Section 1 Absolute Address Access
C51 provides three methods to access absolute addresses:
1. Absolute macros:
In the program, use "#include" to use the macros defined in it to access absolute addresses, including:
CBYTE, XBYTE, PWORD, DBYTE, CWORD, XWORD, PBYTE, DWORD.
For specific usage, you can take a look at absacc.h. For
example:
rval=CBYTE[0x0002]; points to the address 0002h of the program memory
rval=XWORD [0x0002]; points to the address 0004h of the external RAM
2. _at_ keyword
Just add _at_ const after the data definition, but note:
(1) Absolute variables cannot be initialized;
(2) Bit-type functions and variables cannot be specified with _at_.
For example:
idata struct link list _at_ 0x40; specifies that the list structure starts at 40h.
xdata char text[25b] _at_0xE000; specifies that the text array starts from 0E000H.
Tip: If the external absolute variable is an I/O port or other data that can change by itself, it needs to be described using the volatile keyword, please refer to absacc.h.
3. Connection positioning control
This method uses the connection control instruction code xdata pdata data bdata to perform "segment" address. If you want to specify a specific variable address, it is very limited and will not be discussed in detail.
Section 2 Keil C51 and assembly interface
1. The interface method within the module
is to use the #pragma statement. The specific structure is:
#pragma asm
assembly line
#pragma endasm
This method essentially tells the C51 compiler through asm and ndasm that the intermediate line does not need to be compiled into an assembly line, so there is SRC in the compilation control instruction to control the storage of these non-compiled lines.
2. Interface between modules
The interface between C module and assembly module is relatively simple. Use C51 and A51 to compile the source file respectively, and then use L51 to connect the obj file. The key issue is the parameter passing between C function and assembly function. There are two parameter passing methods in C51.
(1) Passing function parameters through registers.
At most 3 parameters can be passed through registers. The rules are as follows:
Number of parameters | char | int | long,float | General pointers |
1 | R7 | R6 & R7 | R4~R7 | R1~R3 |
(2) Fixed memory transfer:
This method transfers bit type parameters to a storage segment:
? function_name?BIT.
Other types of parameters are transferred to the following segment: ? function_name?BYTE, and are stored in a pre-selected order.
As for where this fixed storage area is located, it is determined by the storage mode.
(3) Function return value:
The function return value is always placed in a register, with the following rules:
Return type | Registev | illustrate |
bit | Flags | Returned by specific flags |
char/unsigned char 1_byte pointer | R7 | A single byte is returned by R7 |
int/unsigned int 2_byte pointer | R6 & R7 | The double byte is returned by R6 and R7, with the MSB in R6 |
long&unsigned long | R4~R7 | MSB at R4, LSB at R7 |
float | R4~R7 | 32-bit IEEE format |
General pointers | R1~R3 | Storage type in R3 high R2 low R1 |
(4) SRC control
This control instruction compiles the C file to generate an assembly file (.SRC). The assembly file can be renamed to generate an assembly .ASM file, and then compiled with A51.
Section 3 Common files in the Keil C51 software package
There are several C source files in the C51LiB directory. These C source files play a very important role. With a slight modification, they can be used in your own dedicated system.
1. Dynamic memory allocation
init_mem.c: This file is the source code for initializing the dynamic memory area. It can specify the location and size of the dynamic memory. Only when init_mem() is used can other functions such as malloc, calloc, realloc, etc. be called back.
calloc.c: This file is the source code for allocating memory to an array. It can specify the unit data type and the number of units.
malloc.c: This file is the source code of malloc, which allocates a fixed size of memory.
realloc.c: This file is the source code of realloc.c, and its function is to adjust the size of the currently allocated dynamic memory.
2. C51 startup file STARTUP.A51
The startup file STARTUP.A51 contains the target board startup code. This file can be added to each project. As long as it is reset, the file will be executed immediately. Its functions include:
defining the internal RAM size, external RAM size, and reentrant stack position;
clearing the internal, external, or external memory in units of pages;
initializing the reentrant stack and stack pointer according to the storage mode;
initializing the 8051 hardware stack pointer
to the main() function; developers
can modify the following data to initialize the system.
Constant Name Meaning
IDATALEN Length of internal RAM to be cleared
XDATA START Specify the starting address of external RAM to be cleared
XDATALEN Length of external RAM to be cleared
IBPSTACK Whether the small mode reentrant stack pointer needs to be initialized, 1 means required. The default value is 0.
