Concept
In the STM32 reference manual, the serial port is described as a universal synchronous asynchronous receiver and transmitter (USART), which provides a flexible method for full-duplex data exchange with external devices using the industry standard NRZ asynchronous serial data format. (Okay, I don't quite understand it, so I'll just post the official definition for now, and you can figure it out yourself)
Configuration steps
Turn on the clock (RCC configuration).
Since the TX, RX and AFIO of UART are all hung on the APB2 bridge, the firmware library function RCC_APB2PeriphClockCmd() is used for initialization. UARTx needs to be discussed separately. If it is UART1, it is hung on the APB2 bridge, so RCC_APB2PeriphClockCmd() is used for initialization. The rest of UART2~5 are hung on APB1.GPIO configuration
The properties of GPIO are included in the structure GPIO_InitTypeDef, where for the TX pin, the GPIO_Mode field is set to GPIO_Mode_AF_PP (multiplexed push-pull output), and the GPIO_Speed switching rate is set to GPIO_Speed_50MHz; for the RX pin, the GPIO_Mode field is set to GPIO_Mode_IN_FLOATING (floating input), and the switching rate does not need to be set. Finally, enable the IO port through GPIO_Init().When NVIC configures
STM32, even if there is only one interrupt, it still needs to configure the priority level, which is used to enable the trigger channel of a certain interrupt. STM32 interrupts have at most two levels, namely preemption priority (main priority) and sub-priority (slave priority), and the length of the entire priority setting parameter is 4 bits, so it is necessary to first divide the preemption priority bit and sub-priority bit, which is implemented through NVIC_PriorityGroupConfig();The interrupt priority NVIC property of a specific device is contained in the structure NVIC_InitTypeDef, where the field NVIC_IRQChannel contains the interrupt vector of the device, which is saved in the startup code; the field NVIC_IRQChannelPreemptionPriority is the primary priority NVIC_IRQChannelSubPriority is the secondary priority, and the value range should be determined according to the bit division; the last NVIC_IRQChannelCmd field is whether it is enabled, which is generally set to ENABLE. Finally, NVIC_Init() is used to enable this interrupt vector.
The USART configuration
is determined by the structure USART_InitTypeDef. The fields in UART mode are as follows:USART_BaudRate: Baud rate (data bits that can be transmitted per second), the default value is 9600.
USART_WordLength: word length
USART_StopBits: Stop bits
USART_Parity: Check mode (parity check)
USART_HardwareFlowControl: Hardware flow control
USART_Mode: Single/duplex, i.e., transmit/receive status.
Finally, set it up through USART_Init().
Code Summary
void uart_init(u32 bound){ GPIO_InitTypeDef GPIO_InitStructure; USART_InitTypeDef USART_InitStructure; NVIC_InitTypeDef NVIC_InitStructure; RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1|RCC_APB2Periph_GPIOA, ENABLE); //Enable USART1 and GPIOA clocks USART_DeInit(USART1); //Reset serial port 1 (set all parameters to default values) //USART1_TX (send data) PA.9 pin GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9; GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz; GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP; //Multiplex push-pull output GPIO_Init(GPIOA, &GPIO_InitStructure); //初始化PA.9 //USART1_RX (receive data) PA.10 pin GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10; GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING; //Floating input GPIO_Init(GPIOA, &GPIO_InitStructure); //初始化PA.10 //NVIC interrupt vector configuration NVIC_InitStructure.NVIC_IRQChannel = USART1_IRQn; NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority=3 ; //Set the preemption priority to 3 NVIC_InitStructure.NVIC_IRQChannelSubPriority = 3; //The sub-priority is set to 3. The priority is determined according to the importance of different interrupts. NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE; //IRQ channel enable NVIC_Init(&NVIC_InitStructure); //Initialize NVIC registers according to the parameters set above //USART initialization settings USART_InitStructure.USART_BaudRate = bound; //Baud rate is 9600; USART_InitStructure.USART_WordLength = USART_WordLength_8b; //Word length is 8 bits USART_InitStructure.USART_StopBits = USART_StopBits_1; //1 stop bit USART_InitStructure.USART_Parity = USART_Parity_No; //No parity bit USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None; //No hardware data flow control USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx; //Transmit and receive mode USART_Init(USART1, &USART_InitStructure); //Serial port initialization USART_ITConfig(USART1, USART_IT_RXNE, ENABLE); //Interrupt enable USART_Cmd(USART1, ENABLE); //Serial port enable}123456789101112131415161718192021222324252627282930313233343536373839
end
Previous article:STM32 GPIO register introduction and settings
Next article:STM32 RCC study notes
Recommended ReadingLatest update time:2024-11-16 16:03
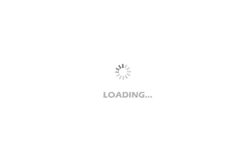
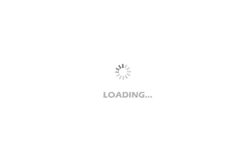
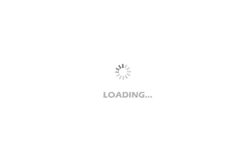
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- [Recruitment] Huawei HiSilicon Semiconductor recruits chip R&D positions
- 【ufun learning】Part 2: Initial test demo example
- Problems with temperature acquisition based on stm32f446re
- MCU+MOS tube stepping subdivision drive control solution
- Do you know A2B, the automotive audio bus technology?
- Detailed explanation of MSP430F5529 system working mode
- SensorTile.Box becomes bricked after firmware upgrade
- Window Comparator
- [GD32L233C-START Review] Part 2 Compilation, Software Development Environment Review
- Stuck inexplicably