1 Basic Concepts
The ARM Coetex-M3 core supports a total of 256 interrupts, including 16 internal interrupts, 240 external interrupts and a programmable 256-level interrupt priority setting. The STM32 currently supports a total of 84 interrupts (16 internal + 68 external), as well as a 16-level programmable interrupt priority setting, using only the upper 4 bits of the 8-bit interrupt priority setting.
STM32 can support 68 interrupt channels, which have been fixedly assigned to corresponding external devices. Each interrupt channel has its own interrupt priority control byte PRI_n (8 bits, but only 4 bits are used in STM32, and the upper 4 bits are valid). The 8-bit interrupt priority control word of each 4 channels constitutes a 32-bit priority register. The priority control words of 68 channels constitute at least 17 32-bit priority registers.
The 4-bit interrupt priority can be divided into 2 groups. From the high position, the front is defined as the preemptive priority, and the back is the response priority. According to this grouping, the 4-bit can be divided into 5 groups in total.
Group 0: All 4 bits are used to specify the response priority;
Group 1: The highest bit is used to specify the preemptive priority, and the following 3 bits are used to specify the response priority;
Group 2: The top 2 bits are used to specify the preemptive priority, and the next 2 bits are used to specify the response priority;
Group 3: The top 3 bits are used to specify the preemptive priority, and the last bit is used to specify the response priority;
Group 4: All 4 bits are used to specify preemptive priority.
The so-called preemptive priority and response priority, the relationship between them is: an interrupt with a high preemptive priority can be responded to during the interrupt processing with a low preemptive priority, that is, interrupt nesting.
When the preemptive priority of two interrupt sources is the same, there will be no nesting relationship between the two interrupts. When an interrupt arrives, if another interrupt is being processed, the later interrupt will have to wait until the previous interrupt is processed. If the two interrupts arrive at the same time, the interrupt controller decides which one to process first based on their response priority; if their preemptive priority and response priority are equal, it decides which one to process first based on their ranking order in the interrupt table. Each interrupt source must define 2 priorities.
There are a few things to note:
1) If the specified preemptive priority or response priority exceeds the range defined by the selected priority group, unexpected results may occur;
2) There is no nesting relationship between interrupt sources with the same preemptive priority level;
3) If an interrupt source is assigned a preemptive priority level and there is no other interrupt source at the same preemptive priority level, any valid response priority level can be assigned to this interrupt source.
2 GPIO external interrupt
In STM32, each GPIO can trigger an external interrupt, but the GPIO interrupt is a unit of group bit, and only one external interrupt in the same group can be used at the same time. For example, PA0, PB0, PC0, PD0, PE0, PF0, PG0 are 1 group. If we use PA0 as the external interrupt source, then the others cannot be used. In this case, we can only use external interrupt sources with different terminal numbers such as PB1 and PC2. Each group uses an interrupt flag EXTIx. The five external interrupts EXTI0 - EXTI4 have their own separate interrupt response functions, EXTI5-9 share an interrupt response function, and EXTI10-15 share an interrupt response function.
For interrupt control, STM32 has a dedicated management mechanism: NVIC. For a detailed explanation of NVIC, you can refer to "ARM Cortex-M3 Authoritative Guide", written by Joseph Yiu, translated by Song Yan, published by Beijing University of Aeronautics and Astronautics Press, Chapter 8 NVIC and Interrupt Control. The enable, suspension, priority, activity, etc. of interrupts are all managed by NVIC. Because my focus in learning STM32 is on how to develop programs, I will not explain some of the internal things in detail here. If you are interested, you can refer to the book mentioned above.
3. Program Development
In fact, the basic concepts and knowledge above are just a general understanding of the STM32 interrupt system. Using programs will help you better understand how to use interrupts. The basic steps for using external interrupts are as follows:
1. Set the corresponding clock;
2. Set the corresponding interrupt;
3. IO port initialization;
4. Set the corresponding IO port as an interrupt line (before setting the external interrupt) and initialize it;
5. Interrupt function in the response function of the selected interrupt channel.
Since the Struggle development board I used did not lead out the corresponding chip pins, I could only use buttons to trigger the corresponding interrupts. According to the schematic diagram, K1/K2/K3 are connected to PC5/PC2/PC3, so I will use three external interrupts EXTI5/EXTI2/EXTI3. PB5/PD6/PD3 are connected to three LED lights respectively. The effect of the interrupt is that when the button is pressed, the corresponding LED light will be lit.
