environment:
Host: XP
Development environment: MDK4.10
Microcontroller: STM32F103C8
Function:
Turn on the RTC alarm, then enter standby mode, wake up with the alarm and exit.
illustrate:
1. When the RTC alarm wake-up event occurs, the alarm interrupt is entered at the same time, and must be associated with the external interrupt line 17 during initialization
2. If you only want to exit standby mode, the RTC alarm event is sufficient and does not need to be associated with external interrupt line 17
3. After exiting standby mode, the following process is similar to pressing the reset button, and the program will start from the beginning
source code:
Initialize the clock, configure the clock to the internal clock LSI, configure the RTC alarm wake-up and external interrupt line 17
void RTC_Configuration(void)
{
//Define the interrupt structure
NVIC_InitTypeDef NVIC_InitStructure;
EXTI_InitTypeDef EXTI_InitStructure;
//Interrupt clock enable
RCC_APB2PeriphClockCmd(RCC_APB2Periph_AFIO, ENABLE);
//Interrupt priority configuration
NVIC_PriorityGroupConfig(NVIC_PriorityGroup_1);
//Set up RTC alarm interrupt
NVIC_InitStructure.NVIC_IRQChannel = RTCAlarm_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 1;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
//The alarm interrupt is connected to the 17th line external interrupt
EXTI_ClearITPendingBit(EXTI_Line17);
EXTI_InitStructure.EXTI_Line = EXTI_Line17;
EXTI_InitStructure.EXTI_Mode = EXTI_Mode_Interrupt;
EXTI_InitStructure.EXTI_Trigger = EXTI_Trigger_Rising;
EXTI_InitStructure.EXTI_LineCmd = ENABLE;
EXTI_Init(&EXTI_InitStructure);
//PWR_WakeUpPinCmd(DISABLE);
//Power management part clock is turned on
RCC_APB1PeriphClockCmd(RCC_APB1Periph_PWR | RCC_APB1Periph_BKP, ENABLE);
// Enable backup register access
PWR_BackupAccessCmd(ENABLE);
BKP_ClearFlag();
BKP_DeInit();
// Enable LSI
RCC_LSICmd(ENABLE);
//Wait for the crystal to start
while (RCC_GetFlagStatus(RCC_FLAG_LSIRDY) == RESET)
{}
//Set the clock to the internal crystal oscillator
RCC_RTCCLKConfig(RCC_RTCCLKSource_LSI);
RCC_RTCCLKCmd(ENABLE);
//Wait for the RSF bit (register synchronization flag) in the RTC_CTL register to be set to 1 by hardware
RTC_WaitForSynchro();
RTC_WaitForLastTask();
// Enable alarm interrupt
RTC_ITConfig(RTC_IT_ALR, ENABLE);
RTC_WaitForLastTask();
//Frequency division coefficient
RTC_SetPrescaler(40000); /* RTC period = RTCCLK/RTC_PR = (32.768 KHz)/(32767+1) */
RTC_WaitForLastTask();
//Initial count value
RTC_SetCounter(0);
RTC_WaitForLastTask();
//Set the alarm time
RTC_SetAlarm(2);
RTC_WaitForLastTask();
}
Alarm wake-up interrupt code:
void RTCAlarm_IRQHandler(void)
{
//Wait for the RSF bit (register synchronization flag) in the RTC_CTL register to be set to 1 by hardware
RTC_WaitForSynchro();
if (RTC_GetITStatus(RTC_IT_ALR) != RESET)
{
USART_SendData(USART1,'d'); //Send data
while(USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET){} //Wait for sending to end
USART_SendData(USART1,'i'); //Send data
while(USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET){} //Wait for sending to end
// Clear EXTI_Line17 suspend bit
EXTI_ClearITPendingBit(EXTI_Line17);
// Check if the wakeup flag is set
if(PWR_GetFlagStatus(PWR_FLAG_WU) != RESET)
{
// Clear the wake-up flag
PWR_ClearFlag(PWR_FLAG_WU);
}
/* Clear the RTC Second interrupt */
RTC_SetCounter(0);
RTC_WaitForLastTask();
