This example will implement the key scanning function of stm32.
Operation Register
When the I/O port of stm32 is used as input, the status of the I/O port is read by reading the contents of the GPIOx -> IDR register.
The description of each bit of the IDR register is as follows:
Since systick cannot generate interrupts as easily as library functions, it is inconvenient to query the status of each pin after querying the systick status bit. Therefore, unlike the library function method, the pin status is directly queried to detect key presses.
The PAout(x), PAin(x) and other functions are called in the sys.h file. See: (The sys.h code refers to the stm32 direct operation register development environment configuration )
Direct operation register code:
#include#include "system.h" //Key button port definition #define key0 PAin(0) // PA0 #define key1 PAin(1) // PA1 #define key2 PAin(2) // PA2 #define key3 PAin(3) // PA3 //LED button port definition #define LED0 PAout(4) // PA4 #define LED1 PAout(5) // PA5 #define LED2 PAout(6) // PA6 #define LED3 PAout(7) // PA7 void Gpio_Init(void);//initialization function void Key_Scan(void); int main(void) { Rcc_Init(9); //System clock settings Gpio_Init(); //Initialize the hardware interface connected to the LED while(1) { Key_Scan(); } } void Key_Scan(void) { if(key0 == 0 || key1 == 0 || key2 == 0 || key3 == 0) //if(GPIOA->IDR != 0x000F) { delay(10000); //debounce if(key0 == 0) { while(key0 == 0); //Detect key release LED0 = !LED0; } if(key1 == 0) { while(key1 == 0); LED1 = !LED1; } if(key2 == 0) { while(key2 == 0); LED2 = !LED2; } if(key3 == 0) { while(key3 == 0); LED3 = !LED3; } } } void Gpio_Init(void) { RCC->APB2ENR|=1<<2; //Enable PORTA clock GPIOA->CRL&=0X0000FFFF; // PA0~3 are set as floating input, PA4~7 are set as push-pull output GPIOA->CRL|=0X33334444; }
Library function operation
Anyone who has studied EDA should know a concept called a state machine. After a certain condition is triggered, it enters another state. If another condition is triggered, it enters the next state. If the condition is not met, it enters the initial state, or does not change the state. This is roughly the idea of implementing key scanning.
Systick generates a 20ms timing and checks in the interruption whether the buttons of each pin are pressed. If a button is pressed, it enters state 1.
If it is pressed, determine whether it is jittering. If it is, return to state 0. If not, determine which pin button is pressed, and enter state 2 after implementing the corresponding function.
In state 2, check whether the key is released. If it is released, return to state 0, otherwise the state does not change.
The code is as follows: main.c
#include "stm32f10x.h" #define KEYPORT GPIOA #define KEY0 GPIO_Pin_3 #define KEY1 GPIO_Pin_1 #define KEY2 GPIO_Pin_2 #define KEY3 GPIO_Pin_0 typedef enum { KeyScanState_0 = 0x00, KeyScanState_1 = 0x01, KeyScanState_2 = 0x02, }KeyScanState_Typedef; KeyScanState_Typedef KeyScanState; void RCC_Configuration(void); void GPIO_Configuration(void); void SysTick_Set(vu32 x); int main(void) { RCC_Configuration(); GPIO_Configuration(); SysTick_Set(20000); while(1); } void SysTick_Handler(void) { vu16 keyState; keyState = GPIO_ReadInputData(KEYPORT) & 0x000f; switch(KeyScanState) { case KeyScanState_0: { if(keyState != 0x000f) { KeyScanState = KeyScanState_1; } break; } case KeyScanState_1: { if(keyState != 0x000f) { if(GPIO_ReadInputDataBit(KEYPORT,KEY0) == 0) { GPIO_WriteBit(GPIOA,GPIO_Pin_4,(BitAction)(1-GPIO_ReadOutputDataBit(GPIOA,GPIO_Pin_4))); }else if(GPIO_ReadInputDataBit(KEYPORT,KEY1) == 0) { GPIO_WriteBit(GPIOA,GPIO_Pin_5,(BitAction)(1-GPIO_ReadOutputDataBit(GPIOA,GPIO_Pin_5))); }else