvIndex ;
u16 MemLength ;
u8 MemDataBuf[MAX_QUEUE_LEN];
} Queue_Mem_Struct , * Queue_Mem_Struct_p ;
Queue_Mem_Struct Queue_Recv ;
boolean QueueMemDataInsert(u8 data)
{
if (MAX_QUEUE_LEN == Queue_Recv.MemLength)
{
return FULL_ERROR;
}
else
{
Queue_Recv.MemDataBuf[Queue_Recv.MemRearRecvIndex] = data;
// if(++Queue_Recv.MemRearRecvIndex >= MAX_QUEUE_LEN){Queue_Recv.MemRearRecvIndex = 0;}
Queue_Recv.MemRearRecvIndex = (Queue_Recv.MemRearRecv Index + 1) % MAX_QUEUE_LEN;
Queue_Recv.MemLength ++ ;
return RW_OK;
}
}
boolean QueueMemDataDel(u8 *data)
{
if (0 == Queue_Recv.MemLength)
{
return EMPTY_ERROR;
}
else
{
*data = Queue_Recv.MemDataBuf[Queue_Recv.MemFrontSendIndex] ;
Queue_Recv.MemFrontSendIndex = (Queue_Recv.MemFrontSendIndex + 1) % MAX_QUEUE_LEN;
Queue_Recv.MemLength -- ;
return RW_OK;
}
}
In this way, the data received by the serial port can be filled into the buffer Queue_Recv.MemDataBuf in sequence through the QueueMemDataInsert function. When processing, the QueueMemDataDel function is called to extract the corresponding number of data for processing. This avoids the problem of packet loss caused by the inability to receive data at the same time during the entire processing process. Of course, at this time, it is necessary to ensure that the data in the buffer is processed in time, otherwise, especially when the amount of data is large, after the queue is filled, it will cause the data to be unable to be filled in.
In addition, it will be found that the above design can be combined with the DMA method. If the design is good, the STM32 utilization and system operation efficiency can be further greatly improved!
Previous article:Summary of stm32 serial communication debugging
Next article:STM32 network communication DM9000A circuit design
Recommended ReadingLatest update time:2024-11-16 15:36
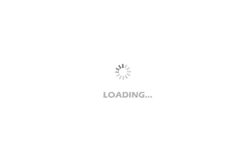
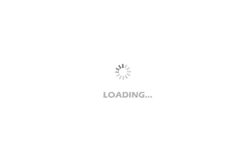
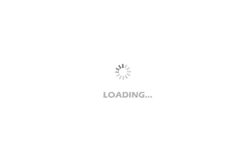
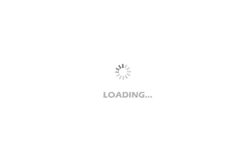
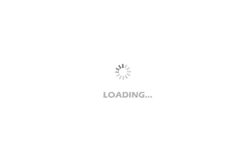
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- PCB package design of electronic devices
- 【RT-Thread Reading Notes】9. RT-Thread Study Chapters 14-16 Reading Notes
- Why are there Flash and EEPROM in microcontrollers?
- TI official website millimeter wave radar application demonstration
- Looking for a displacement sensor, impact sensor, acceleration sensor and GPS kit to assemble into a system
- Industrial Automation Equipment Recruitment
- C5000 ringtone keeps bugging
- Has anyone tried driving Zhengyuan Motor's coreless DC brushless motor (AM-BL2453A/B series) with STM32?
- News: Qorvo, a well-known RF chip company, also makes power solutions
- Why "It's time to abandon the switch matrix for multi-port testing" is all in Keysight's white paper ~ Limited time gifts are waiting for you