RTC_ClearITPendingBit(RTC_IT_ALR);
RTC_WaitForLastTask();
//RTC_SetAlarm(2);
//RTC_WaitForLastTask();
}
return;
}
Test code: (This is part of the project and contains some irrelevant code)
int main(void)
{
struct _match_string_header match_string_header;
struct _match_string_tail match_string_tail;
unsigned char buffer[LEN_BUF];
unsigned char buffer1[LEN_BUF];
int len = 0;
int i = 0;
int j = 0;
int flag = 0;
int flag2 = 0;
int flag3 = 0;
int baud = 0;
unsigned short temp = 0;
// Initialize the system
heat();
// Initialize Bluetooth
//Read the baud rate in flash
//write_baud(&edit_flash,9600);
//baud = read_baud(&edit_flash);
//Read valid
if (baud > 0)
{
set_uart_baud(1,baud);
set_uart_baud(2,baud);
}
else
{
//Set the default baud rate
set_uart_baud(1,DEFAULT_BAUD);
set_uart_baud(2,DEFAULT_BAUD);
}
//Set the default baud rate
//Delay(10);
init_blue(DEFAULT_BAUD);
set_uart_baud(1,DEFAULT_BAUD);
set_uart_baud(2,DEFAULT_BAUD);
//Delay(500);
init_blue(DEFAULT_BAUD);
set_uart_baud(1,DEFAULT_BAUD);
set_uart_baud(2,DEFAULT_BAUD);
// Initialize matching characters
init_match_string_header(&match_string_header,"AT+BAUD");
init_match_string_tail(&match_string_tail,"END",8);
//Read the value in backup register 2
temp = BKP_ReadBackupRegister(BKP_DR2);
if (temp == 0xabcd)
{
USART_SendData(USART1,'j'); //Send data
while(USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET){} //Wait for sending to end
USART_SendData(USART1,'d'); //Send data
while(USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET){} //Wait for sending to end
USART_SendData(USART1,'h'); //Send data
while(USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET){} //Wait for sending to end
}
else
{
//Write to backup register 2
BKP_WriteBackupRegister(BKP_DR2,0xabcd);
USART_SendData(USART1,'9'); //Send data
while(USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET){} //Wait for sending to end
USART_SendData(USART1,'9'); //Send data
while(USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET){} //Wait for sending to end
}
//Test low power consumption: standby mode
for (i = 0;i < 30000;i++)
{
for (j = 0;j < 500;j++)
{
__nop();
}
}
PWR_EnterSTANDBYMode();
while (1);}
Previous article:STM32 external interrupt test
Next article:STM32F103 and STM32F407 pin compatibility issues
Recommended ReadingLatest update time:2024-11-17 02:36
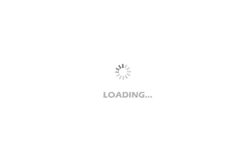
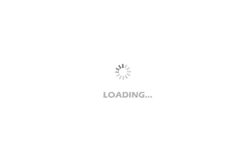
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- Rambus Launches Industry's First HBM 4 Controller IP: What Are the Technical Details Behind It?
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- [Discussion] There is no place to make money during the seven days of National Day holiday
- ESP32 firmware is divided into two versions: ESP-IDF v3.x/4.x
- Two methods of programming TMS320C6748
- Enabling higher-performance front-end radar to make Vision Zero a reality
- Power supply control principle of three-phase fuel pump used in Mercedes-Benz passenger cars
- Review summary: Free review of Fudan Micro FM33LG0 series, Winsilver chip
- ESP32-S2-Saola-1 calculates pi
- DCDC H-bridge circuit, what is the principle of boost and buck?
- How to measure positive and negative 1.5V signals using a single power supply system?
- [Zhongke Bluexun AB32VG1 RISC-V evaluation board] Current and voltage detection project development