//Resolution 12 bits
#include
#include
#define uchar unsigned char
#define uint unsigned int
uchar digit[10]="0123456789";
//Delay 1 millisecond program
void delayms();
//Delay s milliseconds program
void delaynms(uint);
//Initialize LCD
void InitLCD();
//Write LCD instruction
void WriteInstruction(uchar);
//Write LCD data
void WriteData(uchar);
//Write address
void WriteAdd(uchar);
//Read LCD status
uchar BusyTest();
//LCD busy query
sbit LCDbusy=P1^7;
//LCD operation bit setting
sbit RS=P2^2;
sbit RW=P2^1;
sbit E=P2^0;
sbit BF=P1^7;
//----- DS1820 declare variable function
sbit DQ=P3^0;
sbit led=P3^1; //Signal indication
uchar TL,TH; //Read DS1820 temperature data high and low bits
//Initialize DS1820
bit InitDS1820();
//Read one byte from DS1820
uchar ReadOneChar();
//Write one byte to DS1820
WriteOneChar(uchar);
//Read temperature
void readtemp();
////Display temperature
void dispay();
uchar flag=0;
//main program
void main()
{
uchar i;
uchar code str[]="thermometer is:";
//Initialize LCD
InitLCD();
//Write address
WriteAdd(0x01);
//Write data
i=0;
while(str[i]!='\0')
{
WriteData(str[i]);
i++;
}
//Write address
while(1)
{
readtemp();
//If it is a negative temperature, display a minus signif
(flag)
{
WriteAdd(0x44);
WriteData('-');
}
else
{
WriteAdd(0x44);
WriteData(' ');
}
WriteAdd(0x45);
dispay();
delaynms(1000);
}
}
//Initialize DS1820
bit InitDS1820()
{
uchar t;
bit flag;
DQ = 1;
_nop_();
DQ = 0;
//Maintain 600us
for(t=0;t<200;t++);
//Release bus
DQ = 1;
//Wait 15~30us, DS1820 outputs response signal,
//Here delay 45us
for(t=0;t<15;t++);
//Sampling response signal
flag=DQ;
//The response signal is maintained for 60~240us
// Here the delay is 300us,
for(t=0;t<100;t++);
return (flag); //Return the detection success flag
}
//Read a character from DS1820
uchar ReadOneChar()
{
uchar i,t;
uchar dat;
for(i=0;i<8;i++)
{
dat>>=1;
DQ=1; //c51 driver
_nop_();
DQ=0; //c51 driver
_nop_(); //Delay 1 us to release the bus
DQ=1; //c51 releases bus
//DS1820 prepares data
_nop_();
_nop_();
_nop_();
_nop_();
_nop_();
//samplingif
(DQ==1) //c51 samplingdat
|=0x80;
else
dat|=0x00;
//It takes 60us to complete a read cycle, and the delay here is 60us.
//One cycle is about 3us
for(t=0;t<20;t++);
}
return dat;
}
//Write one byte to DS1820
WriteOneChar(uchar dat)
{
uchar i,t;
for(i=0;i<8;i++)
{
DQ=1; //c51 driver_nop_
();
DQ=0; //c51 releases the bus
//Starting from the low bit, the i-th bit of data is transmitted to the bus
DQ=dat&0x01;
//DS1820 samples 15~60us after DQ=0.
//So the delay is 60us,
for(t=0;t<20;t++);
//Prepare the next data
dat>>=1;
}
}
//Read temperature
void readtemp()
{
InitDS1820();
//Ignore DS1820 address
WriteOneChar(0xcc);
//Start temperature conversion, time 750ms
WriteOneChar(0x44);
//Delay 1000ms,
delaynms(1000);
InitDS1820();
//Ignore DS1820 address
WriteOneChar(0xcc);
//Read temperature
WriteOneChar(0xbe);
TL=ReadOneChar();
TH=ReadOneChar();
}
//Display temperature
void dispay()
{
uchar b,s,g; //Represents hundreds, tens and ones
uchar d1,d2; //1st and 2nd decimal places
unsigned int ti,td;
if(TH<0xfc)
{
//positive temperature
flag=0;
ti=TH*16+TL/16; //integer part
td=TL%16; //decimal part
//
}
else
{
//Negative temperature,
flag=1;
ti=TH*256+TL;
ti=~ti+1;
ti=ti/16;
td=(~TL+1)%16;
}
g=ti%10 ;
s=(ti%100)/10;
b=ti/100;
d1=td*10/16; // 1st decimal
d2=td*100/16%10; // 2nd decimal
//
WriteData(digit[b]);
WriteData(digit[s]);
WriteData(digit[g]);
WriteData('.');
WriteData(digit[d1]);
WriteData(digit[d2]);
}
//Initialize LCD
void InitLCD()
{
delaynms(15);
WriteInstruction(0x38); //Display mode setting
delaynms(5);
WriteInstruction(0x38);
delaynms(5);
WriteInstruction(0x38);
delaynms(5);
WriteInstruction(0x0d); //Display mode setting: on display, no cursor, blinking cursor
delaynms(5);
WriteInstruction(0x06); //Display mode setting: cursor moves right, text does not move.
