I believe that those who have played with 51 should have played with DS18B20. Although it is used on ARM11, the operation is still the same. One more thing is that you need to switch the IO direction before reading and writing IO. In fact, 51 needs to be switched to a high level before reading, which is generally done by the compiler.
/****************************************************** *************************************************** **********
* File name: ds18b20.c
* Function: S3C6410 DS18B20 driver
* Author: cp1300@139.com
* Created: September 17, 2012 22:45
* Last modified: September 17, 2012
* Details: Delay function support is required
* Make sure the delay accuracy is as high as possible
* Do not interrupt the reading process for a long time, because 1wire has strict timing requirements.
*************************************************** *************************************************** *********/
#include "system.h"
#include "timer.h"
#include "delay.h"
#include "ds18b20.h"
//DS18B20 uses GPIOE0
#define Set18b20IOout() (rGPECON |= 1) //Set DS18B20 IO to output,
#define Set18b20IOin() (rGPECON &= (~0xf)) //Set DS18B20 IO to floating input,
#define Read18b20IO() ((rGPEDAT & BIT0) ? 1 : 0) //Read DS18B20 IO
#define Write18b20IO(x) (x ? (rGPEDAT |= BIT0) : (rGPEDAT &= ~BIT0)) //Write DS18B20 IO
/****************************************************** *************************************************** ************************
*Function: u8 DS18B20_Reset(void)
*Function: Reset DS18B20
*Parameters: None
*Return: 0: success; 1: failure
*Depends on: underlying macro definitions
*Author : cp1300@139.com
*Time: 20120917
*Last modified: 20120917
* Description: None
*************************************************** *************************************************** *********************/
u8 DS18B20_Reset(void)
{
u8 i = 0;
Set18b20IOout(); //Host port push-pull output mode
Write18b20IO(1);
Delay_US(1);
Write18b20IO(0); //Pull the bus low for 480us~240us
Delay_US(500); //>480US delay
Write18b20IO(1);
Delay_US(2); //Reset completed
Set18b20IOin(); //Host port floating input mode
while(Read18b20IO()) //Wait for low level response signal
{
i++;
Delay_US(1);
if(i > 100)
return 1; //Waiting timeout, initialization failed, return 1;
}
Delay_US(250); //Skip reply signal
return 0x00; //DS18B20 detected and initialized successfully
}
/****************************************************** *************************************************** ************************
*Function: u8 DS18B20_ReadData(void)
*Function: Read DS18B20 data
*Parameters: None
*Return: data
*Depends on: underlying macro definitions
*Author : cp1300@139.com
*Time: 20120917
*Last modified: 20120917
* Description: None
*************************************************** *************************************************** *********************/
u8 DS18B20_ReadData(void)
{
u8 i,data = 0;
for(i = 0;i < 8;i ++)
{
Set18b20IOout(); //Host port push-pull output mode
Write18b20IO(0); //Pull the bus low for 10-15us
data >>= 1;
Delay_US(12);
Write18b20IO(1); //Release the bus
Set18b20IOin(); //Host port floating input mode
Delay_US(1);
if(Read18b20IO()) //Read data, about 40-45us delay after reading
data |= 0x80;
Delay_US(42);
}
return data;
}
/****************************************************** *************************************************** ************************
*Function: void DS18B20_WriteData(u8 data)
*Function: Write data to DS18B20
*Parameter: data
*Return: None
*Depends on: underlying macro definitions
*Author : cp1300@139.com
*Time: 20120917
*Last modified: 20120917
* Description: None
*************************************************** *************************************************** *********************/
void DS18B20_WriteData(u8 data)
{
u8 i;
Set18b20IOout(); //Host port push-pull output mode
for(i = 0;i < 8;i ++)
{
Write18b20IO(0); //Pull the bus low for 10-15us
Delay_US(12);
Write18b20IO(data & 0x01); //Write data bit, keep 20-45us
Delay_US(30);
Write18b20IO(1); //Release the bus
data >>= 1;
Delay_US(2);
}
}
/****************************************************** *************************************************** ************************
*Function: s16 DS18B20_ReadTemper(void)
*Function: Read DS18B20 temperature
*Parameters: None
*Return: Temperature value
*Depends on: underlying macro definitions
*Author : cp1300@139.com
*Time: 20120917
*Last modified: 20120917
* Note: The temperature value is expanded 100 times, and the temperature value is a signed number.
