Cx51 programs can be easily interfaced with 8051 assemblers. The A51 assembler is an 8051 macro assembler that emits object modules in OMF-51 format. By observing some programming rules, you can call assembler programs from C programs and vice versa. Public variables declared in assembly modules can also be used in C programs.
There are several reasons to call an assembler from a C program. First, you can use an existing assembler, second, you want it to run faster, and third, you want to use it directly in assembly to manipulate SFRs or use I/O memory images.
For an assembler to be called from a C program, its parameter passing rules and value return rules must be consistent with C functions. In terms of application, it must look like a C function.
Function Parameters
In general, a C function can pass three parameters through registers. The other parameters are passed through fixed memory. Of course, NOREGPARAMS can be used to prohibit the use of registers to pass parameters. If it is specified that registers are not allowed to pass parameters or there are too many parameters, fixed memory areas are used to pass parameters. When generating code, the function that passes parameters in registers has "_" added to the front of the name, and the function that uses fixed memory areas to pass parameters does not change its name.
Parameter Passing in Registers
Passing parameters in registers
C functions can pass parameters in registers or in fixed storage areas. Up to three parameters can be passed in registers. The following table shows the rules for parameter passing:
Arg Number char, 1-byte ptr int, 2-byte ptr long, float generic ptr
1 R7 R6 & R7 R4—R7 R1—R3
(MSB in R6, (Mem type in R3,
LSB in R7) MSB in R2
LSB in R1)
2 R5 R4 & R5 R4—R7 R1—R3
(MSB in R4, (Mem type in R3
LSB in R5) MSB in R2
LSB in R1)
3 R3 R2 & R3 R1—R3
(MSB in R2, (Mem type in R3
LSB in R3) MSB in R2,
LSB in R1)
Example
Declaration Description
func1 (int a) parameter a is passed through R6 and R7
func2 (int b,int c,int *d) parameter b is passed through R6 and R7, parameter c is passed through R4 and R5, and parameter d is passed through R1, R2 and R3
func3 (long e, long f) parameter e is passed through R4, R5, R6, R7, and parameter f is passed through a fixed storage area
func4 (float g, char h) parameter g is passed through R4, R5, R6, R7, and parameter h is passed through a fixed storage area
Parameter Passing in Fixed Memory Locations
Passing parameters via fixed storage area
Procedures that pass parameters through fixed storage areas ?function_name?BYTE and ?function_name?BIT name and carry parameter values and pass them to function_name. Bit parameters are copied to the ?function_name?BIT segment before calling the function. All other parameter data is copied to the ?function_name?BYTE segment. All parameters are assigned storage space within these segments, even if they may use registers to pass parameters. Parameters are stored in the corresponding segments in the order they are declared.
Depending on the storage mode, the fixed storage area for parameter transfer may be the internal data memory or the external data memory. The small storage mode uses the internal data memory as the parameter transfer area, which is the most efficient. The Compact and large storage modes use the external data memory as the parameter transfer area.
Function Return Values
The return value of a function always uses CPU registers. The following table lists the registers used by possible return values.
Return Value Type Register Description
bit Carry Flag A single bit is returned in the carry position
char / unsigned char, R7 A single byte is returned in R7
1-byte pointer
int / unsigned int, R6 & R7 high bit in R6, low bit in R7
2-byte ptr
long / unsigned long R4-R7 The high bit is in R4, the low bit is in R7
float R4-R7 32-bit IEEE format
generic pointer R1-R3 memory type in R3, high in R2, low in R1
Using the SRC Directive
You can use the Cx51 compiler to generate an assembly code program, and use this program to determine the transfer rules that should be used. The instruction SRC can specify that Cx51 generate an assembly program instead of an object program. For example, the following C program:
#pragma SRC
#pragma SMALL
unsigned int asmfunc1 (
unsigned int arg)
{
return (1 + arg);
}
Generates the following assembler:
; ASM1.SRC generated from: ASM1.C
NAME ASM1
?PR?_asmfunc1?ASM1 SEGMENT CODE
PUBLIC _asmfunc1
; #pragma SRC
; #pragma SMALL
;
; unsigned int asmfunc1 (
RSEG ?PR?_asmfunc1?ASM1
USING 0
_asmfunc1:
;---- Variable 'arg?00' assigned to Register 'R6/R7' ----
; SOURCE LINE # 4
; SOURCE LINE # 6
; return (1 + arg);
; SOURCE LINE # 7
MOV A,R7
ADD A,#01H
MOV R7,A
CLR A
ADDC A,R6
MOV R6,A
; }
; SOURCE LINE # 8
?C0001:
RIGHT
; END OF _asmfunc1
END
In this example, the function name asmfunc1 is prefixed with an underscore in the assembler to indicate that the parameters are passed through registers. The parameter arg is passed through registers R6 and R7.
The following program is an assembler compiled from the same source program, except that the NOREGPARMS directive is used:
; ASM2.SRC generated from: ASM2.C
NAME ASM2
?PR?asmfunc1?ASM2 SEGMENT CODE
?DT?asmfunc1?ASM2 SEGMENT DATA
PUBLIC ?asmfunc1?BYTE
PUBLIC asmfunc1
RSEG ?DT?asmfunc1?ASM2
?asmfunc1?BYTE:
arg?00: DS 2
; #pragma SRC
; #pragma SMALL
; #pragma NOREGPARMS
;
; unsigned int asmfunc1 (
RSEG ?PR?asmfunc1?ASM2
USING 0
asmfunc1:
; SOURCE LINE # 5
; SOURCE LINE # 7
; return (1 + arg);
; SOURCE LINE # 8
MOV A,arg?00+01H
ADD A,#01H
MOV R7,A
CLR A
ADDC A,arg?00
MOV R6,A
; }
; SOURCE LINE # 9
?C0001:
RIGHT
; END OF asmfunc1
END
Note that in the example, there is no underscore prefix to the function name asmfunc1, and the parameters are passed in the ?asmfunc1?BYTE segment.
Previous article:C51 Compiler - Advanced Programming Skills (4) - Register Application
Next article:C51 Compiler - Advanced Programming Skills (2) - Segment Naming Convention
Recommended ReadingLatest update time:2024-11-17 02:34
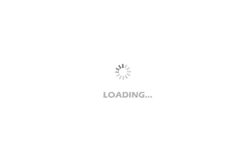
- Popular Resources
- Popular amplifiers
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
Modern Compiler Principles C Language Description (Ampel)
-
How to read electrical control circuit diagrams (Classic best-selling books on electronics and electrical engineering) (Zheng Fengyi)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- Rambus Launches Industry's First HBM 4 Controller IP: What Are the Technical Details Behind It?
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- TI can solve common problems of high-precision ultrasonic flow measurement at low flow rates
- c2000 I2C read register frame format reference routine
- Javascript Smart Watch
- Introduction to Zigbee communication technology transmission distance and wall penetration capability
- 51 single chip microcomputer
- HyperLynx High-Speed Circuit Design and Simulation (XI) Quantitative Analysis of Signal Overdrive and Underdrive
- Data丨Xunwei IMX6ULL development board-main frequency and clock configuration routine (Part 2)
- Problems still occur after installing AD16
- [Environmental Expert's Smart Watch] Part 6: BLE broadcast temperature, humidity and air pressure data
- How to clear the OE bit of the TMS320C6748 serial port register LSR