if(GPIO_ReadInputDataBit(KEYPORT,KEY2) == 0) { GPIO_WriteBit(GPIOA,GPIO_Pin_6,(BitAction)(1-GPIO_ReadOutputDataBit(GPIOA,GPIO_Pin_6))); }else if(GPIO_ReadInputDataBit(KEYPORT,KEY3) == 0) { GPIO_WriteBit(GPIOA,GPIO_Pin_7,(BitAction)(1-GPIO_ReadOutputDataBit(GPIOA,GPIO_Pin_7))); } KeyScanState = KeyScanState_2; }else{ KeyScanState = KeyScanState_0; } break; } case KeyScanState_2: { if(keyState == 0x000f) { KeyScanState = KeyScanState_0; } break; } } } void SysTick_Set(vu32 x) { if(SysTick_Config(x*72)) //Configuration error returns 1, max 16777216 { GPIO_SetBits(GPIOA, GPIO_Pin_7); //Error handling } } void GPIO_Configuration(void) { GPIO_InitTypeDef GPIO_InitStructure; GPIO_InitStructure.GPIO_Pin = GPIO_Pin_4 | GPIO_Pin_5 | GPIO_Pin_6 | GPIO_Pin_7; GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz; GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP; GPIO_Init(GPIOA, &GPIO_InitStructure); GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0 | GPIO_Pin_1 | GPIO_Pin_2 | GPIO_Pin_3; GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING; GPIO_Init(GPIOA, &GPIO_InitStructure); } void RCC_Configuration(void) { /* Define enumeration type variable HSEStartUpStatus */ ErrorStatus HSEStartUpStatus; /* Reset system clock settings */ RCC_DeInit(); /* Enable HSE*/ RCC_HSEConfig(RCC_HSE_ON); /* Wait for HSE to start oscillating and stabilize*/ HSEStartUpStatus = RCC_WaitForHSEStartUp(); /* Determine whether the HSE is successfully started, if yes, enter if() */ if(HSESTartUpStatus == SUCCESS) { /* Select HCLK (AHB) clock source as SYSCLK divided by 1 */ RCC_HCLKConfig(RCC_SYSCLK_Div1); /* Select PCLK2 clock source as HCLK (AHB) divided by 1 */ RCC_PCLK2Config(RCC_HCLK_Div1); /* Select PCLK1 clock source as HCLK (AHB) divided by 2 */ RCC_PCLK1Config(RCC_HCLK_Div2); /* Set the FLASH delay cycle number to 2 */ FLASH_SetLatency(FLASH_Latency_2); /* Enable FLASH prefetch cache */ FLASH_PrefetchBufferCmd(FLASH_PrefetchBuffer_Enable); /* Select the phase-locked loop (PLL) clock source as HSE 1 division, the multiplier is 9, then the PLL output frequency is 8MHz * 9 = 72MHz */ RCC_PLLConfig(RCC_PLLSource_HSE_Div1, RCC_PLLMul_9); /* Enable PLL */ RCC_PLLCmd(ENABLE); /* Wait for PLL output to stabilize */ while(RCC_GetFlagStatus(RCC_FLAG_PLLRDY) == RESET); /* Select SYSCLK clock source as PLL */ RCC_SYSCLKConfig(RCC_SYSCLKSource_PLLCLK); /* Wait for PLL to become the SYSCLK clock source */ while(RCC_GetSYSCLKSource() != 0x08); } /* Turn on the GPIOA clock on the APB2 bus */ RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE); //RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM2, ENABLE); }
In this example, the Systick interrupt processing function is moved from stm32f10x_it.c to main.c, avoiding the need to declare external variables in stm32f10x_it.c.
Previous article:stm32 i2c communication [operation register + library function]
Next article:The most convenient timer Systick on stm32 [operation register + library function]
Recommended ReadingLatest update time:2024-11-16 15:49
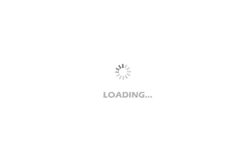
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- TouchGFX design——by supermiao123
- EEWORLD University Hall----Overview of THS6222 Broadband PLC Line Driver
- Optimization and Implementation Method of Task Scheduling in μC/OS
- Does anyone know what model this monster is?
- MSP430 MCU Development Record (6)
- Using ESP's rtc memory in mpy
- Linux serial communication 1——96-N-8-1 format
- [STM32WB55 Review] Summary
- I need help with the simplest program to control 12864 with keys
- TUSB9261 -- USB3.0 to SATA interface bridge chip programming guide