1. Set the corresponding clock
First, you need to turn on GPIOB, GPIOC, and GPIOE (because the other end of the button is connected to the PE port). Then, because it is used to trigger an interrupt, you also need to turn on the GPIO multiplexing clock. The corresponding functions are explained in detail in the GPIO study notes. The detailed code is as follows:
void RCC_cfg()
{
// Turn on the PE PD PC PB port clock and turn on the multiplexed clock
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOE | RCC_APB2Periph_GPIOC | RCC_APB2Periph_GPIOD | RCC_APB2Periph_GPIOB | RCC_APB2Periph_AFIO, ENABLE);
}
The RCC function required to set the corresponding clock is in stm32f10x_rcc.c, so add this file to the project.
2. Set up the corresponding interrupt
Setting the corresponding interrupt is actually setting NVIC. In the STM32 firmware library, there is a structure NVIC_InitTypeDef, which contains the corresponding flag bit settings, and then initialized with the NVIC_Init() function. The detailed code is as follows:
void NVIC_cfg()
{
NVIC_InitTypeDef NVIC_InitStructure;
NVIC_PriorityGroupConfig(NVIC_PriorityGroup_2); //Select interrupt group 2
NVIC_InitStructure.NVIC_IRQChannel = EXTI2_IRQChannel; //Select interrupt channel 2
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0; //Set the preemptive interrupt priority to 0
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0; // Set the responsive interrupt priority to 0
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE; //Enable interrupt
NVIC_Init(&NVIC_InitStructure);
NVIC_InitStructure.NVIC_IRQChannel = EXTI3_IRQChannel; //Select interrupt channel 3
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 1; //Set the preemptive interrupt priority to 1
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 1; // Set the responsive interrupt priority to 1
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE; //Enable interrupt
NVIC_Init(&NVIC_InitStructure);
NVIC_InitStructure.NVIC_IRQChannel = EXTI9_5_IRQChannel; //Select interrupt channel 5
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 2; //Set the preemptive interrupt priority to 2
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 2; // Set the responsive interrupt priority to 2
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE; //Enable interrupt
NVIC_Init(&NVIC_InitStructure);
}
Since there are 3 interrupts, according to the previous article, 3 bits are required to specify the preemption priority, so the second group is selected. Since EXTI5-9 share an interrupt response function, the interrupt channel selected by EXTI5 is EXTI9_5_IRQChannel. Detailed information can be found in the header file. The NVIC-related library functions used are in stm32f10x_nivc.c. This file needs to be copied and added to the project. For the specific location, see the notes about GPIO. This code compiles without any problems, but it will report an error when linking. You need to add STM32F10xR.LIB to the project. The specific location is...KeilARMRV31LIBSTSTM32F10xR.LIB. [page]
3. IO port initialization
void IO_cfg()
{
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_2; //Select pin 2
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz; //Maximum output frequency 50MHz
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP; //Output with pull-up resistor
GPIO_Init(GPIOE,&GPIO_InitStructure);
GPIO_ResetBits(GPIOE,GPIO_Pin_2); //Set PE.2 pin to low level output
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_2 | GPIO_Pin_3 | GPIO_Pin_5; //Select pins 2 3 5
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING; //Select input mode as floating input
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz; //Maximum output frequency 50MHz
GPIO_Init(GPIOC,&GPIO_InitStructure); //Set PC.2/PC.3/PC.5
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_3 | GPIO_Pin_6; //Select pin 3 6
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz; //Maximum output frequency 50MHz
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP; //Output with pull-up resistor
GPIO_Init(GPIOD,&GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_5; //Select pin 5
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz; //Maximum output frequency 50MHz
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP; //Output with pull-up resistor
GPIO_Init(GPIOB,&GPIO_InitStructure);
}
The pin connected to the external interrupt needs to be set to input state, and the pin connected to the LED needs to be set to output state. Initializing PE.2 is to make the other end of the button output a low level. The functions in GPIO are in stm32f10x_gpio.c.
