#include
#include
#include "lcdbn.h"
uchar data1[4]={0,2,5,0};
uchar data2[4]= {0,0,0,0};
uchar j,key=0;
uchar chr[]="pre: *2%";
uchar *chr1[3]={"openc V: ",
"shutc V: ",
"acceler: "} ;
uchar counter1=0x84;
uchar counter2=0xc8;
uchar flag; //flag is the switch enable
int E1r;
int E2r=0,E3r=0;
uint speedr=15,c_r=0;// maxc=700;
void init_intr(void)
{
SREG=0x80;
TIMSK=0x40;
TCCR2=0x06;
TCCR1A=0xe3; //A is high level, B is low level
TCCR1B=0x0a;
MCUCR=0x0a; ///External INT1/0 interrupt rising valid
GICR=0xc0;
}
/////////// ///////////////////////////
/////Left and right motor PWM settings
/////////////// //////////////////////
void setpwmr(uchar *p)
{ uint temp1;
temp1=(uint)p[1]*10+p[2];
if( temp1<25)
{
speedr=(uint)28-temp1*28/25;
}
else
{
speedr=(uint)temp1*28/25-28;
}
temp1=1023*temp1/50;
OCR1A=temp1;
OCR1B=temp1;
}
/////Speed increase/decrease//////
void add(uchar*p)
{
if(p[1]!=5)
{
p[2]+ +;
if(p[2]==10)
{
p[2]=0;p[1]++;
}
}
}
void sub(uchar *p)
{
uchar temp;
temp=p[1]*20+ p[2]*2;
if(temp!=0)
temp=temp-2;
p[1]=temp/20; //Setting value restoration//
p[2]=(temp-p[1]* 20)/2;
}
void display()
{ write_string(0x80,chr);
write_string(0xc0,chr1[key]);
write_order(counter1+0);
write_Data(data1[0]+0x30);
for(j=1 ;j<3;j++)
{write_order(counter1+j);
write_Data(data1[j]+0x30);
}
for(j=0;j<4;j++)
{
write_order(counter2+j);
write_Data(data2[j]+0x30);
}
}
//////Speed adjustment////
void speed_change(void)
{
if(PINA.1==0)
{
delay_ms(100);
if(PINA.1==0)
{add(data1); }
}
if(PINA.2==0)
{delay_ms(100);
if(PINA.2==0)
{sub(data1);}
}
}
/////Keyboard reading////
void testkey(void )
{
uchar flag2=0;
if(PINA!=0x0f)
{ delay_ms(10);
if(PINA!=0x0f)
{flag2=1;}
}
if(flag2)
{
while(PINA.3!=0)
{
while(PINA .0==0)
{ delay_ms(100);
if(PINA.0==0)
{
if(key<2)
{key++;}
else
key=0;}
}
switch (key)
{case 0: //Mode 1PID adjustment
flag=0;
speed_change();
setpwmr(data1);
display();
break;
case 1: //Mode 2 open loop
flag=1;
speed_change();
setpwmr(data1);
init_intr();
display() ;
break;
case 2:
display();
break;
default:break; }
}
flag2=0;
}
}
/* void speed_convert(uint c) //Convert speed measurement into pwm value
{
}*/
void new_pid(uint cl,uchar k) //Integral separation method
{ int temp3,z,p; //p is the integral coefficient. The larger the error, the smaller P
if(flag)
{ E1r=speedr-cl;
if(speedr>0&abs( E1r)<11)
{
switch(abs(E1r)/2)
{
case 5: p=1;
break;
case 4: p=3;
break;
case 3: p=5;
break;
case 2: p=8;
break;
case 1: p=10;
break;
default :
break;
}
z=23*(E1r-E2r)+p*(E1r-2*E2r+E3r)-10*E3r;
z=(int)z*102 /k;
temp3=OCR1A;
temp3=temp3+z;
OCR1A=temp3;
OCR1B=temp3;
E3r=E2r;
E2r=E1r;
}
}
}
/*void pid_motr(uint cr,uchar k)
{
int temp,u;
if(flag)
{
if(speedr>0)
{
E1r=speedr-cr;
if(E1r==speedr) //E has a large error When the maximum rated current accelerates
{ OCR1A=maxc;
OCR1B=maxc; }
else if(abs(E1r)>0&&abs(E1r)<7)
{
u=(int)(24*E1r-35*E2r+14*E3r)* 102/k;
temp=OCR1A;
temp=temp+u;
OCR1A=temp;
OCR1B=temp;
E3r=E2r;
E2r=E1r;
}
}
}
} */
////////Speed data decomposition/// ////
void speed_count(uint r)
{
data2[3]=r%10;
r=r/10;
data2[2]=r%10;
r=r/10;
data2[1]=r%10;
r=r/10;
data2[0]=r%10;
}
/////Use external interrupt to measure speed//////////
interrupt[EXT_INT0]void int_0(void)
{ c_r++;
}
/////T2 timing 80ms to sample speed////////
interrupt[TIM2_OVF]void time_2(void)
{
MCUCR=0x00;
GICR=0x00;
new_pid(c_r,500);
//pid_motr(c_r,100);
speed_count(c_r);
MCUCR=0x0f; ///External INT1/0 interrupt rising valid
GICR=0xc0;
c_r=0;
}
void initall_IO(void)
{
DDRB=0xf0;
PORTB=0x00;
DDRA=0xF0;
PORTA=0x0F;
DDRC=0XFF;
PORTC=0X00;
DDRD=0xF0;
PORTD=0x0F;
}
void main(void)
{
initall_IO();
init_lcd();
while(1)
{
DDRA=0xF0;
PORTA=0x0F;
testkey();
init_intr();
display();
}
}
The PID algorithm formula is a bit wrong: it should be changed to
z=100*(E1r-E2r)+p*E1r+125*(E1r-2*E2r+E3r);
After the teacher's guidance: the M method for speed sampling is not suitable for low speed, so for the large speed regulation range, the M/T method is used, which is suitable for high and low speed measurement! The speed measurement must also be digitally filtered! The first-order inertia/median method, average method, etc. can be used!
Previous article:Real-time clock chip DS12887 interface driver
Next article:M16-based TC1 can generate 100HZ-1MHZ frequency square wave generator
Recommended ReadingLatest update time:2024-11-16 12:48
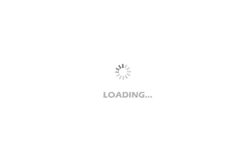
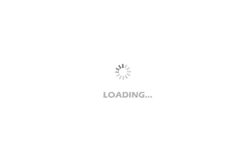
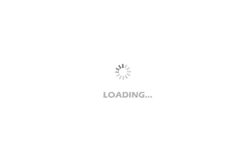
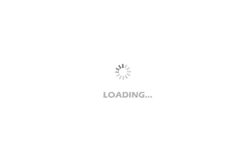
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- The difference and relationship between embedded Linux and embedded development of 51/430/STM32
- 【DM642】Porting of H.264 source code on DM642
- [2022 Digi-Key Innovation Design Competition] Material Unboxing
- Prize-giving live broadcast: Book a session on "Meeting the test challenges in 5G signal generation" and win Keysight gifts
- Simple stopwatch based on MSP430f149 microcontroller
- A power backup solution for NVR/DVR systems
- Is there any solution to adjust the voltage accurately to 0.1V and start continuously and stably? ??? ? Help! ! ! !
- Learn about the internal structure of ST waterproof pressure sensor in this 32-second video
- 【Qinheng CH582】Evaluation Summary
- Introduction to oscilloscope related terms (Part 2)