The GPIO_Init function is the initialization function of the IO pin. It performs the initialization configuration of each pin. It mainly accepts two parameters, one is the configuration pin group (GPIO_TypeDef* GPIOx), and the other is the configuration parameter (GPIO_InitTypeDef* GPIO_InitStruct), as follows
void GPIO_Init(GPIO_TypeDef* GPIOx, GPIO_InitTypeDef* GPIO_InitStruct)
/*The first parameter is the group of pins. Each group has 16 pins, and each group has a different register configuration address. The second parameter is a data structure, which is where the basic configuration information is placed, and then the structure is passed into the function for configuration*/
//The data structure can be expressed as follows
typedef struct
{
uint16_t GPIO_Pin; //pin number
GPIOSpeed_TypeDef GPIO_Speed; //Configure speed
GPIOMode_TypeDef GPIO_Mode; //Working mode
}GPIO_InitTypeDef;
//The configuration mode and working mode are enumeration variables of GPIOSpeed_TypeDef and GPIOMode_TypeDef
In order to analyze this function conveniently, we need to list the definitions of several constants.
//First is the pin definition
#define GPIO_Pin_0 ((uint16_t)0x0001) /* Pin 0 selected */
#define GPIO_Pin_1 ((uint16_t)0x0002) /* Pin 1 selected */
#define GPIO_Pin_2 ((uint16_t)0x0004) /* Pin 2 selected */
#define GPIO_Pin_3 ((uint16_t)0x0008) /* Pin 3 selected */
#define GPIO_Pin_4 ((uint16_t)0x0010) /* Pin 4 selected */
#define GPIO_Pin_5 ((uint16_t)0x0020) /* Pin 5 selected */
#define GPIO_Pin_6 ((uint16_t)0x0040) /* Pin 6 selected */
#define GPIO_Pin_7 ((uint16_t)0x0080) /* Pin 7 selected */
#define GPIO_Pin_8 ((uint16_t)0x0100) /* Pin 8 selected */
#define GPIO_Pin_9 ((uint16_t)0x0200) /* Pin 9 selected */
#define GPIO_Pin_10 ((uint16_t)0x0400) /* Pin 10 selected */
#define GPIO_Pin_11 ((uint16_t)0x0800) /* Pin 11 selected */
#define GPIO_Pin_12 ((uint16_t)0x1000) /* Pin 12 selected */
#define GPIO_Pin_13 ((uint16_t)0x2000) /* Pin 13 selected */
#define GPIO_Pin_14 ((uint16_t)0x4000) /* Pin 14 selected */
#define GPIO_Pin_15 ((uint16_t)0x8000) /* Pin 15 selected */
#define GPIO_Pin_All ((uint16_t)0xFFFF) /* All pins selected */
// Followed by the mode definition
typedef enum
{ GPIO_Mode_AIN = 0x0, //Analog input
GPIO_Mode_IN_FLOATING = 0x04, //Floating input mode, default
GPIO_Mode_IPD = 0x28, //Pull-up/pull-down input mode
GPIO_Mode_IPU = 0x48, //Reserved
GPIO_Mode_Out_OD = 0x14, //General purpose open drain output
GPIO_Mode_Out_PP = 0x10, //General push-pull output
GPIO_Mode_AF_OD = 0x1C, //Multiplexed (open drain) output
GPIO_Mode_AF_PP = 0x18 //Multiplexed (push-pull) output
}GPIOMode_TypeDef;
//Finally, the speed definition
typedef enum
{
GPIO_Speed_10MHz = 1,
GPIO_Speed_2MHz,
GPIO_Speed_50MHz
}GPIOSpeed_TypeDef;
It is easy to see from the pin definition that if pin 0 is 1, the 0th position of the 16 bits is 1, if pin 2 is 1, and so on. Therefore, if you want to define multiple pins, you only need to use the OR logic operation (|).
Secondly, the mode definition also has its rules. Referring to the "stmf10xxx Reference Manual", it can be concluded that every 4 bits in the high and low configuration registers store a mode + speed, where the mode occupies the high 2 bits and the speed occupies the low 2 bits. 16 pins have 4*16=64 bits to store, so this definition makes sense. The reason is that the mode occupies the high bit. For example, 10 represents the pull-up/pull-down output mode, which occupies the high 2 bits in the 4 bits, which should be 1000. The low 2 bits are first represented by 00, so the pull-up/pull-down output mode can be represented as 1000=0x8, or it can be represented by the fourth bit of 0x28. The same is true for the others, that is, the fifth bit 1 represents the output mode and 0 represents the input mode.
