LPC2131 UART [Query method] Operation flow: Initialize baud rate, line control register -> Query line status register -> Read data
-> Process error status -> Send data
LPC2131 UART initialization operation flow
1. Set the uart line control register U0LCR and set bit7 to 1 to enable writing to the U0DLM U0DLL register
2. Set the baud rate. The baud rate register value should be Fpclk / (16*baud). The upper 8 bits are written to U0DLM and the lower 8 bits are written to U0DLL.
3. Set the uart line control register U0LCR, set bit7 to 0, disable writing to U0DLM and U0DLL, and enable writing to the rest of U0RBR
4. Set the uart line control register U0LCR to set the character length, stop bit, parity check
U0LCR Description
Bit
1:0 Word length selection 00: 5 bits 01: 6 bits 10: 7 bits 11: 8 bits
2 Stop bit
3 Parity enable
5:4 Parity selection 00: Odd parity 01: Even parity
6 Interval control
7 Divisor latch access 0: Disable access to the divisor latch 1: Enable access to the divisor latch
After initialization is completed, you can send and receive characters.
By reading the UART line status register U0LSR, the status of UART transmission and reception can be obtained.
U0LSR Description
Bit
0 Receive Data Ready
1 Overrun Error
2 Parity Error
3 True Error
4 Break Error
5 Transmit Holding Register Empty
6 Transmitter Empty
7 FIFO Error
Read and write UART send hold, receive buffer register can send and receive data
. U0RBR is the highest byte of UART Rx FIFO, contains the earliest received character, which can be read out through the bus interface. If the received character is less than 8 bits, the unused MSB is filled with 0.
By setting the FIFO control register U0FCR, you can set the number of characters in the receive buffer FIFO. If it is full of 8 characters, an interrupt request will be issued.
LPC2131 UART [interrupt mode]
Based on the above query initialization, if the U0FCR FIFO control register and the U0IER interrupt enable register are set, the UART interrupt mode can be enabled. The interrupt is mainly generated in the following three situations:
1. The data in FIFO reaches the trigger point. The trigger point can be selected as 1, 4, 8, or 14 characters.
2. FIFO timeout. After all, the data may not be "complete". When the FIFO does not reach the trigger point and exceeds the delay time, half of the delay time is 4-5 times the sending time, a timeout interrupt will be generated. At this time, the incomplete data in the FIFO can be read. Note that if the trigger point of the FIFO is set to 8 characters, there are 4 characters in the current FIFO, the sending is suspended, and a timeout interrupt is generated, then 4 times will be generated. After each interrupt, one character is read out, and the remaining characters wait in the FIFO, and an interrupt is generated again, and so on.
3. Data errors, including parity error, frame error, overflow error, etc., can be queried in UxLSR
Interrupt priority is 3 > 2 = 1 > THRE
There is another type of interrupt - THRE interrupt, which will be discussed later.
A typical initialization
A typical initialization
uart0_init(mode, 115200); // Initialize UART, DLAB = 0
U0FCR = 0x81; // Enable UART FIFO mode Trigger point 8 characters
U0IER = 0x01; // Enable RBR interrupt and disable THRE Rx line status interrupt (when DLAB=0)
VICIntSelect = 0; // Select all interrupts as IRQ interrupts
VICVectCntl0 = 0x20 | 0x06; // slot0 corresponding interrupt source is UART
VICVectAddr0 = (uint32)uart_int; // slot0 interrupt service routine
VICIntEnable = 1 << 0x06; // Enable UART interrupt source
IRQEnable(); // Enable target board IRQ interrupt
A typical interrupt handling routine
void __irq uart_int()
...{
uint8 i;
uart0_send_str("Interrupt! ");
if ((U0IIR & 0x0F) == 0x04) ...{ // If the interrupt is caused by FIFO data reaching the trigger point
rcv_new = 1;
for (i=0; i<8; i++) ...{
buffer[i] = U0RBR;
}
} else if ((U0IIR & 0x0F) == 0x0C) ...{ // If the interrupt was caused by a FIFO timeout
buffer[0] = U0RBR;
uart0_send_byte(buffer[0]);
}
VICVectAddr = 0; // Clear interrupt flag, no need to reset EXTINT if it is not an external interrupt
Previous article:STM32 bit operation method
Next article:The baud rate of the newly created UART0 communication is inconsistent (the baud rate is reduced by 4 times)
Recommended ReadingLatest update time:2024-11-23 02:39
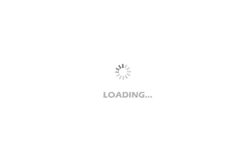
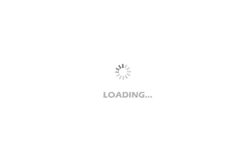
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- CANBus series varistors specifically designed for automotive ESD protection
- 24GHz human detection radar
- A complete guide to the design of electronic control for sensorless brushless DC motors
- Integrated circuit detection method
- Gizwits ESP8266 controls the lifting of rolling shutter doors
- How to connect unused op amps in the same package?
- Help analyze this small wind power generation circuit
- Lessons learned from transplanting LwIP on C6414
- Hall sensor applied to brushless motor drive control
- Using Power Management