4. Set the corresponding IO port as an interrupt line
Since GPIO is not a dedicated interrupt pin, when using GPIO to trigger an external interrupt, it is necessary to connect the corresponding GPIO pin and the interrupt line. The specific code is as follows:
void EXTI_cfg()
{
EXTI_InitTypeDef EXTI_InitStructure;
// Clear the interrupt flag
EXTI_ClearITPendingBit(EXTI_Line2);
EXTI_ClearITPendingBit(EXTI_Line3);
EXTI_ClearITPendingBit(EXTI_Line5);
//Select interrupt pins PC.2 PC.3 PC.5
GPIO_EXTILineConfig(GPIO_PortSourceGPIOC, GPIO_PinSource2);
GPIO_EXTILineConfig(GPIO_PortSourceGPIOC, GPIO_PinSource3);
GPIO_EXTILineConfig(GPIO_PortSourceGPIOC, GPIO_PinSource5);
EXTI_InitStructure.EXTI_Line = EXTI_Line2 | EXTI_Line3 | EXTI_Line5; //Select interrupt line 2 3 5
EXTI_InitStructure.EXTI_Mode = EXTI_Mode_Interrupt; //Set to interrupt request, not event request
EXTI_InitStructure.EXTI_Trigger = EXTI_Trigger_Rising_Falling; //Set the interrupt trigger mode to rising and falling edge trigger
EXTI_InitStructure.EXTI_LineCmd = ENABLE; //External interrupt enable
EXTI_Init(&EXTI_InitStructure);
}
The functions that need to be called in EXTI_cfg are all in stm32f10x_exti.c.
5. Write interrupt response function
Unlike C51 microcontrollers, STM32 can use the interrupt keyword to define interrupt response functions. The STM32 interrupt response function interface is stored in the interrupt vector table, which is given by the startup code. The default interrupt response function is in stm32f10x_it.c. Therefore, we need to add this file to the project.
In this file, we found that many functions only have a function name, but no function body. We found the function EXTI2_IRQHandler(), which is the function for EXTI2 interrupt response. My goal is to light up the LED, so the function body is actually very simple:
void EXTI2_IRQHandler(void)
{
//Light up the LED
GPIO_SetBits(GPIOD,GPIO_Pin_6);
// Clear the interrupt flag to prevent continuous interruption
EXTI_ClearITPendingBit(EXTI_Line2);
}
void EXTI3_IRQHandler(void)
{
GPIO_SetBits(GPIOD,GPIO_Pin_3);
EXTI_ClearITPendingBit(EXTI_Line3);
}
void EXTI9_5_IRQHandler(void)
{
GPIO_SetBits(GPIOB,GPIO_Pin_5);
EXTI_ClearITPendingBit(EXTI_Line5);
}
Since EXTI5-9 share the same interrupt response function, all response functions of EXTI5 - EXTI9 are written in this one.
6. Write the main function
#include "stm32f10x_lib.h"
void RCC_cfg();
void IO_cfg();
void EXTI_cfg();
void NVIC_cfg();
int main()
{
RCC_cfg();
IO_cfg();
NVIC_cfg();
EXTI_cfg();
while(1);
}
There is a function declaration before the main function. The main function body calls the initialization configuration function and then enters an infinite loop, waiting for the interrupt response.
Since this article involves many library functions, we can find out what the corresponding functions do by looking up the library function documentation in "ARM®-based 32-bit MCU STM32F101xx and STM32F103xx firmware library". There is also a Chinese version of the documentation available online for reference.
Previous article:STM32 Learning Notes (3): System Clock and SysTick Timer
Next article:STM32 learning notes (1): Use of GPIO ports
Recommended ReadingLatest update time:2024-11-16 17:46
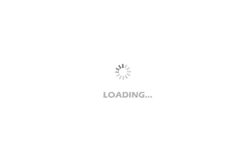
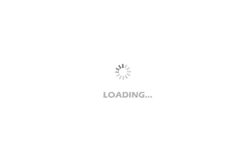
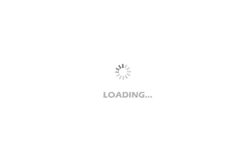
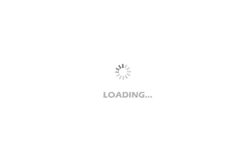
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- 【AT32WB415 Review】Serial communication test (including Bluetooth test)
- TI Voltage Reference (VREF) Application Design Tips and Tricks
- What happens if the input voltage of the window comparator is equal to the upper and lower limits?
- Banknote number recognition system based on ARM.pdf
- My knowledge of computers
- Sampling rate of MCU ADC
- [Sipeed LicheeRV 86 Panel Review] 7-Waft Graphical Interface Development Test (Part 2)
- I don't understand the principle of ultrasonic signal processing.
- Let’s talk about the first big event in 2019...
- Wifi controlled LED screen