The speed is directly represented by 1, 2, and 3.
The specific function analysis is as follows
void GPIO_Init(GPIO_TypeDef* GPIOx, GPIO_InitTypeDef* GPIO_InitStruct)
{
/* Initialize each variable */
uint32_t currentmode = 0x00, currentpin = 0x00, pinpos = 0x00, pos = 0x00;
uint32_t tmpreg = 0x00, pinmask = 0x00;
//currentmode is used to store temporary LCIR
//currentpin is used to store the configured pin position
//pinpos is used to store the pin number of the current operation
//pos stores the pin position of the current operation
//tmreg current CIR
//pinmask
//Judge the parameters
assert_param(IS_GPIO_ALL_PERIPH(GPIOx));
assert_param(IS_GPIO_MODE(GPIO_InitStruct->GPIO_Mode));
assert_param(IS_GPIO_PIN(GPIO_InitStruct->GPIO_Pin));
// Take out the mode information in the configuration information and take its lower 4 bits
currentmode = ((uint32_t)GPIO_InitStruct->GPIO_Mode) & ((uint32_t)0x0F);
if ((((uint32_t)GPIO_OInitStruct->GPIO_Mode) & ((uint32_t)0x10)) != 0x00) //Output mode
{
//Judge the parameters
assert_param(IS_GPIO_SPEED(GPIO_InitStruct->GPIO_Speed));
//Put the speed information into the lower two bits of currentmode
currentmode |= (uint32_t)GPIO_InitStruct->GPIO_Speed;
}
if (((uint32_t)GPIO_InitStruct->GPIO_Pin & ((uint32_t)0x00FF)) != 0x00) //The pin is defined
{
//Save the current CRL
tmpreg = GPIOx->CRL;
//Loop the lower eight pins
for (pinpos = 0x00; pinpos < 0x08; pinpos++)
{
//The current pin is at position 1
pos = ((uint32_t)0x01) << pinpos;
//Read the current pin in the pin information
currentpin = (GPIO_InitStruct->GPIO_Pin) & pos;
if (currentpin == pos) //If the current pin exists in the configuration information
{
pos = pinpos << 2; //pos = pin number x2
pinmask = ((uint32_t)0x0F) << pos; //1111<
tmpreg &= ~pinmask; //The CRL bit that should be operated currently is cleared to 0
tmpreg |= (currentmode << pos); //Set the CRL bit of the current operation
if (GPIO_InitStruct->GPIO_Mode == GPIO_Mode_IPD) // Set the port to high level
{
GPIOx->BRR = (((uint32_t)0x01) << pinpos);
}
else
{
if (GPIO_InitStruct->GPIO_Mode == GPIO_Mode_IPU) // Port cleared
{
GPIOx->BSRR = (((uint32_t)0x01) << pinpos);
}
}
}
}
GPIOx->CRL = tmpreg;
}
The last step is to transfer the configured CRL to the CRL register.
Previous article:STM32 USART configuration
Next article:STM32 input and output summary
Recommended ReadingLatest update time:2024-11-16 20:24
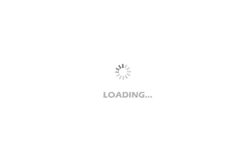
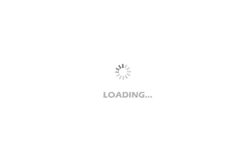
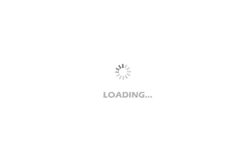
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- 06 Make GD32L233C expansion board
- The names, wavelengths, characteristics and application fields of each band in the electromagnetic spectrum
- [TI Course] How is the anti-interference ability of TI millimeter-wave radar?
- The ampere-second product of capacitance and the volt-second product of inductance
- Regarding the distinction between NMOS tubes and PMOS tubes
- Pins 2 and 4 are missing from the schematic and package of the BNX016-01 device
- SIMetrix-SIMPLIS ~1~
- What is the difference between a vector signal source and an RF signal source?
- I don't have enough download points. Is there any good way to earn points?
- Commonly used algorithms for drones - Kalman filter (VIII)