IBPSTACKTOP specifies the top address of the small mode reentrant stack. XBPSTACK specifies
whether the large mode reentrant stack pointer needs to be initialized. The default value is 0. XBPSTACKTOP specifies the top address of the large mode reentrant stack . PBPSTACK specifies whether the Compact reentrant stack pointer needs to be initialized. The default value is 0. PBPSTACKTOP specifies the top address of the Compact mode reentrant stack. PPAGEENABLE P2 initialization enable switch PPAGE specifies P2 value PDATASTART The first address of the external RAM page to be cleared PDATALEN The length of the external RAM page to be cleared. Tip: If you want to initialize P2 as the high-end address of the compact mode, you must: PPAGEENAGLE=1, PPAGE is the P2 value, for example, specify a page 1000H-10FFH, then PPAGE=10H, 3. Standard input and output file putchar.c putchar.c is a low-level character output subroutine. Developers can modify it and apply it to their own hardware systems, such as outputting characters to CLD or LEN. Default: putchar.c outputs a character to the serial port. XON and XOFF are flow control marks. The line feed character "*n" is automatically converted to carriage return/line feed " ". getkey.c The getkey function is a low-level character input subroutine. This program can be used in your own hardware system, such as matrix keyboard input. By default, characters are input through the serial port. 4. Other files include the INIT.A51 function with unique functions for Watch-Dog and functions applicable to 8×C751. Please refer to the source code. Section 4 Segment Naming Conventions and Program Optimization 1. Segment Naming Conventions The target files generated by the C51 compiler are stored in many segments. These segments are some units of code space or data space. A segment can be relocatable or absolute. Each relocatable segment has a type and name. The C51 segment name has the following regulations: Each segment name consists of a prefix and a module name. The prefix indicates the storage type, and the module name is the name of the compiled module. For example: ? CO? main1: Indicates the constant part of the code segment in the main1 module ? PR? function1? module refers to the executable segment of function function1 in module module. For specific regulations, please refer to the manual. 2. Program optimization C51 compiler is a compiler with optimization function. It provides six levels of optimization function. Ensure the highest efficiency of generating target code (least code, fastest running speed). For specific six-level optimization content, please refer to the help. The following compilation control instructions are provided in C51 to control code optimization: OPTIMIZE (SJXE): Try to use subroutines to reduce program code. NOAREGS: Do not use absolute register access, and the program code is independent of the register segment. NOREGPARMS: Parameter passing is always implemented in the local data segment, and the program code is compatible with the lower version of C51. OPTIMIZE (SIZE) AK OPTIMIZE (speed) provides 6 levels of optimization function, the default is: OPTIMIZE (6, SPEED).
Previous article:Keil C51 compilation error warning solution accumulation
Next article:How to use Keil C51 software
Recommended ReadingLatest update time:2024-11-16 19:25
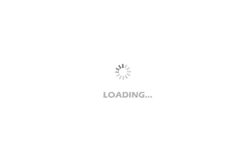
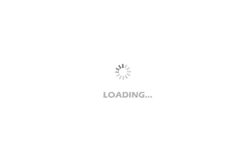
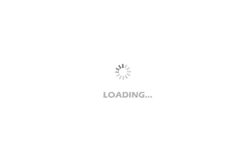
![Quickly learn Arm (20)--Interrupt Vector Controller VIC[2]](https://6.eewimg.cn/news/statics/images/loading.gif)
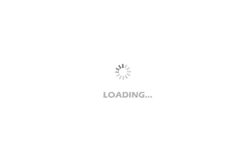
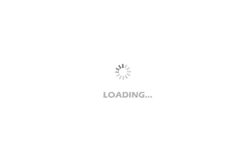
- Popular Resources
- Popular amplifiers
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
How to read electrical control circuit diagrams (Classic best-selling books on electronics and electrical engineering) (Zheng Fengyi)
-
Multithreaded computing in embedded real-time operating systems - based on ThreadX and ARM
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Chapter 3 Interrupts, Clocks, and Low Power Consumption
- The difference between C64x+ and C64x CACHE in the C6000 series
- Realization of voltage to current conversion in Multisim12
- 【Smart Network Desk Lamp】7. Get real-time weather information and analyze it
- [RISC-V MCU CH32V103 Review] +01 Try the use of GPIO to light up the first LED
- PCB layout optimization of multi-output buck converter based on phase-shift control to improve EMI performance
- [Raspberry Pi Pico Review] Small size, big use~PICO here I come~
- 12V 60Ah large capacity lithium battery
- Review summary: National Technology's new M4F core N32G430 is here~
- Can you tell me what the Cf2 capacitor does in the circuit? Thanks!