question:
How do I write a C program to echo characters from an 8051 serial interface?
answer:
The functions _getkey and putchar use the on-chip serial port to perform serial I/O. These subroutines are included in the C51 library, and the source files for each function are located in the \C51\LIB directory. See your C51 user manual for more information about these subroutines.
The following example program sets up the on-chip serial port, sets the baud rate, and waits for a character (using the _getkey function). When a character is received, it outputs it using the putchar function.
#i nclude "reg51.h" void main (void) while (1) |
question:
I want to call a function written in C from my assembly program. How can I do this?
answer:
The easiest way is to let the C compiler generate the correct assembly code for you.
Suppose you have a C function called "foo" that takes a single parameter of type unsigned char and returns an unsigned char. In a new C file, write a dummy function, also called "foo". Here is an example:
#pragma src extern unsigned char foo(unsigned char); void dummy(void) |
When the file is compiled, #pragma SRC instructs the C compiler to generate assembly code. The extension of the assembly file is "src".
If you look at the src file, you will find out how to call the function "foo" from assembly. The file shows the registers and memory addresses used to pass function variables, and the registers and memory addresses used to return values. In addition, it also tells you the correct function naming conventions, which is necessary for interfacing assembly with C.
Then, you can use the src file as a template to write your own assembly call code. Note that you must include the EXTRN directive in the function, that is:
EXTRN CODE (_foo)
question:
In the C51 compiler manual, there is an example of an assembly function calling a C function. So is there an example of a C program calling an assembly subroutine?
answer:
It's not in the manual, but it's easy to make one yourself. An ASM subroutine must know how parameters are passed, what the return value is, and the naming conventions for segments. Follow the steps below and you can make one yourself.
Write a simple function in C that passes parameters and returns values the same way you would write it in assembly.
Use the SRC directive (#PRAGMA SRC at the top of the file) to have the C compiler generate a .SRC file instead of an .OBJ file.
Compile the C file. Because the SRC directive is used, a .SRC file is generated. The .SRC file contains the assembly code generated from the C code you wrote.
Rename the .SRC file to .A51 file.
Edit the .A51 file and insert the assembly code you want to execute in the assembly function body.
For example, the following code
#pragma SRC unsigned char my_assembly_func { unsigned int argument) { return (argument + 1); // Insert dummy lines to access all variables and intevals. } |
The SRC file is generated when compiling.
question:
Do you have any examples of mixed C and assembly programming?
answer:
The following example shows how to mix C and assembly in an 8051 program.
This example starts with a MAIN C function, which calls an assembly subroutine and then calls a C function.
The MAIN C module is as follows:
extern void a_func (void); void main (void) |
Function a_func is an assembly subroutine:
NAME A_FUNC ?PR?a_func?A_FUNC SEGMENT CODE RSEG ?PR?a_func?A_FUNC END |
Note that the assembler calls the C function c_func:
void c_func (void) { } |
The actual code for the assembly module is generated using the SRC pragma directive and the following C source file:
extern void c_func (void); void a_func (void) |
You can download C2ASM2C.ZIP from the Keil website.
Previous article:Calendar clock program written in C513
Next article:How to locate a subroutine segment at a fixed address in C51
Recommended ReadingLatest update time:2024-11-16 15:39
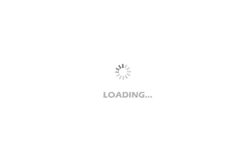
- Popular Resources
- Popular amplifiers
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
How to read electrical control circuit diagrams (Classic best-selling books on electronics and electrical engineering) (Zheng Fengyi)
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- How to use Labview to perform secondary development on Ginkgo 2
- Design principle and usage of true random number generator of low power MCU RJM8L303
- How to make an elevator controller based on 8952 microcontroller?
- Interpretation of JTAG interface for embedded development ARM technology
- EEWORLD University ---- Jixin STM32 Smart Car
- Experience in using PWM of 28069 and 28377D of C28x series
- At 10:00 this morning, we invite you to listen to the award-winning live broadcast: ADI's digital active noise reduction headphone solution allows technology to calm us down~
- Common MOS tube models and parameter comparison table
- From terminals to architecture, TE Connectivity (TE) helps you connect to the 5G high-speed future. Watch the video and answer questions to win gifts!
- ST's latest evaluation activity! Get the first-hand experience of the NUCLEO_G431RB development board here