*************************************************** *************************************************** *********************/
s16 DS18B20_ReadTemper(void)
{
u8th, tl;
s16 data;
if(DS18B20_Reset())
{
return 0xffff; //return error
}
DS18B20_WriteData(0xcc); //Skip reading serial number
DS18B20_WriteData(0x44); //Start temperature conversion
DS18B20_Reset();
DS18B20_WriteData(0xcc); //Skip reading serial number
DS18B20_WriteData(0xbe); //Read temperature
tl = DS18B20_ReadData(); //Read the lower eight bits
th = DS18B20_ReadData(); //Read the upper eight bits
data = th;
data <<= 8;
data |= tl;
data *= 6.25; //Expand the temperature value 100 times, accurate to 2 decimal places
return data;
}
#ifndef DS18B20_H_
#define DS18B20_H_
s16 DS18B20_ReadTemper(void); //Read DS18B20 temperature
#endif /*DS18B20_H_*/
DELAY.C
/****************************************************** *************************************************** **********
* File name: delay.c
* Function: Delay function, call timer 1 for delay
* Author: cp1300@139.com
* Created: September 17, 2012 22:32
* Last modified: September 17, 2012
* Details: Delay error 1-2us, using timer 1
* Cannot be used in interrupt service routines
*************************************************** *************************************************** *********/
#include "system.h"
#include "timer.h"
#include "delay.h"
/****************************************************** *************************************************** ************************
*Function: void Delay_US(u32 Delay)
*Function: US delay
*Parameter: Delay: delay time, unit US
*Return: None
*Depends on: underlying macro definitions
*Author : cp1300@139.com
*Time: 20120917
*Last modified: 20120917
* Note: If timer 0 is called for delay, then timer 0 cannot be used, and the delay error is 1US
*************************************************** *************************************************** *********************/
void Delay_US(u32 Delay)
{
rTCFG0 |= 65; //Timer 0 pre-divided by 65+1, PCLK=66 provides clock, 66 division generates 1MHz timer clock
rTCON &= (~0xff); //Clear settings
rTCON |= BIT3; //Timer 0 automatic update enable
rTCNTB0 = Delay; //Reload value
rTINT_CSTAT |= BIT5; //Clear interrupt flag
rTINT_CSTAT &= ~BIT0; //Disable timer 0 interrupt
//The following operation starts timer 0
rTCON |= BIT1; //manual update
rTCON &= ~BIT1; //End manual update
rTCON |= BIT0; //Start timer 0
while(!(rTINT_CSTAT & BIT5)); //Wait for the delay to arrive
rTINT_CSTAT |= BIT5; //Clear interrupt flag
}
/****************************************************** *************************************************** ************************
*Function: void Delay_MS(u32 Delay)
*Function: MS delay
*Parameter: Delay: delay time, unit US
*Return: None
*Depends on: underlying macro definitions
*Author : cp1300@139.com
*Time : 20120918
*Last modified: 20120918
* Note: If timer 0 is called for delay, then timer 0 cannot be used, and the delay error is <1ms
*************************************************** *************************************************** *********************/
void Delay_MS(u32 Delay)
{
while(Delay --)
{
Delay_US(1000);
}
}
Later, this program will be ported to LINUX as a DS18B20 driver.
Previous article:S3C6410 Bare Metal Hardware JPEG Decoding
Next article:S3C6410 bare metal resistive screen driver
Recommended ReadingLatest update time:2024-11-16 15:19
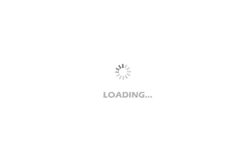
- Popular Resources
- Popular amplifiers
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Arduino and LabVIEW Development Practice (Shen Jinxin)
-
51 MCU application development from entry to mastery
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- How to distinguish between pads and vias_Differences between vias and pads
- Could you please tell me what circuit is generally used to implement the 0/4-20mA drive circuit?
- Principle and application of diaphragm pressure gauge
- Showing off your products (6) - silicon lab
- EEWORLD University - How to draw a high-end PCB ruler with Altium20
- Agitek case sharing - Metrology and testing demonstration case of automotive electronic modules
- How to turn off MPLAB XIDE's code optimization function
- IoT Smart Parking Solutions
- EPWM1 A and B pulses are not complementary
- Find the parameters of the low-pass filter