#define uchar unsigned char
#define uint unsigned int
sbit DQ = P3^3 ; //Define DS18B20 port DQ
sbit BEEP=P3^6 ; //Buzzer drive line
bit presence ; //Check whether 18b20 is properly plugged in
sbit LCD_RS = P2^0 ;
sbit LCD_RW = P2^1 ;
sbit LCD_EN = P2^2 ;
uchar code cdis1[ ] = {" Niuniu Intelligent Technology "} ;
uchar code cdis2[ ] = {" WENDU: . C "} ;
uchar code cdis3[ ] = {" DS18B20 ERR0R "} ;
uchar code cdis4[ ] = {" PLEASE CHECK "} ;
unsigned char data temp_data[2] = {0x00,0x00};
unsigned char data display[5] = {0x00,0x00,0x00,0x00,0x00};
unsigned char code ditab[16] = {0x00,0x01,0x01,0x02 ,0x03,0x03,0x04,0x04,
0x05,0x06,0x06,0x07,0x08,0x08,0x09,0x09} ;
void beep() ;
unsigned char code mytab[8] = {0x0C,0x12,0x12,0x0C,0x00, 0x00,0x00,0x00};
#define delayNOP() ; {_nop_() ;_nop_() ;_nop_() ;_nop_() ;} ;
/****************************************************** ******************/
void delay1(int ms)
{
unsigned char y;
while(ms--)
{
for(y = 0; y<250; y++)
{
_nop_() ;
_nop_() ;
_nop_() ;
_nop_() ;
}
}
}
/******************************************************************/
/*Check LCD busy status */
/*When lcd_busy is 1, it is busy and waiting. When lcd-busy is 0, it is idle and can write instructions and data. */
/**********************************************************************/
bit lcd_busy()
{
bit result ;
LCD_RS = 0 ;
LCD_RW = 1 ;
LCD_EN = 1 ;
delayNOP() ;
result = (bit)(P0&0x80) ;
LCD_EN = 0 ;
return(result) ;
}
/*Write instruction data to LCD */
/*RS=L, RW=L, E=high pulse, D0-D7=instruction code. */
/********************************************************************/
void lcd_wcmd(uchar cmd)
{
while(lcd_busy()) ;
LCD_RS = 0 ;
LCD_RW = 0 ;
LCD_EN = 0 ;
_nop_() ;
_nop_() ;
P0 = cmd ;
delayNOP() ;
LCD_EN = 1 ;
delayNOP() ;
LCD_EN = 0 ;
}
/*******************************************************************/
/*Write display data to LCD */
/*RS=H, RW=L, E=high pulse, D0-D7=data. */
/***********************************************************************/
void lcd_wdat(uchar dat)
{
while(lcd_busy()) ;
LCD_RS = 1 ;
LCD_RW = 0 ;
LCD_EN = 0 ;
P0 = dat ;
delayNOP() ;
LCD_EN = 1 ;
delayNOP() ;
LCD_EN = 0 ;
}
/* LCD initialization settings */
/********************************************************************/
void lcd_init()
{
delay1(15);
lcd_wcmd(0x01); //Clear LCD display contents
lcd_wcmd(0x38); //16*2 display, 5*7 dot matrix, 8-bit data
delay1(5);
lcd_wcmd(0x38);
delay1(5);
lcd_wcmd(0x38);
delay1(5);
lcd_wcmd(0x0c); //Display on and off cursor
delay1(5);
lcd_wcmd(0x06); //Move cursor
delay1(5);
lcd_wcmd(0x01); //Clear LCD display
delay1(5);
}
/* Set display position */
/***********************************************************************/
void lcd_pos(uchar pos)
{
lcd_wcmd(pos | 0x80); //data pointer = 80 + address variable
}
/*Write custom characters to CGRAM */
/*******************************************************************/
void writetab()
{
unsigned char i ;
lcd_wcmd(0x40) ; //Write CGRAM
for (i = 0 ; i< 8 ; i++)
lcd_wdat(mytab[ i ]) ;
}
/*us level delay function */
/***********************************************************************/
void Delay(unsigned int num)
{
while( --num );
}
/*Initialize ds1820 */
/*******************************************************************/
Init_DS18B20(void)
{
DQ = 1 ; //DQ reset
Delay(8); //delay a little
DQ = 0; //MCU pulls DQ down
Delay(90); //Precise delay greater than 480us
DQ = 1; //Pull the bus high
Delay(8);
presence = DQ ; //If = 0, initialization is successful, = 1, initialization fails
Delay(100) ;
DQ = 1 ;
return(presence) ; //Return signal, 0 = presence, 1 = no presence
}
/* Read one byte */
/*******************************************************************/
ReadOneChar(void)
{
unsigned char i = 0 ;
unsigned char dat = 0 ;
for (i = 8 ; i > 0 ; i--)
{
DQ = 0 ; // give pulse signal
dat >>= 1 ;
DQ = 1 ; // give pulse signal
if(DQ)
dat |= 0x80;
Delay(4);
}
return (dat) ;
}
/* Write one byte */
/********************************************************************/
WriteOneChar(unsigned char dat)
{
unsigned char i = 0 ;
for (i = 8 ; i > 0 ; i--)
{
DQ = 0 ;
DQ = dat&0x01 ;
Delay(5) ;
DQ = 1 ;
dat>>=1 ;
}
}
/* Read temperature */
/*******************************************************************/