delaynms(5);
}
//Write LCD instruction
void WriteInstruction(uchar instruction)
{
//LCD is busy, waiting.
while(BusyTest()==1);
//Write instruction
RS=0;
RW=0;
E=0;
_nop_();
_nop_();
_nop_();
//Instruction
P1=instruction;
_nop_();
_nop_();
_nop_();
E=1;
_nop_();
_nop_();
_nop_();
E=0;
}
//Write LCD data
void WriteData(uchar d)
{
//LCD is busy, waiting.
while(BusyTest()==1);
//Write data
RS=1;
RW=0;
E=0;
_nop_();
_nop_();
_nop_();
//Instruction
P1=d;
_nop_();
_nop_();
_nop_();
E=1;
_nop_();
_nop_();
_nop_();
E=0;
}
//Write address, belongs to write instruction
void WriteAdd(uchar ad)
{
uchar addr=ad+0x80;
WriteInstruction(addr);
}
//Read LCD status
uchar BusyTest()
{
bit result;
//Read LCD status
RS=0;
RW=1;
E=1;
_nop_();
_nop_();
_nop_();
//Command
result=BF;
_nop_();
_nop_();
_nop_();
E=0;
return result;
}
void delaynms(uint s)
{
uint tem;
for(tem=0;tem
{
delayms();
}
}
//Delay 1ms
void delayms()
{
uchar i;
for(i=0;i<250;i++);
for(i=0;i<80;i++);
}
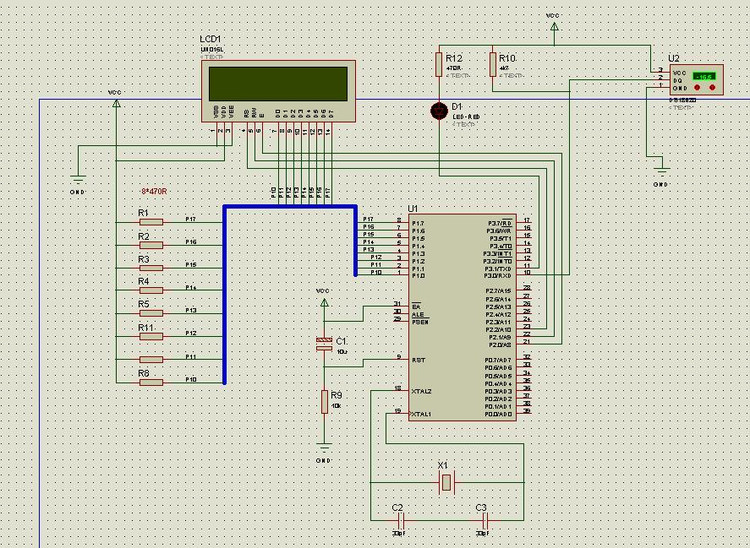
Previous article:c51: Read the DS1820 serial number and display it
Next article:C51: LCD1602 displays the measured value
Recommended ReadingLatest update time:2024-11-16 15:48
- Popular Resources
- Popular amplifiers
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
How to read electrical control circuit diagrams (Classic best-selling books on electronics and electrical engineering) (Zheng Fengyi)
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Leverage the advantages of real-time operating systems with MSP432 MCUs
- Start with TF card made by card reader
- 【RT-Thread software package application works】Smart home
- EEWORLD University----Live Replay: How to Build a Car Charger
- IoT standards: Game over
- 无需重新设计电路板?三大提示助你显著改善降压转换器中的EMI!
- TouchGFX design——by supermiao123
- EEWORLD University Hall----Overview of THS6222 Broadband PLC Line Driver
- Optimization and Implementation Method of Task Scheduling in μC/OS
- Does anyone know what model this monster is?