Read_Temperature(void)
{
Init_DS18B20() ;
WriteOneChar(0xCC) ; // Skip reading serial number and column number
WriteOneChar(0x44) ; // Start temperature conversion
Init_DS18B20() ;
WriteOneChar(0xCC) ; //Skip the operation of reading the serial number and column number
WriteOneChar(0xBE) ; //Read the temperature register
temp_data[0] = ReadOneChar() ; //low 8 bits of temperature
temp_data[1] = ReadOneChar() ; //high 8 bits of temperature
}
/* Data conversion and temperature display */
/***************************************************************************/
Disp_Temperature()
{
display[4]=temp_data[0]&0x0f ;
display[0]=ditab[display[4]]+0x30 ;//Look up the table to get the decimal value
display[4]=((temp_data[0]&0xf0)>>4)|((temp_data[1]&0x0f)<<4) ;
display[3]=display[4]/100+0x30 ;
display[1]=display[4]%100 ;
display[2]=display[1]/10+0x30 ;
display[1]=display[1]%10+0x30 ;
if(display[3]==0x30) //The high bit is 0, not displayed
{
display[3]=0x20 ;
if(display[2]==0x30) //The second high bit is 0, not displayed
display[2]=0x20 ;
}
lcd_pos(0x48);
lcd_wdat(display[3]); //Hundreds display
lcd_pos(0x49);
lcd_wdat(display[2]); //Tens display
lcd_pos(0x4a);
lcd_wdat(display[1]); //Ones display
lcd_pos(0x4c);
lcd_wdat(display[0]); //Decimal places display
}
/***********************************************************************/
/* The buzzer sounds once */
/*******************************************************************/
void beep()
{
unsigned char y ;
for (y=0 ;y<100 ;y++)
{
Delay(60) ;
BEEP=!BEEP ; //Invert BEEP
}
BEEP=1 ; //Turn off the buzzer
Delay(40000) ;
}
/* DS18B20 OK displays the menu */
/*******************************************************************/
void Ok_Menu ()
{
uchar m ;
lcd_init() ; //Initialize LCD
lcd_pos(0) ; //Set the display position to the first character of the first line
m = 0 ;
while(cdis1[m] != '\0')
{ //Display character
lcd_wdat(cdis1[m]) ;
m++ ;
}
lcd_pos(0x40) ; //Set the display position to the first character of the second line
m = 0 ;
while(cdis2[m] != '\0')
{
lcd_wdat(cdis2[m]) ; //Display character
m++ ;
}
writetab() ; //Write custom characters to CGRAM
delay1(5) ;
lcd_pos(0x4d) ;
lcd_wdat(0x00) ; //Display custom characters
}
/* DS18B20 ERROR display menu */
/****************************************************************/
void Error_Menu ()
{
uchar m ;
lcd_init() ; //Initialize LCD
lcd_pos(0) ; //Set the display position to the first character of the first line
m = 0 ;
while(cdis3[m] != '\0')
{ //Display character
lcd_wdat(cdis3[m]) ;
m++ ;
}
lcd_pos(0x40) ; //Set the display position to the first character of the second line
m = 0 ;
while(cdis4[m] != '\0')
{
lcd_wdat(cdis4[m]) ; //Display character
m++ ;
}
}
/* Main function */
/****************************************/
void main()
{
Ok_Menu () ;
do
{
Read_Temperature() ; //Read temperature
Disp_Temperature() ; //Display temperature
}
while(!presence) ;
Error_Menu () ;
do
{
Init_DS18B20() ;
beep() ;
}
while(presence) ;
}
Previous article:C language programming of single chip microcomputer controlling multiple stepper motors
Next article:1602 LCD display infrared remote control decoding C language programming
Recommended ReadingLatest update time:2024-11-17 00:44
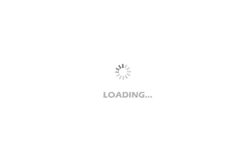
- Popular Resources
- Popular amplifiers
-
Intelligent computing systems (Chen Yunji, Li Ling, Li Wei, Guo Qi, Du Zidong)
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
Single-chip microcomputer C language programming and simulation
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- 【GD32E503 Review】+Hardware System and Environment Establishment
- After my air conditioner became a spirit, my chanting machine also became a spirit
- IO port power saving in various situations
- [2022 Digi-Key Innovation Design Competition] Material Unboxing - STM32H745-DISCO
- How MSP430FRx MCUs achieve higher performance
- SparkRoad Review - Development Environment Installation and Unboxing Review
- Common RF transceiver structure
- Let me tell you about my experience using Gaoyun FPGA these days
- How can a 37-year-old programmer still "dominate the workplace"?
- 【AT-START-F403A Review】+OLED screen driver and application